Excel VBA CBool Function
VBA CBool Function: The CBool function in VBA calculates an expression and returns the result as a Boolean data type.
Syntax
CBool (Expression)
Parameter
Expression (required) – This parameter represents the expression that that you want to convert to a Boolean.
Return
This function returns the result as a Boolean data type.
Example 1
Sub CBoolFunction_Example1() Dim val1 As Integer, val2 As Integer, val3 As Integer Dim bool1 As Boolean, bool2 As Boolean val1 = 11 val2 = 15 val3 = 11 bool1 = CBool(val1 = val2) ' bool1 is now equal to False Cells(1, 1).Value = bool1 bool2 = CBool(val1 = val3) ' bool2 is now equal to True Cells(2, 1).Value = bool2 End Sub
Output
FALSE |
TRUE |
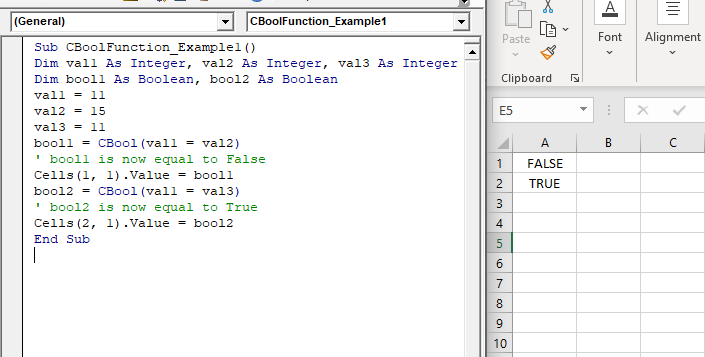
Example 2
Sub CBoolFunction_Example2() ' Converting Integer value into Boolean Dim bool As Boolean bool = CBool(0) ' bool1 is now equal to False Cells(1, 1).Value = bool End Sub
Output
FALSE
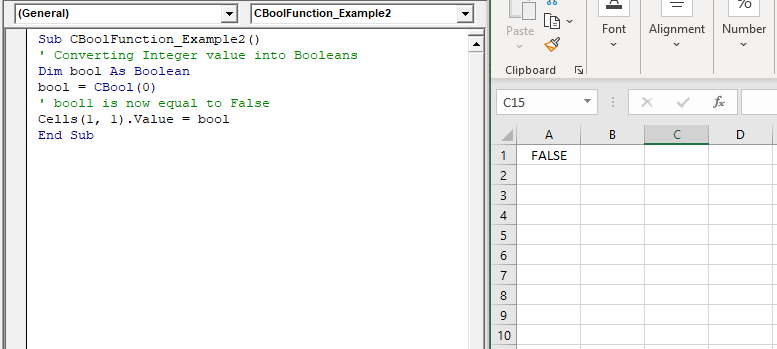
Example 3
Sub CBoolFunction_Example3() ' Converting Integer value into Boolean Dim bool As Boolean bool = CBool(-11.8) ' bool1 is now equal to True Cells(1, 1).Value = bool End Sub
Output
True
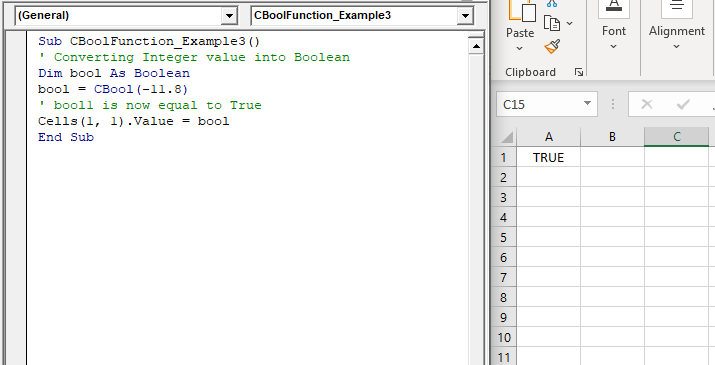