Servlet AsyncEvent and AsyncListener
AsyncEvent
AsyncEvent is an asynchronous event class responsible to generate events. This class is present in javax.servlet package.
This event occurs when various asynchronous tasks operating on ServletRequest get completed, expired or generate errors. ServletRequest is initiated by calling startAsync() method of ServletRequest type.
Method of AsyncEvent
Method |
Description |
AsyncContext getAsyncContext() |
This method is used to initialize AsyncEvent. |
ServletRequest getSuppliedRequest() |
This method returns the ServletRequest which is used to initialize AsyncEvent. If ServletRequest is not used to initialize AsyncEvent then it returns null. |
ServletResponse getSuppliedResponse() |
This method returns the ServletResponse which is used to initialize AsyncEvent. If ServletResponse is not used to initialize AsyncEvent then it returns null. |
Throwable getThrowable() |
This method returns the Throwable which is used to initialize AsyncEvent. If Throwable is not used to initialize AsyncEvent then it returns null |
AsyncListener
AsyncListener is an Asynchronous Listener Interface used to indicate that event is occurred. This interface is also present in javax.servlet package.
It notifies that event occurs for asynchronous tasks operating on ServletRequest when listener has been added gets completed, expired or generates errors.
Methods of AsyncListener
Following are the methods provided by AsyncListener.
Method |
Description |
void onComplete(AsyncEvent event) |
This method indicates that an asynchronous operation has been completed. |
void onError(AsyncEvent event) |
This method indicates that an asynchronous operation generates an error. |
void onStartAsync(AsyncEvent event) |
This method indicates that a startAsync() method of ServletRequest type is invoked to generate new asynchronous cycle. |
void onTimeout(AsyncEvent event) |
This method indicates that an asynchronous operation has been expired. |
Example of Asynchronous Event and Listener
DemoServlet.java
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import javax.servlet.annotation.*; @WebServlet(asyncSupported = true, value = "/serv") public class DemoServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException{ response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.println("<html><body><center>"); out.println("<h1>Asynchronous Event and Listener Example</h1>"); out.println("<h2>Before startAsync() method is invoked<h2>"); out.println("<br><h3>Is Async Started : " + request.isAsyncStarted()+"</h3>"); AsyncContext ac = request.startAsync(); ServletRequest req = ac.getRequest(); boolean b = req.isAsyncStarted(); out.println("<br><h2>After startAsync() method is invoked</h2>"); out.println("<br><h3>Is Async Started : "+b+"</h3>"); out.println("<center></body></html>"); ac.complete(); ac.addListener(new AsyncListener() { @Override public void onComplete(final AsyncEvent event) throws IOException { System.out.println("Async Completed"); } @Override public void onTimeout(final AsyncEvent event) throws IOException { System.out.println("Async Timeout"); } @Override public void onError(final AsyncEvent event) throws IOException { System.out.println("Async generates an Error"); } @Override public void onStartAsync(final AsyncEvent event) throws IOException { System.out.println("Async Started"); } }); }}Output:
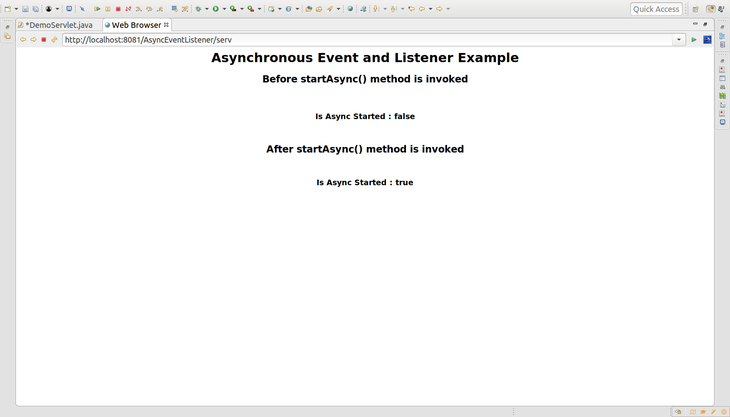
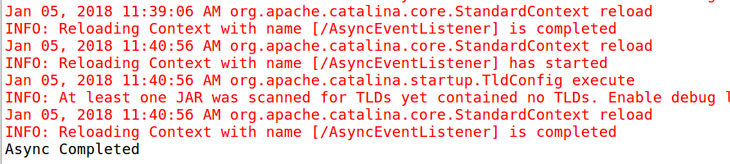