CodeIgniter URL Helper
The helper file contains some predefined functions that are used to work with URL, such as generate the current file path, transfer control, redirect, create a link, etc.
Load the URL Helper
Before using the URL helper in the CodeIgniter application, you must load it in the controller file such as:
Syntax
$this->load->helper(‘url’);
Functions of URLs Helper:
- site_url(): It is used to return the site URL that you have defined in the config file. You can pass any string or an array that you want to appear on the browser.
Syntax
site_url ( [ $url = ‘ ‘ [, $protocol = NULL ] ]);
It has two parameters:
$url: It defines a URL string or an array that you can pass to the site_url () function.
$protocol: It defines the protocol such as ‘http’ or ‘https’ and if you don’t want to show any protocol before the URL, you can use NULL value.
Example
echo site_url ( ‘hello/welcome/to/codeigniter’ ,https’ ); // It prints https://localhost/codeIgniter-3.1.11/index.php/hello/boy
- base_url(): A base_url () is used to return the site base URL that you set in the config file. It is similar to site_url () function used to return the site URL. However, site_url () returns the URL with index.php or url_suffix, that you defined in the config file, but base_url () returns only the URL without index.php.
Syntax
base_url( $url = ‘ ‘, $protocol = NULL );
It has two parameters:
$url: It defines a URL string or an array that you can pass to the base_url () function.
$protocol: It defines the protocol such as ‘http’ or ‘https’ and if you don’t want to show any protocol before the url, you can use NULL value.
Example
echo base_url(); // It prints ‘http://localhost/codeIgniter-3.1.11/’
- current_url(): It is used to return the full URL of the current page with the controller’s name and the function.
Syntax
current_url();
Example
echo current_url(); // It prints http://localhost/codeIgniter- 3.1.11/index.php/online/url_helper
- uri_string(): It is used to fetch the URL segments of any page by defining the name of the url_string() function on any page.
Syntax
uri_string();
Example:
echo uri_string(); // It prints online/url_helper
- index_page(): It is used to return the value of the index_php, which you have set in the config file.
Syntax
index_page();
Example
echo index_page(); // It prints index.php
- anchor() : It is used to create a link on the web page.
Syntax
anchor ( $uri = ‘ ‘ , $title = ‘’, $attributes = ‘’ );
It has three parameters:
$uri: It consists of a string or an array segment that can be appended to the URL.
$title: In this field, you can define the title of the link that can jump to the specific URLs.
$attributes: It contains a list of attributes that you want to display such as 'the title of the link when the user places the mouse over the link', etc.
Example
Create a controller file Online.php and save it in application/controller/Online.php. After that, write the following program in your controller file.
Online.php
<?php class Online extends CI_Controller { public function url_helper() { echo "<title> Tutorial and Example </title>"; $this->load->helper('url'); echo "<b>anchor() : </b>". anchor('/online/localpopover', 'Click me', 'title="transfer control"'); } public function localpopover() { echo "<h2 > welcome to the tutorialandexample </h2>"; } } ?>
When you execute the above program in localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/online/url_helper function, it shows the output, as shown below.
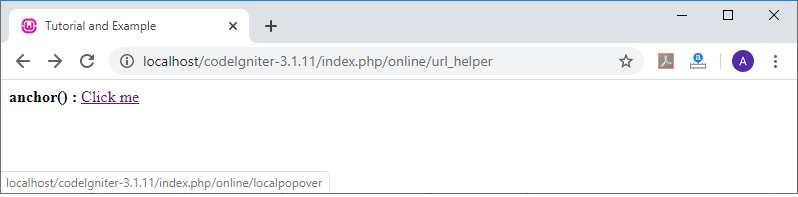
When you click on the ‘Click me’ link, it shows the output as shown below.
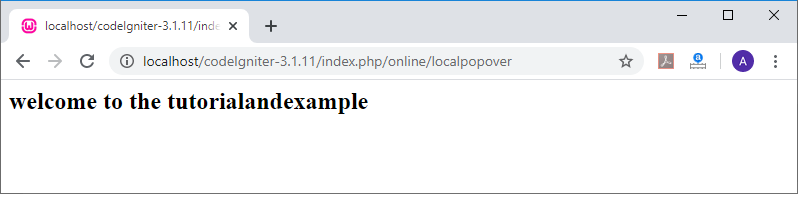
- anchor_popup(): This function is used to create a new popup window when the user clicks on the given link.
Syntax
anchor_popup ( $uri = ‘ ‘, $title = ‘ ‘, $attributes = ‘ ‘);
It has three parameters:
$uri: It consists of a string or an array segment that can be appended to the URL.
$title: In this field, you can define the title of the link that can jump to the specific URLs.
$attributes: It contains a list of attributes that you want to display, such as 'the title of the link, window_name, status, height, width, etc.
Example
Create a controller file Online.php and save it in application/controller/Online.php. After that, write the following program in your controller file.
Online.php
<?php class Online extends CI_Controller { public function url_helper() { echo "<title> Tutorial and Example </title>"; $this->load->helper('url'); echo "<h3> anchor_popup() function "."<br>"; echo "<b>anchor() : </b>". anchor('/online/localpopover', 'Click me', 'title="transfer control"'); } public function localpopover() { echo "<h2 > welcome to the tutorialandexample </h2>"; } } ?>
When you execute the above program in localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/online/url_helper function, it shows the output, as shown below.

After that click on ‘Click Me!’ link, it will show a popup screen.
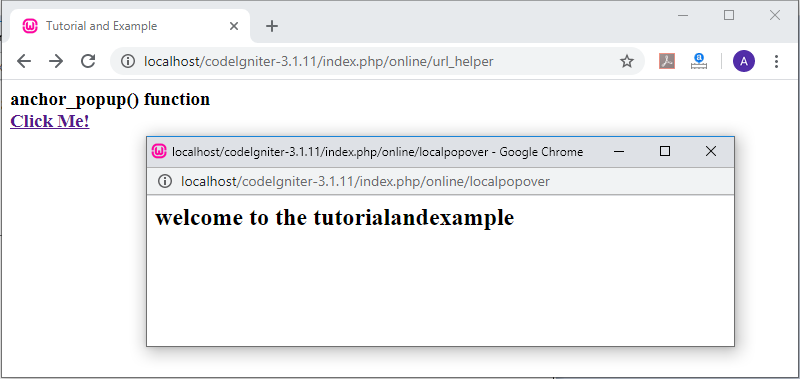
- mailto(): It is used to create a standard email link.
Syntax
mailto ($email, $title = ‘ ‘, $attributes = ‘’ );
It has three parameters:
$email: In this field, you can define an email address.
$title: In this field, you can define the title of the link that can jump to the specific URLs.
$attributes: If you want to show any additional attributes, you can define it in this field.
Example
echo mailto('[email protected]', 'Click Here to read more’);
- safe_mailto(): It is similar to the mailto () function for creating mail links, but it uses JavaScript that helps to secure an email address from spam bots.
Syntax
safe_mailto ($email, $title = ’’, $attributes = ‘’);
It has three parameters:
$email: In this field, you can define an email address.
$title: In this field, you can define the title of the link that can jump to the specific URLs.
$attributes: If you want to show any additional attributes, you can define it in this field.
Example
$attributes = array('title' => 'Email me'); echo safe_mailto('[email protected]', 'Click Me', $attributes);
- auto_link(): As the name defines, this function is used to create an auto-link of the given string automatically.
Syntax
auto_link ($str, $type = ‘both’, $popup = FALSE);
It has three parameters:
$str: It defines an input string for generating a link.
$type: It defines the link type such as ‘email’, ‘URL’ or ‘both’.
$popup: It allows the user to create a popup links.
Example
$string = "[email protected]"; $string = auto_link($string, 'both', TRUE); echo $string;
- url_title(): It is used to create a human friendly URL of the given string.
Syntax
url_title ($str, $seperator = ‘_’, $lowercase = FALSE);
It has three parameters:
$str: It takes an input string for creating a URL.
$separator: It is a word delimiter that is used to separate a string or statement. By default, it uses dashes (-). You can also use underscore ( _ ) for separating a string.
$lowercase: It defines whether you want to convert the output string to lowercase.
Example
$title = "Learn web designing with CodeIgniter”; $url_title = url_title($title); echo url_title;
- prep_url(): It is used to add the prefix http:// to a protocol that is missing in the URL.
Syntax
prep_url ($str = ‘ ‘);
$str: It defines a URL string that pass in prep_url() function.
Example
$url = prep_url('tutorialandexample.com'); echo $url;
- redirect(): It is used to redirect you to any method or page of the application. In other words, it is similar to the base URL function that is used to transfer control from one method to other methods of the application.
Syntax
redirect ($uri = ‘’, $method = ‘auto’, $code = NULL);
It has three parameters:
$uri: It contains a URL string to transfer the control to another method.
$method: It is an optional parameter that allows the user to follow a particular method strictly.
$code: It is also an optional parameter that allows the user to send a specific HTTP response code. It uses 301, 302, and 303 code to redirect with a search engine, location redirects, etc. By default, it uses a 302 responsive code. It is used to redirect the location, not for refreshing.
Example
Create a controller file Online.php and save it in application/controller/Online.php. After that, write the following program in your controller file.
Online.php
<?php class Online extends CI_Controller { public function url_helper() { echo "<title> Tutorial and Example </title>"; $this->load->helper('url'); $logged_in = ''; if ($logged_in == FALSE) { redirect('/Welcome/test'); } } } ?>
When you execute the above program in localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/online/url_helper function. It shows the output, as shown below.

When the above URL will execute, it automatically redirects to Welcome/test page, as shown below.

Example:
Create a controller file Online.php and save it in application/controller/Online.php. After that, write the following program in your controller file.
Online.php
<?php class Online extends CI_Controller { public function url_helper() { echo "<title> Tutorial and Example </title>"; $this->load->helper('url'); echo "<h2> Functions of URL Helpers:</h2>"; echo "<b> site_url() : </b>".site_url('hello/boy', 'https')."<br>"; $data = array('hello', 'welcome', 'to','the', 'codeigniter','3.1.11'); echo "<b> site_url() : </b>".site_url($data); echo "<br>"; echo "<b> base_url() : </b>".base_url(); echo "<br>"; echo "<b> current_url() : </b>".current_url(); echo "<br>"; echo "<b> uri_string() : </b>".uri_string(); echo "<br>"; echo "<b> index_page() : </b>".index_page(); echo "<br>"; echo "<b>mailto() : </b>".mailto('[email protected]', 'Click Here to Read more..'); echo "<br>"; $attr = array('title' => 'Email me'); echo "<b>safe_mailto() : </b>".safe_mailto('[email protected]', 'Click Me', $attr); echo "<br>"; $string = "[email protected]"; $string = auto_link($string, 'both', TRUE); echo "<b>auto_link() : </b>".$string; echo "<br>"; $title = "Learn web designing with CodeIgniter”;"; $url_title = url_title($title); echo "<b> url_title() : </b>".$url_title; echo "<br>"; $title = "Learn web designing with CodeIgniter”;"; $url_title = url_title($title, 'underscore', TRUE); echo " <b> url_title() : </b>".$url_title; echo "<br>"; $url = prep_url('tutorialandexample.com'); echo "<b> prep_url() : </b> ".$url; } } ?>
When you execute the above program in localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/online/url_helper function, it shows the output, as shown below.
