Creational design patterns in Python
We create new objects using creational design patterns. It is more concerned about the way of creating it. However, constructors are rarely used and often hidden in some classes. The main reason behind the creation of objects is changing the class that fits the situation. Constructors are inadequate to return different instances of different classes, so we use alternatives. Here inheritance is used to vary the classes of objects. Creating objects by the conventional method can make difficulties for design or might create problems, but here we can control the object creation. Sometimes the nature of the object might change along with the change in classes, so in this case, the creational design patterns provide a flexible approach to handle this situation. Two main ideas that are being used in creational design patterns are encapsulating and hiding how these are created and combined.
- It promotes the use of interfaces and reduces coupling.
- Encapsulation of all the details of the object is performed.
- A simple and controlled mechanism of object creation is performed.
There are mainly six approaches used in creational design patterns-
- Factory design pattern.
- Singleton design patterns.
- Object pool pattern.
- Builder pattern.
- Abstract factory pattern.
- Prototype pattern.
We will discuss all of them one by one.
Factory design patterns
The factory method is used when the client-side object does not know which class to instantiate. It is a creational design pattern that defines or makes an abstract class for creating an object but allows to alter the type of created objects. This method can create instances of the appropriate classes by using the family hierarchy of classes. It can be one part of the client class, or it can also have a separate class. As we have already discussed, design patterns are used to solve the recurring problem, so let's try to understand the factory method by an example problem where you can understand the practical approach where it can be used.
Problem -
Suppose you have a logistics management application for the very basic taxi management. Then after some months, your app started gaining popularity. You received many requests to add a few more transportation methods to your app, so you decided to add some more transportation methods .firstly, you only have taxi objects. Then you thought to add a few more objects like trucks and bikes, and then you have to make changes in the whole codebase. After some time, you try to add sea transport to your app now again, you have to change the whole codebase, and the result of adding a few things and changing the codebase multiple times can make your codebase a mess and nasty. It can affect the behaviour of the app too.
Solution-
The solution that developers use throughout the world is the factory method. The factory pattern method suggests replacing the constructor call with a special factory method. The new() keyword is used for the creation of objects. We have moved the constructor to another instance of the program, and now we can easily change the product class, and the subclass can also be changed.
Limitation: the objects must have some basic property to return the proper object and appropriate class.
Where factory method can be used -
- When you need to extend the object to a subclass.
- The product changes over time, but the client remains the same or unchanged.
- We will use the factory method when a product does not know the exact subclass.
Example using python
class French_Localizer:
def __init__(self):
self.translations = {"girl": "fille", "boy": "garcon",
"men":"hommes","women":"femmes",}
def localize(self, msg):
return self.translations.get(msg, msg)
class dutch_Localizer:
def __init__(self):
self.translations = {"girl": "meisje", "boy": "jongen",
"men":"heren","women":"dames",}
def localize(self, msg):
return self.translations.get(msg, msg)
class greekLocalizer:
def __init__(self):
self.translations = {"girl": "????ts?", "boy": "?????",
"men":"??d?e?","women":"???a??a",}
def localize(self, msg):
return self.translations.get(msg, msg)
class irish_Localizer:
def __init__(self):
self.translations = {"girl": "chailín", "boy": "buachaill",
"men":"fir","women":"mná",}
def localize(self, msg):
return self.translations.get(msg, msg)
class English_Localizer:
def localize(self, msg):
return msg
def Factory(language ="English"):
localizers = {
"French": French_Localizer,
"English": English_Localizer,
"dutch": dutch_Localizer,
"greek": greekLocalizer,
"irish": irish_Localizer,
}
return localizers[language]()
if __name__ == "__main__":
f = Factory("French")
e = Factory("English")
d = Factory("dutch")
z = Factory("greek")
i = Factory("irish")
message = ["girl", "boy", "men","women"]
for msg in message:
print(e.localize(msg))
print(f.localize(msg))
for msg in message:
print(d.localize(msg))
print(z.localize(msg))
print(i.localize(msg))
Output :
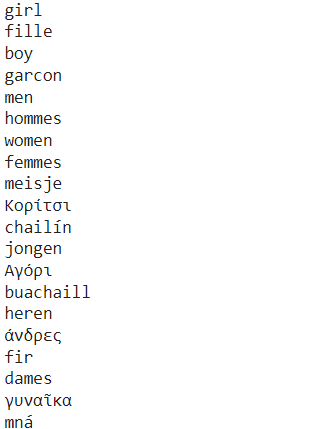
Explanation: It is a very simple code where we have utilized the factory method in an ordinary fashion just for example so here you can see a total of five classes are used and we have used factory method to add these and use as subclass just look at the code below-
if __name__ == "__main__":
f = Factory("French")
e = Factory("English")
d = Factory("dutch")
z = Factory("greek")
i = Factory("irish")
If you want to add more languages to it, it is very simple. You just have to add it in the localizer and use the factory method to add this in the subclass, and that language will be inserted very easily.