Selenium WebDriver - Firefox or Gecko (Marionette) browser
Selenium web driver-Firefox or Gecko (Marionette) browser
In this tutorial, we are going to learn how to run the Selenium WebDriver test script in the Firefox Browser using the Gecko Driver.
Before going further with this segment, let us first understand the basics of a Gecko Driver.
What is the Gecko Driver?
The gecko driver is a web browser that is used in the developed application by the Mozilla Firefox browser. And it is produced by Mozilla Corporation and Mozilla Foundation.
Gecko Driver lies between our tests script in Selenium and the Firefox browser as a link.
It is a substitute between the W3C WebDriver-compatible clients like Eclipse, NetBeans, etc. to interact with Gecko-based browser (Mozilla Firefox).
Marionette (the next generation of Firefox Driver) is turned on by default from Selenium 3.
Selenium uses the W3C Web driver protocol to send requests to the Gecko Driver, which translates them into a protocol named Marionette.
Even if you are working with older versions of the Firefox browser, Selenium 3 expects you to set the path to the driver executable by the webdriver.gecko.driver.
Note: To open the Firefox driver, we will use the marionette driver instead of the default initialization, which is supported earlier.
We will create a test case in the same test suite (new_test), which we created in the previous tutorials of selenium WebDriver.
Step1:
- Firstly, right-click on the src folder and create a new Class File from New ? Class.
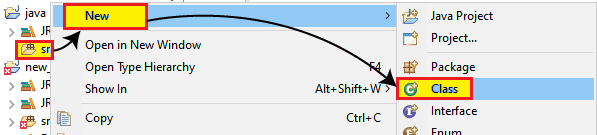
- And give your Class name as Test_gecko and click on the Finish button.
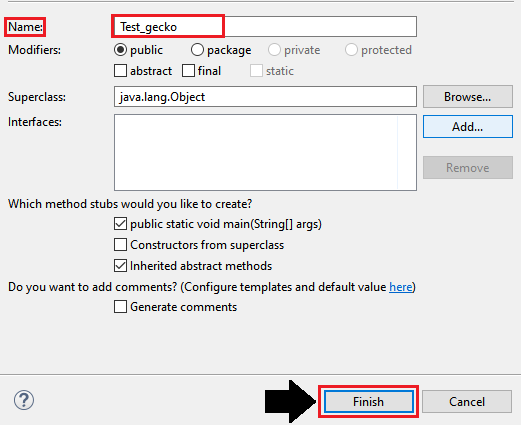
Step2:
- Click on the below link, and it will navigate you to the download page of the Gecko driver in your browser.
https://github.com/mozilla/geckodriver/releases
- Here, you can download the latest version of the Gecko driver and installed it based on the operating system which you are currently working on.
So, we are downloading the 64bit version for the v0.25.0 release of the Gecko Driver for windows.
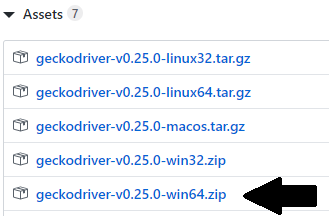
- Download the zip file into your local system and unzip the folder, and it will generate the geckodriver.exe file automatically.

We have three ways to use Gecko Driver in selenium WebDriver:
1. Set the system property for gecko driver:-
Run the server before launching the Firefox browser, with the help of System.property.
Syntax:
System.SetProperty(“key”, ”value”);
Where,
Key = webdriver.gecko.driver
And the path of our geckoDriver.exe file to invoke the GeckoDriver class is as shown below,
Value= C:\\Users\\JTP\\Downloads\\geckodriver-v0.25.0-win64\\geckodriver.exe
Here, is the sample code to set the system property,
// System Property for the gecko Driver System.setProperty("webdriver.gecko.driver","C:\\Users\\JTP\\Downloads\\geckodriver-v0.25.0-win64\\geckodriver.exe"); // create an object for Firefox Driver class. WebDriver driver=new FirefoxDriver();
Or
Using Desired Capabilities:-
Here, the code to set the Gecko driver using Desired Capabilities class:
DesiredCapabilities cap = DesiredCapabilities.firefox(); cap.setCapability("marionette",true);
Sample code for the desired capabilities:
System.setProperty("webdriver.gecko.driver"," C:\\Users\\JTP\\Downloads\\geckodriver-v0.25.0-win64\\geckodriver.exe " ); DesiredCapabilities cap = DesiredCapabilities.firefox(); cap.setCapability("marionette",true); WebDriver driver= new FirefoxDriver(cap);
2. Using marionette property:-
It can also be initialized using the marionette property.
System.setProperty("webdriver.firefox.marionette"," C:\\Users\\JTP\\Downloads\\geckodriver-v0.25.0-win64\\geckodriver.exe ");
- Code for Desired Capabilities is not mandatory for the marionette method.
3. Using Firefox Options:-
Marionette driver has a legacy system for Firefox 47 or later versions. And, the marionette driver can be called using Firefox Options, which is shown below,
FirefoxOptions options = new FirefoxOptions(); options.setLegacy(true);
Now, let us see one sample test case in which we will try to automate the following scenarios in the Firefox browser.
Steps | Action | Method Used | Input | Excepted Result |
1. | Open the Firefox Browser. | System.setProperty() | The Firefox browser must be opened. | |
2. | Navigate to the Given URL of the website. | get() | https://www.facebook.com/ | The home page window of Facebook application must be displayed. |
3. | Identify the username text box and passing the value for it. | The username text box should be identified, and the value should be passed. | ||
4. | Identify the password text box and passing the value for it. | The password text box should be identified, and the value should be passed. | ||
5. | Close the Browser. | close() | The browser should beterminated. |
Here is the sample code for the above example,
package testpackage; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class test_gecko { public static void main(String[] args) throws InterruptedException { // set the system property for gecko driver System.setProperty("webdriver.gecko.driver","C:\\Users\\JTP\\Downloads\\geckodriver-v0.25.0-win64\\geckodriver.exe"); // create driver object for gecko browser WebDriver driver=new FirefoxDriver(); // Launch the Website driver.navigate().to("https://www.facebook.com/"); // pass the value to the email text box driver.findElement(By.id("email")).sendKeys("hello world!"); Thread.sleep(2000); System.out.println("msg printed"); // pass the value to the password text box driver.findElement(By.name("pass")).sendKeys("123456"); Thread.sleep(2000); System.out.println("passed"); //close the browser driver.close(); } }
- Now, right-click on the Eclipse code and select Run as ? Java Application.
The output of the above test script would be displayed in the Firefox browser.

- The output of all print command of the above test script would be displayed in the Eclipse console window.
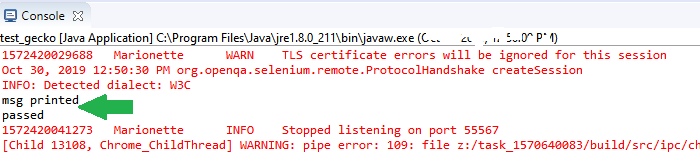