OpenGL Drawable: GLCanvas
GLCanvas is the heavy-weight AWT component. This class is the subclass of java.awt.Canvas class.
JOGL Canvas with AWT Frame:
Steps to create JOGL basic frame using GLCanvas and AWT Frame -
- Create a new java project give the name to it and click on Finish Button.
- Add jars files required for JOGL application.
- Create a new class in the package. The class must implement GLEventListener interface. For that we have to import the package java.media.opengl. Then we can implement all four methods, init(), reshape(), display() and dispose(). We are creating the basic frame. So, we can keep the methods blank.
- Create the object of GLProfile class. Use the get() method of GLProfile class and mention proper OpenGL API. We are using GL2. GLProfile glp = GLProfile.get ( GLProfile.GL2 );
- Create the GLCapabilities class using constructor and pass GLProfile class object to it. GLCapabilities cap = new GLCapabilities ( glp );
- Create the GLCanvas object using the constructor and pass GLCapabilities object to it. GLCanvas canvas = new GLCanvas ( cap );
- The addGLEventListener() method is used to add GLEventListener to the canvas. BasicFrame bf = new BasicFrame(); canvas.addGLEventListener ( bf );
- Size of canvas can be decided using setSize() method canvas.setSize (400,400);
- Create AWT frame object using constructor. Canvas can be added to frame. The add() method is used to add GLCanvas object to frame. Using setVisible(true) method we can activate the frame. Frame f = new Frame(“ Simple Frame ”); f.add ( canvas ); f.setVisible ( true );
- In order to view the frame clearly, setSize() method is used with Frame class Object to set its dimensions.
Creating Simple Frame using GLCanvas in JOGL –
Code:
import javax.media.opengl.GLAutoDrawable; import javax.media.opengl.GLEventListener; import javax.media.opengl.GLProfile; import javax.media.opengl.GLCapabilities; import javax.media.opengl.awt.GLCanvas; import java.awt.Frame; public class SimpleFrame implements GLEventListener { @Override public void display(GLAutoDrawable drawobj) { } @Override public void dispose(GLAutoDrawable drawobj) { } @Override public void init(GLAutoDrawable drawobj) { } @Override public void reshape(GLAutoDrawable drawobj, int x, int y, int width, int height) { } public static void main(String[] args) { GLProfile glp = GLProfile.get (GLProfile.GL2); GLCapabilities cap = new GLCapabilities(glp); GLCanvas canvas = new GLCanvas(cap); BasicFrame bf = new BasicFrame(); canvas.addGLEventListener(bf); canvas.setSize(500, 500); Frame frame = new Frame (" Simple Frame using GLCanvas "); frame.add(canvas); frame.setSize( 580, 580 ); frame.setVisible(true); } }
Output:
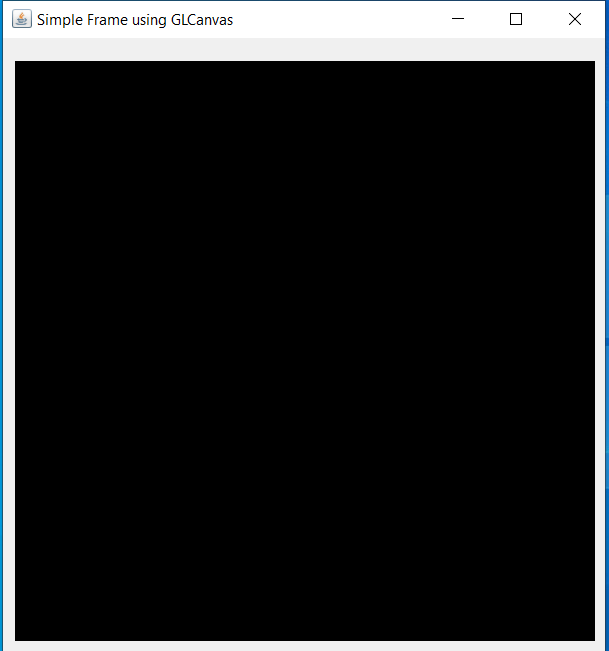
Explanation:
Step 1: For constructing the basic frame using GLCanvas we have to import all the required classes and interfaces in packages such as GLAutoDrawable, GLEventListener, GLProfile, GLCapabilities, GLCanvas. Frame class in java.awt is also imported to use awt components.
Step 2: We have to declare a class and implement GLEventListener interface to override 4 required methods that is init(), display(), reshape(), and dispose().
Step 3: Then we have to create a object of GLProfile class which is passed as an argument to GLCapabilities constructor. The GLCapabilities object is passed as the argument to GLCanvas constructor.
Step 4: BasicFrame object is created and then addEventListener method is called with canvas object.
Step 5: setSize() method is then called with canvas object to set size of canvas.
Step 6: Then Frame constructor is called with title passed in it.
Step 7: The canvas can be added to the frame. The add() method is used to add GLCanvas object to the frame. frame.getContentPane().add(canvas) is used to add our canvas inside the frame.
Step 8: Using frame.setSize() we can set the size of the frame and using setVisible(true) method, we can make the frame visible to the user.
JOGL Canvas with Swing JFrame:
Steps to create JOGL basic frame using GLCanvas and Swing JFrame -
- Create a new java project give name to it and click on Finish Button.
- Add jars files required for JOGL application.
- Create a new class in the package. The class must implement GLEventListener interface. For that we have to import the package java.media.opengl. Then we can implement all four methods, init(), reshape(), display() and dispose().
- Create the object of GLProfile class. Use the get() method of GLProfile class and mention proper OpenGL API. GLProfile glp = GLProfile.get ( GLProfile.GL2 );
- Create the GLCapabilities class using constructor and pass GLProfile class object to it. GLCapabilities cap = new GLCapabilities ( glp );
- Create the GLCanvas object using constructor and pass GLCapabilities object to it. GLCanvas canvas = new GLCanvas ( cap );
- The addGLEventListener() method is used to add GLEventListener to the canvas. BasicFrame bf = new BasicFrame(); canvas.addGLEventListener ( bf );
- Size of canvas can be decided using setSize() method. canvas.setSize (400,400);
- Create Swing Jframe object using constructor. Canvas can be added to Jframe. The add() method is used GLCanvas object to Jframe. Using setVisible(true) method we can activate the frame. JFrame jf = new JFrame(“ Simple Swing JFrame ”); jf.add ( canvas ); jf.setVisible ( true );
- In order to view the frame clearly, setSize() method is used with JFrame class object to set its dimensions.
Creating Simple JFrame using GLCanvas in JOGL –
Code:
import javax.media.opengl.GLAutoDrawable; import javax.media.opengl.GLEventListener; import javax.media.opengl.GLProfile; import javax.media.opengl.GLCapabilities; import javax.media.opengl.awt.GLCanvas; import javax.swing.JFrame; public class SimpleFrame implements GLEventListener { Override the init(), display(), reshape() and dispose() methods public static void main(String[] args) { GLProfile glp = GLProfile.get(GLProfile.GL2); GLCapabilities cap = new GLCapabilities(glp); GLCanvas canvas = new GLCanvas(cap); BasicFrame bf = new BasicFrame(); canvas.addGLEventListener(bf); canvas.setSize(400, 400); JFrame frame = new JFrame (" Simple Swing JFrame using JOGL"); frame.getContentPane().add(canvas); frame.setSize(480,480); frame.setVisible(true); } }
Output:
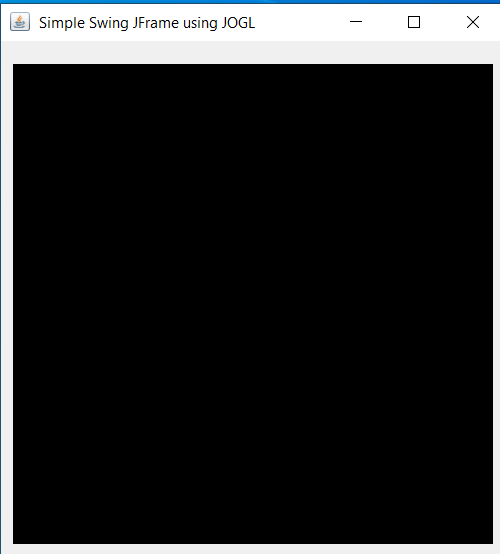
Explanation:
Step 1: Import all the required classes and interfaces such as GLAutoDrawable, GLEventListener, GLProfile, GLCapabilities, GLCanvas and JFrame class in javax.swing
Step 2: Then declare a class which implement GLEventListener interface and override 4 required methods which are init(), display(), reshape(), and dispose().
Step 3: The Object of GLProfile class is created which is passed as an argument to GLCapabilities constructor. The GLCapabilities object is passed as the argument to GLCanvas constructor.
Step 4: BasicFrame object is created and then addEventListener method is called with canvas object.
Step 5: In order to set size of canvas, setSize() method is then called.
Step 6: JFrame constructor is called with title passed in it.
Step 7: frame.getContentPane().add( canvas ) method is used to add our canvas inside the frame.
Step 8: Using frame.setSize() we can set the size of the frame and using setVisible( true ) method, we can make the frame visible to the user.