Singleton design pattern in python
It is a very simple design pattern. Here we create only a single instance of the class. In other words, we only create a single object. We have to make sure that no other object is created. The class can directly access its only object and does not need to instantiate the object to access it. It has only one private constructor to restrict the instantiation of the class. It provides a global access point to access or get the object of the class. Apart from python, it is also used in core java. This method can be implemented in python in many different. singleton breaks modularity of code
You might think about the practical use of this design pattern method. It has many implementations. One of them is explained here, so try to understand the example below-
You might end up creating a mess because it can make things complex. Suppose you have a module, so there should be only one configuration or error manager for multiple configuration files for some module. The entire module should have only one configuration manager for DB connections. There should only be one DB connection.
There are two types of instantiation -
- Lazy instantiation
- Early instantiation.
Lazy instantiation: here object is created when needed, with no pre-creation of the object.
Example -
public class Lazy_Initialized_Singleton_ex {
private static LazyInitializedSingleton_example instance;
private Lazy_Initialized_Singleton_ex(){}
public static Lazy_Initialize_Singleton_ex getInstance(){
if(instance == null){
instance = new Lazy_Initialized_Singleton_ex();
}
return instance;
}
}
Output:

Explanation: we know if we will declare any class as static so we can access it without instantiating it. So here, as you can see, we have declared Lazy_Initialize_Singleton_ex getInstance() as static so we can access it without instantiating it, and the same object will be returned whenever it is called.no, the object will be created until it is called, and that why it is called lazy instantiation.
[this example is in java just for the sake of explanation]
Early instantiation: here, we create an object before it is called or needed.
Different ways to implement singleton design patterns in python -
- Module-level singleton method
- Classic singleton pattern method
- Borg singleton pattern method
Module-level singleton method: here, all modules will be a singleton. Let us understand with an example.
Create three python files named example1, example 2 and example3.
Example1.py code:
example_variable = "javaTpoint"
Example2.py code:
import Example1
print(singleton.example_variable)
singleton.example_variable += "(modified by example2)"
Example3.py code:
import Example1
print(singleton.example_variable)
Output:
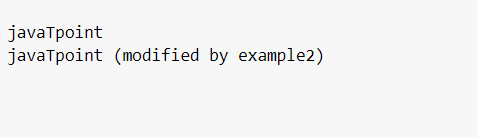
Explanation: here, as we know, only a single instance will be created, and that is why firstly, example_variable was"javaTpoint", and when we modify it in example2, we print it again in example3. This will print the updated variable. If you change the variable in any file, the changes will be reflected irrespective of any specific file.
Classic singleton pattern method
Here it will create an instance only once, which means if the instance is already created, it will not create any instance even after calling it. It will return the previously created instance wherever it will be called.
Lets understand it by an example :
class Singleton_Class(object):
def __new__(cls):
if not hasattr(cls, 'instances'):
cls.instances = super(Singleton_Class, cls).__new__(cls)
return cls.instances
singleton_ex = Singleton_Class()
new_singleton_ex = Singleton_Class()
print(singleton_ex is new_singleton_ex)
singleton_ex.singl_variable = "Singleton Variable"
print(new_singleton_ex.singl_variable)
Output:
True
Singleton Variable
Explanation: here, we have called the method twice, and it returns true, meaning no changes happened.
Let us verify whether the objects have the same instances or not by checking it practically -
Example.py code :
class Singleton_Meta(type):
_instances = {}
def __call__ex(cls, *ar_gs, **kw_args):
if cls not in cls._.instances:
instance = super().__call__ex(*ar_gs, **kw_args)
cls._.instances[cls] = instance
return cls._.instances[cls]
class Singleton(metaclass=Singleton_Meta):
def some_business_logic(self):
if __name__ == "__main__":
s12 = Singleton()
s23 = Singleton()
if id(s12) == id(s23):
print("Singleton works, both variables contain the same instance.")
else:
print("Singleton failed, variables contain different instances.")
Output :

Explanation:
This example clearly shows that objects in the singleton method have the same instances. Here we have given a name to our class as Singleton_Meta and wrote some code just for creating instances, and then we have called the singleton method twice, once for s12 and then for s23. Here instance will be created for both the call. Lastly, we compare both instances. If both are the same, the output will be "Singleton works, both variables contain the same instance". Else it would be "Singleton failed, variables contain different instances.". After executing the following code in the terminal, we got the output as above, which verifies that in singleton method creates only one instance no matter how many times it is called.
This was all about the Classic singleton pattern method
Borg singleton method :
It has one interesting feature that is distinct from the above two methods. It allows state sharing. Let us understand this by one practical example.
Example :
class Borg_Singleton(object):
_shared_borg_state = {}
def __new__(cls, *ar_gs, **kw_args):
obj = super(Borg_Singleton, cls).__new__(cls, *ar_gs, **kw_args)
obj.__dict__ = cls._shared_borg_state
return obj
borg = Borg_Singleton()
borg.shared_variable = "yes Shared Variable actually"
class ChildBorg_ex(Borg_Singleton):
pass
childBorg = ChildBorg_ex()
print(childBorg is borg)
print(childBorg.shared_variable)
Output:
False
yes Shared Variable actually
Explanation:
Here we have created one class named Borg_Singleton, and inside this, we have written similar code as previous to achieve the creation of instances. We have tried to share state borg = Borg_Singleton(), and then we have compared both the state and both are not equal because the state is shared and the output is false. You can reset it and commit the new changes if you want a different state.
Use cases of the singleton method :
- We can implement different interfaces using Singleton.
- This can be passed as a parameter.
- We can control it polymorphically.
- It can be used in file management.
- Database connections can be managed using the singleton design method.
So here, I hope you have gained a basic understanding of using singleton design patterns.