Numpy Attributes
Numpy Attributes
The Numpy collections give attributes to the numpy arrays. These attributes assist us with knowing the shape, aspect, and different properties of a given numpy array. As we know, it is a Python library consisting of multidimensional array objects. The quantity of components in each aspect is named as the state of an array. NumPy arrays have a characteristic considered shape that profits a tuple, with each record having the quantity of comparing components. This can likewise be utilized to resize the array
ndarray.shape
This array property returns a tuple comprising array aspects. It can likewise be utilized to resize the array. The ndarray.shape characteristic returns the element of the array. A tuple of whole numbers demonstrates the size of your NumPy array. For example, if you make a matrix with a rows and b columns, the shape will be (a * b).
Example 1
import numpy as np
int = np.array ([[2,3,4],[5,6,7]])
print ( int. shape)
Output
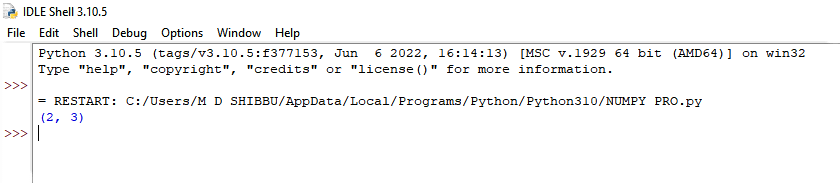
In the above example, we first imported numpy as np by using features of the numpy library in Python. After that, we implemented arrays, and we assigned that to a variable named int.In the last line of code, we have printed the shape of the np.array by utilizing int.shape. It will give the shape of the array.
Example 2
#This is how we can resize the ndarray
import numpy as np
int = np.array([[2,3,4],[5,6,7]])
int.shape = (3,2)
print (int)
Output
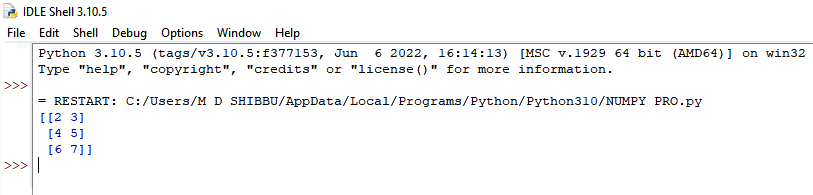
In example 2, we have also imported numpy as np. But here, we have resized the shape of the array by assigning the value to a.shape.
NumPy library also gives us a reshape function to resize an array.
import numpy as np
int = np.array([[2,3,4],[5,6,7]])
b = int.reshape(3,2)
print (b)
Output
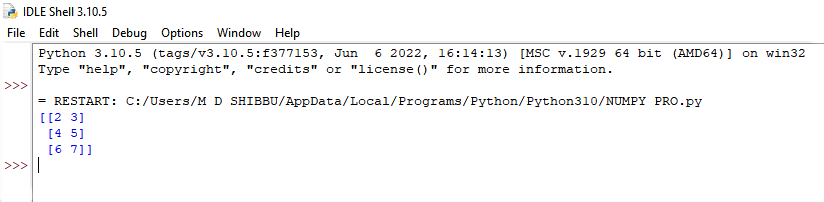
In the numpy library, we also have a reshape function through which we can change the shape of the array. Now we have two methods to reshape the dimensions of the array.
ndarray.ndim
This array attribute provides the number of array dimensions. These characteristic assists track down the quantity of components of a NumPy with arraying. For example, 1 implies that the array is 1D,2 means that the array is 2D, etc.
Example 1
# array of even numbers
import numpy as np
inte = np.arange(28)
print (inte)
Output
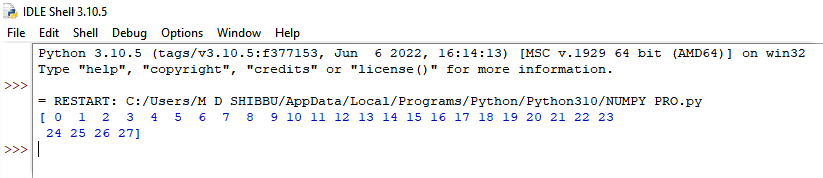
In the above example, we have used np.arange to check the number of array dimensions from 0 to 28. It will provide all the array dimensions from 0 to 28.
Example2
# the one-dimensional array
import numpy as np
ara = np.arange(24)
ara.ndim
# now we are reshaping it
b = ara.reshape(2,4,3)
print (b)
Output
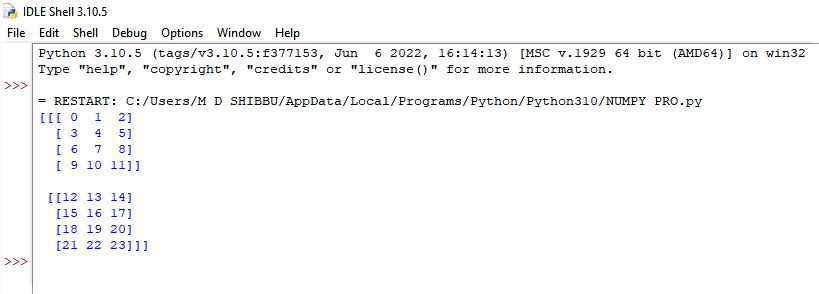
We can also reshape the array dimension after arranging the dimensions. Reshaping an array implies changing the state of an array. By this, we can add or eliminate aspects or change the number of components in each aspect.
ndarray.T
The T attribute is used to get the transpose of an array.
import numpy as np
ara = np.array([[1, 2, 3], [4, 5, 6]])
print("ara is:")
print(ara)
print("\nTranspose of ara is:")
print(ara.T)
Output
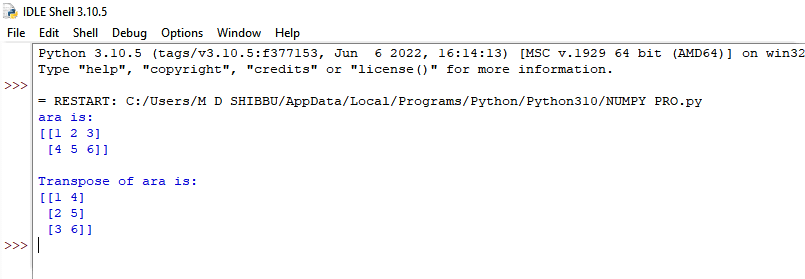
Size: This attribute computes the complete number of components present in the NumPy array.
dtype: This characteristic assists with checking what kind of components are put away in the NumPy array.
itemsize: This characteristic assists with finding the length of every component of the NumPy array in bytes. For instance, if you have whole number qualities in your array, this characteristic will bring 8 back 8 as whole number qualities and take 8,8-bits in memory.
Information: This characteristic is a cushioned object that focuses on the beginning of the NumPy array. Be that as it may, this characteristic isn't involved much since we mostly access the components in the array utilizing records.
Let us understand these numpy attributes by using a single program. In this program, we have implemented all the numpy attributes.
import numpy as np
fst_array = np.array([2,3,4])
sec_array = np.array([[2,3,4],[5,6,7]])
print(" The First array is: ",fst_array)
print(" The Second array is: ",sec_array)
print("\nThe number of dimensions in first array: ",fst_array.ndim)
print("The number of dimensions in second array: ",sec_array.ndim)
print("\nThe shape of array first array: ",fst_array.shape)
print("The shape of array second array: ",sec_array.shape)
print("\nThe itemsize of first array: ",fst_array.itemsize)
print("The itemsize of first array: ",sec_array.itemsize)
print("\nThe data of first array is: ",fst_array.data)
print("The data of second array is: ",sec_array.data)
print("\nThe data type of first array: ",fst_array.dtype)
print("The data type of second array: ",sec_array.dtype)
print("\nThe size of first array: ",fst_array.size)
print("The size of second array: ",sec_array.size)
Output
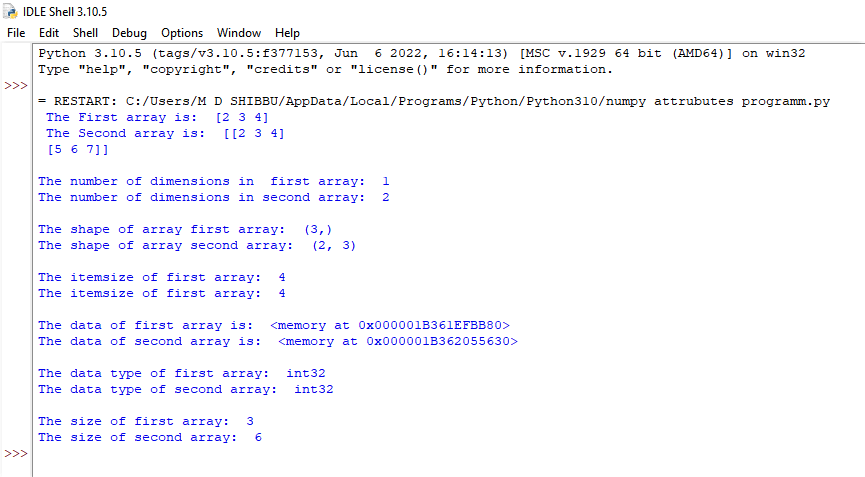