COBOL Arrays
COBOL ARRAYS
- Instead of defining the same type of data multiple times that may fall under the same category or group, COBOL provides us with a powerful function called as array.
- To optimize the usage and property of elements, these elements of the same type and behaviour can be grouped in the internal table, and termed as array.
- The records/data, which are to be stored in an array must contain similar properties, i.e., PIC CLAUSE.
- Arrays are divided in the form of rows and columns.
- An array can be of different types; 1 - dimensional, 2-dimensional.
INTERNAL TABLE COMMAND OR ARRAYS
FOR EXAMPLE,
Suppose we have to define the days of the week, either we can represent statements like this.
01 WEEK 05 DAY01 PIC X(9) VALUE 'MONDAY' . 05 DAY02 PIC X(9) VALUE 'TUESDAY' . 05 DAY03 PIC X(9) VALUE 'WEDNESDAY' . 05 DAY04 PIC X(9) VALUE 'THURSDAY' . 05 DAY05 PIC X(9) VALUE 'FRIDAY' . 05 DAY06 PIC X(9) VALUE 'SATURDAY' . 05 DAY07 PIC X(9) VALUE 'SUNDAY' .
Instead of doing this, we can simply define internal table week and later input these elements in a single group making it easy for us to manipulate data.
01 WEEK-TABLE. 05 DAY-NAME PIC X(9) OCCURS 7 TIMES . OR 01 WEEK-TABLE. 05 DAY-NAME OCCURS 7 TIMES PIC X(9) .
OCCUR CLAUSE:
- The occur Claus is used to define the number of occurrences of an element in a table or array.
- Occur Claus must be used for group or elementary data items only.
- Depending on the type of array, i.e., one dimensional or two-dimensional, we can have multiple occur Claus.
- To access the elements of the table, we can use index or subscript.
- One dimensional array contains one OCCUR clause, and the two-dimensional array contains two OCCUR Claus.
REFERRING ELEMENTS IN AN ARRAY:
There are two ways to refer to the elements in an array:
1) INDEX:-
It refers to the displacement position of number from the starting of an array, also known as offset. We use the " INDEX BY " clause to declare index, and we do not define index in the working storage section.
SYNTAX =>
01 TABLE-WEEK . 05 NAME-DAY PIC X(09) OCCURS 7 TIMES [ ASCENDING / DESCENDING KEY IS key-1 . . . ] [ INDEXED BY index-name ] .
EXAMPLE => using the above syntax
NAME-DAY(1) has a displacement of 0
NAME-DAY(2) has a displacement of 9
NAME-DAY(1) has a displacement of 18 and so on . . .
If we increase the index by 1, the length gets increased by the length defined in the PIC clause.
How to assign a value to the index?
The SET CLAUSE is used to initiate INDEX or to set the value of the INDEX.
EXAMPLE =>
a) SET index-1 to index-2
b) SET index-1 to 1
c) SET index-1 UP BY 1
d) SET index-1 DOWN BY 1
NOTE:- SET is also used to set the value of switch to ON / OFF. It can also be used to assign TRUE / FALSE to a conditional statement.
2) SUBSCRIPT:-
- An integral data item that describes the number of occurrence of elements in an array.
- Subscript refers to the memory location of a particular data item and where it resides. It means the allocated memory is not the actual memory; instead points towards the position of the data item.
- The subscripts are defined in the working storage section with
' PIC a9(04) COMP ' .
- To initialize the subscript, we have to use the MOVE statement.
- Subscript starts with number 1 and later 1 is increased every time with the help of ADD function.
EXAMPLE =>
-- To refer an element one in the last example, we have to give NAME-DAY(1)
-- In order to select element two in the last example, we have to do the following
NAME - DAY ( 2 ) and so on . . .
SAMPLE PROGRAM -
This is a demo program to learn and understand the concept of one-dimensional array and how to assign values to array.
CREATING 1 DIMENSIONAL ARRAY IDENTIFICATION DIVISION. PROGRAM-ID. ONE-D-ARR. DATA DIVISION. WORKING-STORAGE SECTION. 01 WS-STR-TABLE. 05 WS-PRD OCCURS 3 TIMES PIC X(10). PROCEDURE DIVISION. MOVE "SOAP " TO WS-PRD(1). MOVE "DETERGENT " TO WS-PRD(2). MOVE "SHAMPOO " TO WS-PRD(3). DISPLAY "I AM EXAMPLE OF ONE DIMENSIONAL ARRAY". DISPLAY "PRODUCT 1 : " WS-PRD(1). DISPLAY "PRODUCT 2 : " WS-PRD(2). DISPLAY "PRODUCT 3 : " WS-PRD(3). STOP RUN.
THE OUTPUT WILL BE
I AM EXAMPLE OF ONE DIMENSIONAL ARRAY PRODUCT 1 : SOAP PRODUCT 2 : DETERGENT PRODUCT 3 : SHAMPOO
Here in this program, we have assigned a few variables with different values and later manipulated the output using different properties of an array to make you clear about the different concepts related to ONE DIMENSIONAL ARRAY. With this program and output, you will be able to understand how convenient and easy arrays are.
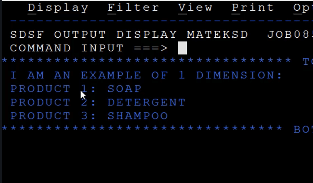
SAMPLE PROGRAM -
This is a demo program to learn and understand the concept of two-dimensional array and how to assign values to array.
CREATING 2 DIMENSIONAL ARRAY IDENTIFICATION DIVISION. PROGRAM-ID. TWO-D-ARR. DATA DIVISION. WORKING-STORAGE SECTION. 01 WS-STORE-PROD-TABLE. 05 WS-STORE-ID OCCURS 3 TIMES. 10 WS-PRODUCT OCCURS 5 TIMES PIC 9(08). 01 WS-TIME PIC 9(08). 01 I PIC 9(01). 01 J PIC 9(01). PROCEDURE DIVISION. ACCEPT WS-TIME FROM TIME. DISPLAY "I AM EXAMPLE OF TWO DIMENSIONAL ARRAY". PERFORM PRODUCT-PARA VARYING I FROM 1 BY 1 UNTIL I > 3 AFTER J FROM 1 BY 1 UNTIL J > 5. STOP RUN. PRODUCT-PARA. MOVE WS-TIME TO WS-PRODUCT(I, J). DISPLAY "PRODUCT" I "," J WS-PRODUCT(I, J). ADD 1 TO WS-TIME.
THE OUTPUT WILL BE
I AM EXAMPLE OF TWO DIMENSIONAL ARRAY PRODUCT1 , 114350661 PRODUCT1 , 214350662 PRODUCT1 , 314350663 PRODUCT1 , 414350664 PRODUCT1 , 514350665 PRODUCT2 , 114350666 PRODUCT2 , 214350667 PRODUCT2 , 314350668 PRODUCT2 , 414350669 PRODUCT2 , 514350670 PRODUCT3 , 114350671 PRODUCT3 , 214350672 PRODUCT3 , 314350673 PRODUCT3 , 414350674 PRODUCT3 , 514350675
Here in this program, we have assigned a few variables with different values and later manipulated the output using different properties of an array to make you clear about the different concepts related to TWO-DIMENSIONAL ARRAY. With this program and output, you will be able to understand how convenient and easy multi-dimensional arrays are.
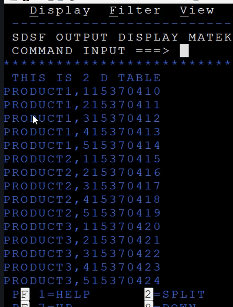
SAMPLE PROGRAM -
This is a demo program to learn and understand the concept of index by property of an array and how to assign values to array.
IDENTIFICATION DIVISION . PROGRAM-ID.INDEXBY. DATA DIVISION . WORKING - STORAGE SECTION . 01 WS - STORE . 05 WS - PRODUCT OCCURS 3 TIMES INDEXED BY IDX . 10 WS - ITEM - CODE PIC 9(05) . 01 WS - ITEM - VAL PIC 9(05) VALUE 12345 . PROCEDURE DIVISION . SET IDX TO 1 . PERFORM PROD-DETAILS UNTIL IDX > 3 . STOP RUN . PROD - DETAILS . MOVE WS - ITEM - VAL TO WS - ITEM - CODE(IDX). DISPLAY " PRODUCT " WS - ITEM - CODE(IDX). ADD 1 TO WS - ITEM - VAL. SET IDX UP BY 1. THE OUTPUT WILL BE PRODUCT 12345 PRODUCT 12346 PRODUCT 12347
Here in this program, we have assigned a few variables with different values and later manipulated the output using different properties of an array to make you clear about the different concepts related to INDEX BY property of an ARRAY.
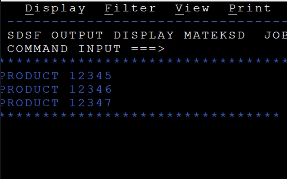
SAMPLE PROGRAM -
This is a demo program to learn and understand the concept of subscript property of an array and how to assign values to array.
IDENTIFICATION DIVISION. PROGRAM-ID. SUBSCR. DATA DIVISION. WORKING-STORAGE SECTION. 01 WS-STORE. 05 WS-PRODUCT OCCURS 3 TIMES INDEXED BY IDX. 10 WS-ITEM-CODE PIC 9(05). 01 WS-ITEM-VAL PIC 9(05) VALUE 12345. 01 WS-SUB PIC 9(01) VALUE 01. PROCEDURE DIVISION. MOVE 1 TO WS-SUB. PERFORM PROD-DETAILS UNTIL WS-SUB > 3. STOP RUN. PROD-DETAILS. MOVE WS-ITEM-VAL TO WS-ITEM-CODE(IDX). DISPLAY "PRODUCT " WS-SUB " " WS-ITEM-CODE(IDX). ADD 1 TO WS-ITEM-VAL. ADD 1 TO WS-SUB. SET IDX UP BY 1.
THE OUTPUT WILL BE
PRODUCT 1 12345 PRODUCT 2 12346 PRODUCT 3 12347
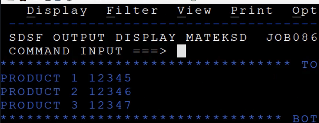
Here in this program, we have assigned a few variables with different values and later manipulated the output using different properties of an array to make you clear about the different concepts related to the SUBSCRIPT property of an ARRAY.
DIFFERENCE BETWEEN INDEX AND SUBSCRIPT
As we are already aware, both index and subscript are powerful tools in COLOB programming and mostly do the same task, but still, there's a huge difference that we will be discussing.
INDEX =>
- Refers to the offset or displacement in the starting of an array.
- We use INDEX BY Claus to initiate the value.
- INDEX is much faster compared to SUBSCRIPT as it refers to the memory location, so it has comparatively better and faster performance.
- INDEX is not defined in the working storage section and is initialized by the SET operation.
SUBSCRIPT =>
- It refers to the array elements' occurrence, and we do not use the INDEX BY clause to initiate.
- SUBSCRIPT is much slower compared to INDEX, as it does not refer to memory location.
- We have to define SUBSCRIPT in the working storage section and is initialized by VALUE or MOVE statements.
SEARCH AND SEARCH ALL
In certain situations, we must search for a particular record or value present in a table. We shall search all the data manually, finding certain data, but that is not that easy. Instead, we can use commands like search or search all to easily filter the complete data and find our desired result.
SEARCH =>
It is a linear or sequential search and is mostly used in tables and array where data is not sorted.
SYNTAX =>
SEARCH TABLE - NAME [ VARYING { identifier1 / index1 } ] [ AT END Statement ] { WHEN ConditionPass { statement / NEXT SENTENCE / CONTINUE } . . . } END - SEARCH .
SEARCH ALL =>
It is a type of binary search and is mostly used when arrays and tables are already sorted.
SYNTAX =>
SEARCH ALL TABLE - NAME [ AT END Statement ] { WHEN ConditionPass { statement / NEXT SENTENCE / CONTINUE } . . . } END - SEARCH .
DIFFERENCE BETWEEN SEARCH AND SEARCH ALL
SEARCH =>
- SEARCH is used to find an element or record present in a linear manner, also known as sequential search.
- We can code the SEARCH command for the tables and arrays that have record either sorted or unsorted, but we mostly use search for unsorted tables.
- In this type of search, the index must be initialized.
- There is no concept for ascending and descending key in SEARCH.
SEARCH ALL =>
- SEARCH ALL is used to find elements or record present in BINARY format, also known as BINARY search.
- We can code the SEARCH ALL command for the tables and arrays that have sorted records.
- In this type of search, the index does not require to be initialized.
- Ascending and descending key must be defined in an array while using the SEARCH ALL command.
SAMPLE PROGRAM -
This is a demo program to learn and understand the concept of search all property of an array and how to assign values to array.
IDENTIFICATION DIVISION. PROGRAM-ID.SEARCH. DATA DIVISION. WORKING-STORAGE SECTION. 01 WS-STORE. 05 WS-PRODUCT OCCURS 3 TIMES ASCENDING KEY IS WS-ITEM-CODE INDEXED BY IDX. 10 WS-ITEM-CODE PIC 9(05). 01 WS-ITEM-VAL PIC 9(05) VALUE 12345. PROCEDURE DIVISION. SET IDX TO 1. PERFORM PROD-DETAILS UNTIL IDX > 3. PERFORM SEARCH-ALL-PARA. STOP RUN. PROD-DETAILS. MOVE WS-ITEM-VAL TO WS-ITEM-CODE(IDX). DISPLAY " PRODUCT " WS-ITEM-CODE(IDX). ADD 1 TO WS-ITEM-VAL. SET IDX UP BY 1. SEARCH-ALL-PARA. SEARCH ALL WS-PRODUCT AT END DISPLAY "RECORD NOT FOUND" WHEN WS-ITEM-CODE(IDX) = 12346 DISPLAY "RECORD FOUND" DISPLAY WS-ITEM-CODE(IDX) END-SEARCH.
THE OUTPUT WILL BE
PRODUCT 12345 PRODUCT 12346 PRODUCT 12347 RECORD FOUND 12346
Here in this program, we have assigned a few variables with different values and later manipulated the output using different properties of an array to make you clear about the different concepts related to the SEARCH ALL property of an ARRAY.
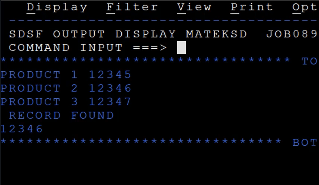