OpenGL Drawable: GLJPanel
GLJpanel:
GLJPanel class is present in javax.media.opengl.awt package. It is lightweight. It is the subclass of javax.swing.JPanel class. It has OpenGL rendering support. It provides the ability to work with swing components. We will be using JFrame using GLJPanel. It uses hardware-accelerated rendering. To get 100% swing integration this is useful.
GLJpanel Constructors:
- GLJPanel() - GLJPanel component is created with the basic set of OpenGL capabilities that can be used all over the program.
- GLJPanel (GLCapabilities capobj) - It is used to create a GLJPanel with the specified set of OpenGL functionalities.
GLJpanel Methods:
- void addGLEventListener(GLEventListener listener) - The addGLEventListener() method is used to add the GLEventListener object at the end of queue consisting of drawable objects.
- void removeGLEventListener(GLEventListener listener) - This method is used to remove the GLEventListener object from the drawable queue.
- void display() - This method is used for OpenGL rendering.
Steps to create JOGL basic frame using GLJPanel and Swing JFrame -
- Create a new java project give the name to it and click on Finish Button.
- Add jars files required for the JOGL application.
- Create a new class in the package. The class must implement the GLEventListener interface. For that we have to import the package java.media.opengl. Then we can implement all four methods, init(), reshape(), display() and dispose(). We are creating the basic frame. So, we can keep the methods blank.
- Create the object of GLProfile class. Use the get() method of GLProfile class and mention proper OpenGL API. We are using GL2. GLProfile glp = GLProfile.get ( GLProfile.GL2 );
- Create the GLCapabilities class using the constructor and pass GLProfile class object to it. GLCapabilities cap = new GLCapabilities ( glp );
- Create the GLJPanel object using the constructor and pass GLCapabilities object to it. GLJPanel panel = new GLJPanel ( cap );
- The addGLEventListener() method is used to add GLEventListener to the panel. BasicFrame bf = new BasicFrame(); panel.addGLEventListener ( bf );
- Size of panel can be decided using setSize() method panel.setSize (400,400);
- Create Swing JFrame object using the constructor. The panel can be added to frame. The add() method is used to add GLJPanel object to the frame. Using the setVisible(true) method we can activate the frame. JFrame f = new JFrame(“ Simple JFrame ”); f.add ( panel ); f.setVisible ( true );
- In order to view the frame clearly, setSize() method is used with JFrame class object to set its dimensions.
Creating Simple JFrame using GLJPanel in JOGL -
Code:
import javax.media.opengl.GLAutoDrawable; import javax.media.opengl.GLEventListener; import javax.media.opengl.GLProfile; import javax.media.opengl.GLCapabilities; import javax.media.opengl.awt.GLCanvas; import javax.swing.JFrame; public class SimpleFrame implements GLEventListener { @Override public void display(GLAutoDrawable drawobj) { } @Override public void dispose(GLAutoDrawable drawobj) { } @Override public void init(GLAutoDrawable drawobj) { } @Override public void reshape(GLAutoDrawable drawobj, int x, int y, int width, int height) { } public static void main( String[] args ) { GLProfile glp = GLProfile.get ( GLProfile.GL2 ); GLCapabilities cap = new GLCapabilities( glp ); GLJPanel panel = new GLJPanel( cap ); BasicFrame bf = new BasicFrame(); panel.addGLEventListener( bf ); panel.setSize(400, 400); JFrame frame = new JFrame (" Simple JFrame using GLJPanel "); frame.getContentPane().add( panel ); frame.setSize( 480,480 ); frame.setVisible( true ); } }
Output:
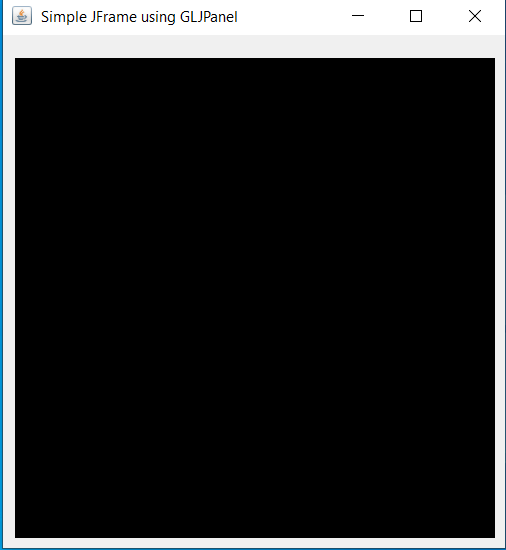
Explanation:
For constructing the basic frame using GLJPanel we have to import all the required classes and interfaces in packages such as GLAutoDrawable, GLEventListener, GLProfile, GLCapabilities, GLCanvas. JFrame class in javax.swing is also imported to use swing lightweight components.
We have to declare a class and implement GLEventListener interface to override 4 required methods that is init(), display(), reshape(), and dispose().
Then we have to create a object of GLProfile class which is passed as an argument to GLCapabilities constructor. The GLCapabilities object is passed as the argument to GLJPanel constructor.
BasicFrame object is created and then addEventListener method is called with panel object.
setSize() method is then called with panel object to set size of panel.
Then JFrame constructor is called with title passed in it.
The panel can be added to the frame. The add() method is used to add GLJPanel object to the frame. frame.getContentPane().add( panel ) is used to add our panel inside the frame.
Using frame.setSize() we can set the size of the frame and using setVisible( true ) method, we can make the frame visible to the user.