Retrieve data from Database
Retrieve data from the Database
Now we will discuss how we can retrieve data from the database that we have inserted to the employees table of the database in our previous topic “inserted data to the database”.
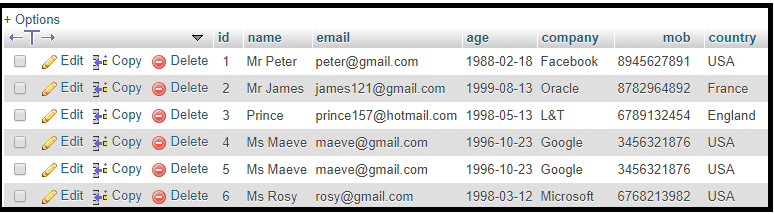
The following are the records of the employees table that we have to retrieve in our CodeIgniter application.
To get the above records, we need to create the following files, as shown below:
Create a controller file Employee_controller.php and save it in application/controller/Employee_controller.php. And write the following program in the controller file.
Employee_controller.php
<?php defined('BASEPATH') OR exit( 'No direct script access allowed'); class Employee_controller extends CI_Controller { function _construct() { parent::_construct(); $this->load->model('Data_Model'); // load a Data_Model } public function index() { $this->load->database(); // load the database $this->load->model('Data_Model'); $query = $this->Data_Model->index(); // fetch records from Data_Model $records['info'] = null; if($query) { $records['info'] = $query; } $this->load->view('fetch', $records); // load a view file } } ?>
Create a model file Data_Model.php and save it in application/model/Data_Model.php. And write the following program in the model file.
Data_Model.php
<?php class Data_Model extends CI_Model { public function __construct() { parent::__construct(); } public function index() { $this->db->select("id, name, email, age, company, mob, country"); /* define the attributes of employees table/ */ $this->db->from('employees'); // retrieve data from an employees table $query = $this->db->get(); return $query->result_array(); } } ?>
Create a view file fetch.php and save it in application/views/fetch.php. And write the following program in the controller file.
fetch.php
<!DOCTYPE> <html> <head> <title> Tutorial and Example </title> </head> <body> <h2> Employee Details </h2> <div id="container"> <p> <b><?php echo anchor('Employee_controller/insert_data', 'Add Employee'); ?> </b></p> <table border = 1> <tr> <th cellpadding = "20"> Id </th> <th cellspacing = "20"> Name </th> <th cellpadding = "20"> Email </th> <th cellpadding = "20"> Employee Age </th> <th cellpadding = "20"> Company </th> <th cellspacing = "20"> Mobile No. </th> <th cellspacing = "20"> Country </th> </tr> <?php foreach ($info as $row): ?> <tr> <td> <?= $row['id'] ?> </td> <td> <?= $row['name'] ?> </td> <td> <?= $row['email'] ?> </td> <td> <?= $row['age'] ?> </td> <td> <?= $row['company'] ?> </td> <td> <?= $row['mob'] ?> </td> <td> <?= $row['country'] ?> </td> </tr> <?php endforeach; ?> </table> </div> </body> </html>
To run the program in the localhost by invoking the URL localhost/CodeIgniter-3.1.11/index.php/Employee_controller function; it shows the output as shown below.
