AngularJS $httpParamSerializer Service
AngularJS, a JavaScript framework developed by Google, provides a set of powerful services to simplify common tasks in web development. One such service is the $httpParamSerializer, designed to facilitate the serialization of JavaScript objects into URL-encoded strings, particularly for HTTP requests. In this extensive guide, we'll delve into the details of the $httpParamSerializer service, exploring its features, use cases, examples, and best practices.
Understanding $httpParamSerializer
The $httpParamSerializer service is a part of the $http service in AngularJS, specifically tailored for handling the serialization of parameters in HTTP requests. Its primary function is to convert JavaScript objects into a URL-encoded string, making it suitable for various scenarios, such as submitting form data or constructing query parameters for API requests.
Understanding the Core Features
Object Serialization:
At the heart of $httpParamSerializer lies the fundamental capability of serializing JavaScript objects into a format amenable for inclusion in URLs or HTTP requests. This process involves encoding the object's properties and values, adhering to the application/x-www-form-urlencoded content type. This feature provides a standardized and interoperable way to transmit data between client and server.
Nested Objects Support:
The service goes beyond basic object serialization, offering seamless support for nested objects. This functionality empowers developers to represent complex data structures within their applications. Nested objects can be serialized and transmitted via HTTP requests, ensuring that the richness of data structures is maintained throughout the communication process.
Array Serialization:
Arrays, often integral to data structures, are handled with finesse by $httpParamSerializer. The service ensures that arrays within JavaScript objects are properly encoded, guaranteeing their accurate representation in URLs or HTTP request bodies. This capability proves crucial in scenarios where arrays play a pivotal role, such as when dealing with multiple selections in form submissions.
Custom Serialization:
Flexibility is a hallmark of $httpParamSerializer. Developers are granted the ability to exert control over the serialization process by introducing custom serializer functions. This empowers fine-tuning of the serialization of specific object properties, catering to the nuanced requirements of diverse applications. Customization ensures adaptability to varying data structures and encoding needs.
Practical Application in Varied Scenarios
Form Data Submission:
A fundamental application of $httpParamSerializer lies in facilitating the seamless submission of form data. In scenarios where users interact with web forms, the form data, encapsulated within a JavaScript object, undergoes serialization using this service before embarking on its journey to the server through an HTTP request. This process is foundational for applications reliant on user inputs, ensuring the efficient transmission of data and fostering a responsive user experience.
Query Parameters in API Requests:
API interactions often demand the inclusion of parameters in URLs to enable actions such as filtering, sorting, or pagination. $httpParamSerializer plays a pivotal role in simplifying this process by efficiently converting JavaScript objects into query parameters. This feature is indispensable in the realm of web development, where constructing API requests with clear and concise parameters is a common requirement for robust data retrieval.
URL Construction:
In the dynamic landscape of AngularJS applications, URL construction is a dynamic and crucial task. The $httpParamSerializer service excels in ensuring that parameters are meticulously encoded, adeptly handling special characters and spaces. This feature contributes to the creation of robust and reliable URLs, crucial for a seamless user experience. The service's ability to handle URL construction ensures the integrity of data transmission in diverse application scenarios.
Scaling the Service for Real-World Demands
As applications evolve and expand in complexity and scale, $httpParamSerializer continues to be a stalwart ally, adapting to the evolving demands of real-world development. Its versatile features and use cases lay the groundwork for addressing diverse challenges encountered in web application development. The service proves invaluable in scenarios where data transmission efficiency is crucial, especially in applications dealing with large datasets or complex user interactions.
Best Practices for Optimal Utilization
Encoding Behavior:
Developers are encouraged to familiarize themselves with the default encoding behavior of $httpParamSerializer. This understanding is pivotal for ensuring harmonious collaboration with server-side processing and maintaining compatibility with the expected content type. A clear comprehension of encoding nuances aids developers in preemptively addressing potential issues related to data transmission.
Custom Serialization Logic:
The flexibility offered by $httpParamSerializer extends to the incorporation of custom serialization logic. When grappling with intricate data structures or specific encoding requirements, developers can strategically leverage custom serializer functions. This empowers them to tailor the serialization process to the unique needs of their applications, enhancing flexibility, and adaptability in handling diverse data scenarios.
Code Organization:
Promoting code organization and maintainability is paramount in a dynamic development environment. Encapsulating the usage of $httpParamSerializer within dedicated services or utility functions is considered a best practice. This approach ensures consistency in approach across an application, making it easier to manage changes and updates. Well-organized code enhances collaboration among development teams and fosters a structured development process.
Form Data Submission
Consider a scenario where a user submits a form on a web page. The form data, stored in a JavaScript object, needs to be sent to the server using an HTTP POST request.
// Here, we are declaring the sample form data object
const formData = {
username: 'john_doe',
password: 'securepass123',
email: '[email protected]',
};
// Here, we are using $httpParamSerializer to serialize the form data
const serializedFormData = $httpParamSerializer(formData);
// Here, we are sending an HTTP POST request with the serialized data
$http.post('/api/user', serializedFormData).then(response => {
// Handle the server response
});
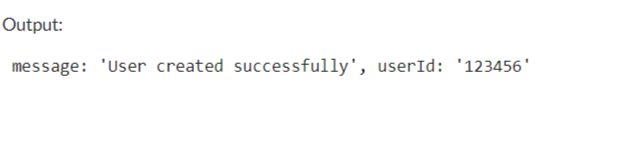
API Request with Query Parameters
In an API request scenario, suppose we want to fetch a list of users with specific filtering criteria.
// Query parameters object
const queryParams = {
role: 'admin',
isActive: true,
};
// Using $httpParamSerializer for query parameter serialization
const serializedParams = $httpParamSerializer(queryParams);
// Constructing the API request URL
const apiUrl = `/api/users?${serializedParams}`;
// Sending an HTTP GET request to fetch users
$http.get(apiUrl).then(response => {
// Process the list of users
});
URL Construction
When dynamically constructing URLs within an AngularJS application, the $httpParamSerializer service ensures that the parameters are properly encoded, taking care of special characters and spaces.
Practical Examples
Let's explore additional practical examples to illustrate how $httpParamSerializer can be used in real-world scenarios.
Dynamic URL Construction
Consider a scenario where the construction of URLs involves dynamic parameters based on user input.
// User-selected filters
const filters = {
category: 'technology',
priceRange: '100-500',
};
// Serialize filters for URL inclusion
const serializedFilters = $httpParamSerializer(filters);
// Constructing a dynamic URL for product listings
const dynamicUrl = `/products?${serializedFilters}`;
// Making an HTTP GET request with the dynamic URL
$http.get(dynamicUrl).then(response => {
// Process the product listings
});
Custom Serialization Logic
When dealing with complex data structures, custom serialization logic can be applied to specific properties of the JavaScript object.
// Custom serializer function for a complex object
function customSerializer(key, value) {
if (key === 'customProperty') {
// Custom logic for serializing 'customProperty'
return value.toUpperCase();
}
return value;
}
// Sample object with a custom property
const complexObject = {
name: 'John Doe',
age: 30,
customProperty: 'customValue',
};
// Using $httpParamSerializer with custom serialization logic
const serializedObject = $httpParamSerializer(complexObject, customSerializer);
// Sending an HTTP request with the serialized object
$http.post('/api/custom', serializedObject).then(response => {
// Handle the server response
});
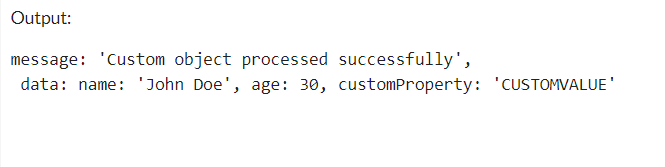
Limitations and Considerations
While the $httpParamSerializer service in AngularJS offers powerful capabilities for parameter serialization, it is essential for developers to be aware of its limitations and considerations. Understanding these aspects ensures informed decision-making and the adoption of alternative strategies when necessary.
Large Data Sets:
Limitation:
The URL-encoded format generated by $httpParamSerializer may not be the most efficient choice when dealing with large amounts of data, especially in scenarios like file uploads or complex datasets.
Consideration:
For substantial data sets, developers should evaluate alternative serialization methods that better suit the nature of the data. JSON serialization, for example, might be more efficient in such cases.
Content Type Considerations:
Limitation:
The serialization performed by $httpParamSerializer is tailored for the application/x-www-form-urlencoded content type. If your application requires a different content type, such as JSON, the service may not be the most appropriate choice.
Consideration:
In situations where the content type is crucial, developers should explore alternative serialization methods that align with the specific requirements of the application. AngularJS provides other services, like $httpParamSerializerJQLike or manual JSON serialization, to address diverse content types.
Security Considerations:
Limitation:
While $httpParamSerializer handles the encoding of parameters, it doesn't inherently provide security features against common web vulnerabilities, such as Cross-Site Scripting (XSS) attacks.
Consideration:
Developers should implement additional security measures, such as input validation, output encoding, and secure communication practices, to mitigate security risks. Careful handling of user inputs and proper sanitation is crucial to prevent security vulnerabilities.
Handling Special Characters:
Limitation:
Encoding behavior for special characters might not align with the specific requirements of certain server-side processing or APIs.
Consideration:
Developers should be attentive to how special characters are encoded and consult relevant documentation to ensure compatibility with the server-side processing. Custom serialization logic can be employed to address specific character encoding needs.