Operational Controls Methods in Selenium WebDriver
Operational Controls Methods
The Operational controls are used to perform all the actions on the web elements like click on the button, pass the value in the text box, and click on the submit button, etc.
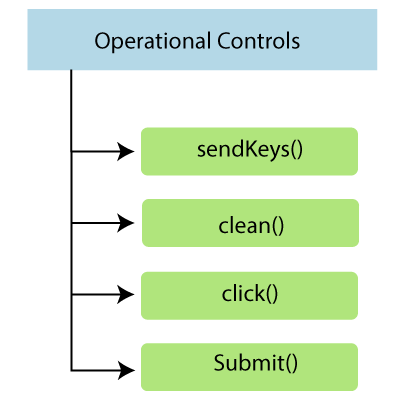
Some of the most commonly used operational controls are as follow:
Operational Controls | Description | Syntax | Command |
sendKeys() | It is used to pass data into textbox or text area. | sendKeys(String) : void | element.sendKeys("text"); |
click() | It is used to perform left click operation on link, checkbox, radio button, image element. | click() : void | element.click(); |
clear() | It is used to clear or reset existing data from the edit box. | clear() :void | element.clear(); |
submit() | It is used to perform click operation only on the submit button. | Submit() :void | element.submit(); |
Let us take a sample test script in which will try to cover mostly used operational_controls Commands:
For our testing purpose, we are using one e-commerce website Myntra, to perform operational commands of the web element controls.
In this test, we will automate the following test scenarios:
Steps | Action | Method Used | Input | Excepted Result |
1. | Invoke the Google Chrome Browser. | System.setProperty() | The Chrome browser must be opened. | |
2. | Navigate to the Myntra home page. | get() | www.myntra.com | Myntra home page must be displayed. |
3. | Pass the date using send keys methods in the search box. | sendKeys() | Puma shoe page | The passed Data should be opened with a particular page. |
4. | Click on the search key button using the click method. | click() | The Search key button should be clicked. | |
5. | Clear the date on the search box using the clear method. | clear() | The Data should be cleared in the text box. | |
6. | Close the browser | close() | The browser should be closed. |
Open the Eclipse IDE and the existing test suite new_test, which we have created in WebDriver Installation section of WebDriver tutorial.
Then, right-click on the src folder and create a new Class File from New > Class.

- Give the Class name as operational_controls and click onthe Finish button.

We are creating our test case step by step to give you a complete understanding of Operational_controls Commands in WebDriver.
Step1:
To launch the Google Chrome browser, we need to download the ChromeDriver.exe file and set the system property to the path of ChromeDriver.exe file.
Here is the code for setting the system property for the google chrome:
//set the system property of Google Chrome System.setProperty("webdriver.chrome.driver","C:\\Users\\JTP\\Downloads\\chromedriver_win32\\chromedriver.exe");
To initialize the Chrome driver using ChromeDriver class:
// create driver object for CHROME browser WebDriver driver=new ChromeDriver();
Step2:
Launch the website using get() method.
//navigate to myntra home page driver.get("https://www.myntra.com");
Step3:
Pass the date using sendkeys() methods in the search box.
// using a send keys method to pass the data into the search box driver.findElement(By.className("desktop-searchBar")).sendKeys(" puma shoes"); //or can also be written like this: WebElement wb=driver.findElement(By.className("desktop-searchBar")); wb.sendKeys("puma shoes");
Step4:
Click on the search key button using the click() method.
//using click method to click on the particular button driver.findElement(By.className("desktop-submit")).click(); //or written like this WebElement wb1=driver.findElement(By.className("desktop-submit")); wb1.click(); // print to check whether the method is working fine or not System.out.println("passed");
Step5:
Clear the date on the search box using the clear() method.
//using the clear method to clear the search box data. driver.findElement(By.className("desktop-searchBar")).clear(); System.out.println("passed1");
Step6:
Finally, we terminate the process and close the browser.
//Close the browser driver.close();
After that, our final test script will look like this:
package testpackage; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class webelement_controls { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\\Users\\JTP\\Downloads\\chromedriver_win32\\chromedriver.exe"); // create driver object for CHROME browser WebDriver driver=new ChromeDriver(); //open the URL driver.get("https://www.myntra.com"); // using send keys method to pass the date into the textbox driver.findElement(By.className("desktop-searchBar")).sendKeys(" puma shoes"); //using click method to act on left-click operation driver.findElement(By.className("desktop-submit")).click(); // print to check whether the method is working fine or not System.out.println("passed"); //used clear method to clear the data from the textbox driver.findElement(By.className("desktop-searchBar")).clear(); System.out.println("passed1"); //close the existing browser driver.close(); } }
- To run the test script in Eclipse, right-click on the window and then click Run as > Java application.
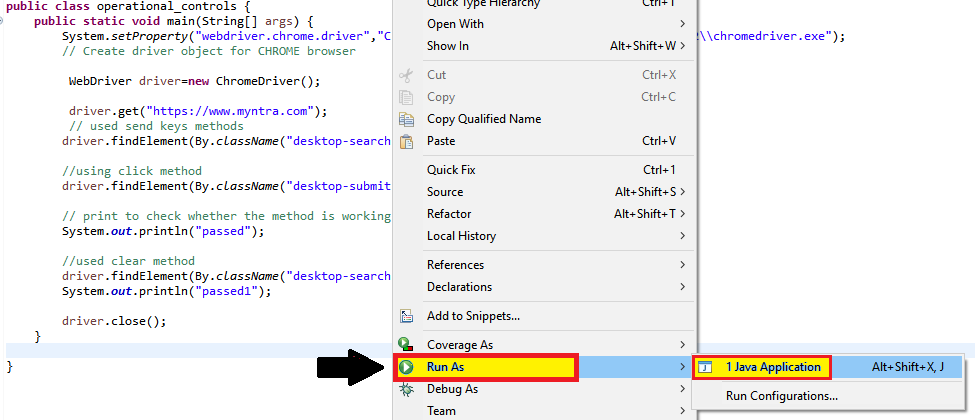
- The test script will be launched in the chrome browser and automate all the test scenarios.