Ionic Actionsheet
Ionic Actionsheet
The Action sheet is a dialog box that displays a group of options. It is shown on the top of the application content and must be dismissed manually before the user can resume interaction with the application. The action sheets slide up from the bottom side of the device screen and display a set of options that can confirm or cancel the action. Sometimes the action sheet can be used as an alternative to lists. However, it should not be used to navigate.
Destructive options become clear in iOS mode. There are several ways to dismiss the action sheet, including touching the bottom or pressing the escape key on the desktop. The Action Sheet always appears on top of any other component of the page and should be ignored to interact with the underlying content. When it is triggered, the rest of the page darkens to give more attention to the options in the action sheet.
The function property of a button can be either destructive or canceled. Buttons that have no role property will have the platform's default look. Buttons with the cancel role will always load as the bottom key, no matter where they are on the list. All other buttons will be shown in the order in which they have been added to the buttons list.
Example: This example explains how you can use the ionic action sheet in the ionic application.
Home.page.html
It is used to design your home page, and it also used to contain HTML elements such as button components, etc.
Home.page.scss
action-sheet-home-page { .ion-md-share { color: rgb(38, 241, 11); } .ion-md-arrow-dropright-circle { color: rgb(240, 34, 137); } .ion-md-heart-outline { color: rgba(31, 133, 60, 0.904); } .action-sheet-cancel ion-icon, .action-sheet-destructive ion-icon { color: #e61010; } }
Home.page.ts
home.page.ts is the main page of the ionic application, and it is used to provide the user interface. Here, you can create the menu, which contains many elements such as destructive, archive, share, delete, create, cancel, etc.
import { Component } from '@angular/core'; import {ActionSheetController } from '@ionic/angular'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { constructor( public actionsheetCtrl: ActionSheetController ) { } async openMenu() { const actionSheet = await this.actionsheetCtrl.create({ header: 'Update Album', buttons: [ { text: 'Destructive', role: 'destructive', handler: () => { console.log('Destructive clicked'); } },{ text: 'Create', handler: () => { console.log('Create clicked'); } },{ text: 'Play', handler: () => { console.log('Play clicked'); } },{ text: 'Favorite', handler: () => { console.log('Favorite clicked'); } },{ text: 'Cancel', role: 'cancel', handler: () => { console.log('Cancel clicked'); } } ] }); await actionSheet.present(); } }
Output: After executing this code, you will get the output, as shown below.
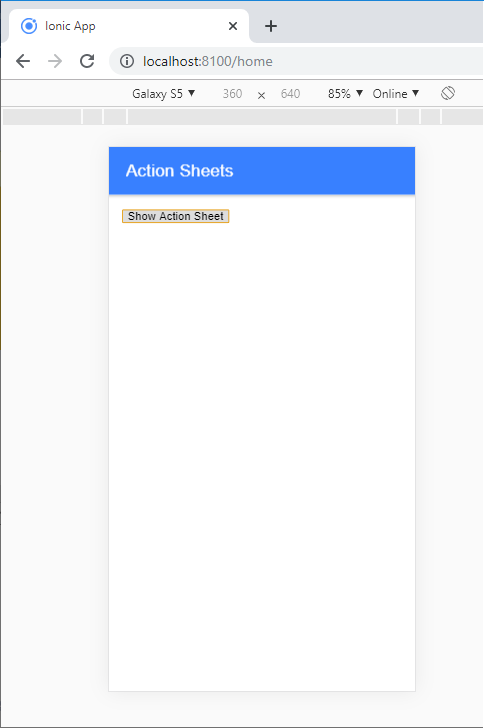
When you click on the show action sheet button, then it shows the below screen. When the button is clicked, the action sheet slide up from the bottom side, and the remainder of the page darkens to give more focus on the options of the action sheet.

Ionic Actionsheet Controller
The Action sheet controllers programmatically control the action sheet element. You can create or dismiss the action sheets from the action sheet controller.
There are some other useful parameters in the $ionicActionSheet.show() method. The following table shows all the parameters of the action sheet.
Properties | Type | Description |
Buttons | Object | It makes the button object with a text field. |
cancelText | String | Text for the cancel button. |
titleText | String | Title for the action sheet. |
destructiveText | String | Text for the destructive button. |
buttonClicked | Function | It calls when one of the buttons tapped. The index is used to keep track of which button is tapped. The action sheet will close when the return is true. |
Cancel | Function | This function called when the cancel button, backdrop, or hardware back button is pressed. |
destructiveButtonClicked | Function | Called when the destructive button pressed. The action sheet will be closed when the return is true. |
cancelOnStageChange | Function | If this is true by default, it will cancel the action sheet when the navigation state is changed. |