CodeIgniter Security Helper
A Security helper file contains some predefined functions that are used to protect application from unauthorized access.
Loading the Helper
The following syntax is used to load the security helper in the CodeIgniter application.
Syntax
$this-> load-> helper (‘security’);
The Function of Security helper
- xss_clean() : xss_clean means Cross-site scripting, which is used to provide security in web application. It is a technique to stop and filter the JavaScript or malicious code and convert it into safe character entities.
Syntax
xss_clean ($str [, $is_image = FALSE]);
It has two parameters:
$str : It takes an input string for passing in xss_clean() function.
$is_image : It shows whether you are dealing with image or not. If no, pass FALSE otherwise TRUE.
Example:
echo "<b> xss_clean() function : </b>". xss_clean($disp, $is_image =FALSE).
2.sanitize_filename(): This function is used to provide security to levels of directory such as application/folder/files by converting them into a plain text.
Syntax
sanitize_filename ($filename);
$filename : It takes a filename as a string.
Example
echo sanitize_filename ('C:/wamp64/www/CodeIgniter-3.1.11/); /* it shows directory level in a simple text such as C:wamp64wwwCodeIgniter-3.1.11 */
3.do_hash(): It is a hashing technique that converts your text to a secure encrypted format using the md5 and sha1 algorithm.
Syntax
do_hash ( $str [, $type = ‘sha1’ ]);
It has two parameters:
- $str : It takes an input string.
- $type : It defines which type of algorithm you want to implement in a hash function.
Example
$info="programmer"; echo do_hash($info, 'md5'); // It encrypt word ‘programmer’ using md5 algorithm
4.strip_image_tags(): It is a security function that removes the image tags from a string and converts the image URLs into a plain text.
Syntax
strip_image_tags ($str);
$str: It defines an image file as an input string.
Example:
$data = '<img src = "CodeIgniter-3.1.11/images/cloud.jpg"></img>'; echo "<b> strip_image_tags() function : </b>".strip_image_tags($data); /* it shows image path as a simple text */
5.encode_php_tags(): This function is used to remove the php tag from the input string and convert it into a secure entity.
Syntax
encode_php_tags ($str);
$str: It holds an input strings that pass on the encode_php_tags () function.
Example:
echo encode_php_tags( “Hello Preogrammer” );
Create a simple program of Security helper
Create a controller file Online.php and save it in application/controller. After that, write the following program in your controller file.
<?php class Online extends CI_Controller { public function secure() { $this->load->helper('security'); echo “<title> Tutorial and Example </title>”; // xss_clean() function $var = "<script> alert ('hello hacker') </script>"; $disp = "<script> It is used to filter out the potential xss attacks. </script>"; echo "<b> xss_clean() function : </b>". xss_clean($disp, $is_image =FALSE). "<br>"; echo xss_clean($var); echo "<br>"; // sanitize_filename() function echo " <b> sanitize_filename() function : </b>".sanitize_filename ('C:/wamp64/www/CodeIgniter-3.1.11/Files/hello'); echo "<br>"; // do_hash() function with SHA1 Algorithm $data ='welcome'; echo "<b>welcome message convert into SHA1 Security </b> :".do_hash($data); // SHA1 echo "<br>"; // do_hash() function with MD5 algorithm $show ="Codeigniter"; echo "<b> CodeIgniter message convert into md5 security </b> :".do_hash($show, 'md5'); // strip_image_tags() function $data = '<img src = "CodeIgniter-3.1.11/images/valley.jpg"></img>'; echo "<b> strip_image_tags() function : </b>".strip_image_tags($data); echo "<br>"; // encode_php_tags() function $va = "Welcome to the CodeIgniter tutorials"; echo "<b> encode_php_tags() function : </b>".encode_php_tags($va); echo "<br>"; } } ?>
When you execute the above program in localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/online/, itcalls the secure functionandshows the output, as shown below.
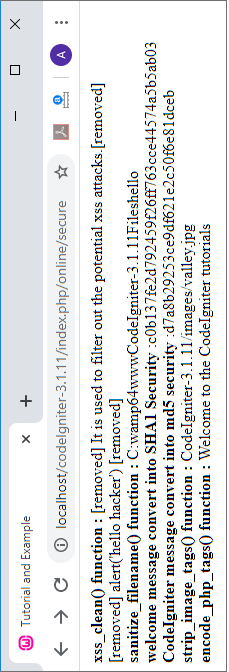