Excel VBA DateSerial Function
The DateSerial function in VBA returns a Date from a supplied year, month, and day number.
Syntax
DateSerial (Year, Month, Day)
Parameter
Year (required) – This parameter represents an integer signifying the year.
Month (required) – This parameter represents an integer signifying the month. If the Integer values are less than 1 or greater than 12, the values are interpreted as:
- -1 – November of the previous year
- 0 – November of previous year
- 13 – January of the following year
- 14 – February of the following year
Day (required) – This parameter represents an integer signifying the day of the month. If the integer values less are than 1 or greater than the number of days in the current month are interpreted as follows:
- -1 – It signifies the second to the last day of the previous month
- 0 – It signifies the last day of the previous month.
- Days in current month + 1 – It signifies the first day of the following month
- Days in current month + 2 – It signifies the second day of the following month.
Return
This function returns a Date from a supplied year, month, and day number.
Example 1
Sub DateSerialFunction_Example1() ' Two different ways to return the date "12/31/2015" Dim date_val1 As Date, date_val2 As Date date_val1 = DateSerial(2020, 6, 31) date_val2 = DateSerial(20, 6, 31) ' The variables date_val1 and date_val2 are equal to the Date 12/31/2015. Cells(1, 1).Value = date_val1 Cells(2, 1).Value = date_val2 End Sub
Output
7/1/2020 |
7/1/2020 |
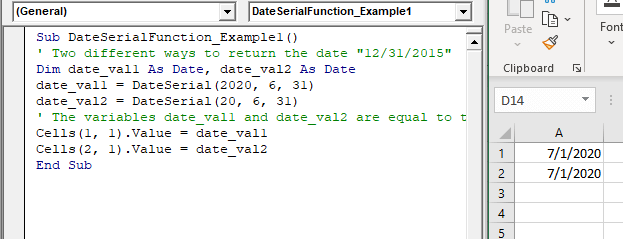
Example 2
Sub DateSerialFunction_Example2() ' Demonstrating two different ways to return the date "12/31/2020" Dim date_val1 As Date, date_val2 As Date date_val1 = DateSerial(2020, 12, 31) date_val2 = DateSerial(2020, 13, 31) ' The variables date_val1 will return 12/31/2020 ' The date_val2 are equal to the Date 1/31/2021. Cells(1, 1).Value = date_val1 Cells(2, 1).Value = date_val2 End Sub
Output
12/31/2020 |
1/31/2021 |
Example 3
Sub DateSerialFunction_Example3() ' Demonstrating two different ways to use Day Numbers That Are Less Than 1 Or Greater Than 31 Dim date_val1 As Date, date_val2 As Date date_val1 = DateSerial(2020, 12, 1) date_val2 = DateSerial(2020, 12, 32) ' The variables date_val1 will return 12/1/2020 ' The date_val2 are equal to the Date 1/1/2021. Cells(1, 1).Value = date_val1 Cells(2, 1).Value = date_val2 End Sub
Output
12/1/2020 |
1/1/2021 |
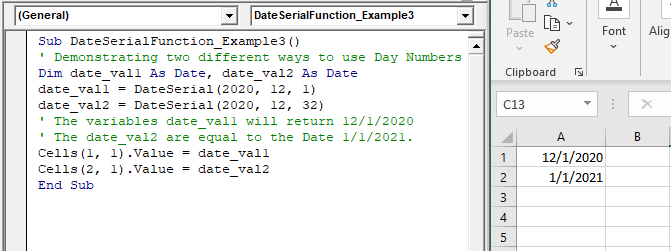