Salesforce Class and Object in Apex
Class and Object in Apex
Class: A class can be defined as a blueprint or template that describes the behavior/states of the object.
Objects: Objects have states and behaviors. An object is an instance of a class.
Example: A student has states – e.g., name, roll-no.
What goes into class?
- The class represents the blueprint of the object.
- The class comes within curly braces.
- The class has one or more methods.
- The methods should be declared inside a class.
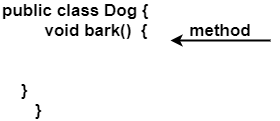

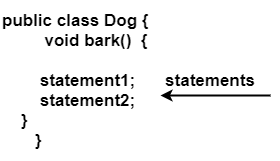
How to create the Object of the class?
Below is the syntax to create the object of the class:
Car a = new Car();
Car a-> Declaration
new Car-> Instantiation
a-> car speed=70
“a” is the reference that holds the memory address of the Car object
Example:
public class Employee { public String name; public String designation; public void show() { System.debug('Name of the employee '+name); System.debug('Designation of the employee '+designation); } } Employee e1=new Employee(); Employee e2=new Employee(); e1.name='Priya'; e1.designation='Software Developer'; e2.name='John'; e2.designation='Manager'; e1.show(); e2.show();
Output:

Example:
public class dog { public String name; public integer age; public void disp() { System.debug('Name of a dog '+name); System.debug('Age of a dog '+age); } } dog a1=new dog(); a1.name='Scooby'; a1.age=8; a1.disp(); dog b1=new dog(); b1.name='Dobby'; b1.age=10; b1.disp();
Output
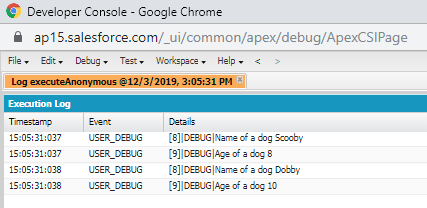
Access Modifiers
Apex grants us to use the private, protected, public, and global access modifiers while defining methods and variables.
Interface methods have no access modifiers. These methods are always global.
By default, a variable or method is visible mainly to the Apex code inside the defining class. We must specify the method or variable as public to make it available to other classes in the same application namespace.
The following access modifiers are available:
- Public: Any Apex can use the method or variable in this namespace or application.
- Private: This is the default modifier that means the method or variable is available only inside the Apex class in which it is defined.
If we do not specify an access modifier, then the variable or method will be private.
- Protected: The method or variable is visible to any inner class in the defined Apex class.
We can use this access modifier, for instance, methods and member variables.
- Global
The method or variable can be used by any Apex code that can access the class.
Example:
public class Cat { public String name; public Integer size; public void display() { System.debug('Name of a cat '+name); System.debug('Size of a cat '+size); } } Cat c=new Cat(); c.name='GUGU'; c.size=12; c.display();
Output:

Example:
public class Cat { private String name; private Integer size; public void setName(String n) { name=n; } public void setSize(integer s) { if(s<=0) { System.debug('We can`t set a wrong value'); size=10; } else { size=s; } } public void display() { System.debug('Name of a cat '+name); System.debug('Size of a cat '+size); } } Cat c=new Cat(); c.setSize(13); c.setName('GUGU'); c.display();
Output:
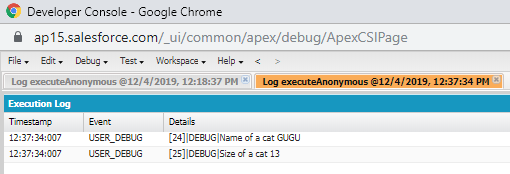
Example:
public class Cat { private String name; private Integer size; public Cat(String n, integer s) { name=n; size=s; } public void display() { System.debug('Name of cat '+name); System.debug('Size of cat '+size); } } Cat c=new Cat('Scooby',14); c.display();
Output:
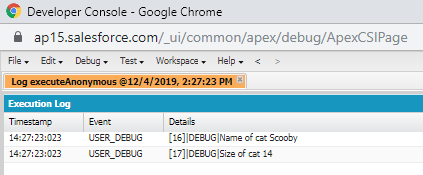