PouchDB Add Attachment
As the names suggest, attachments are attached to the document. To add a binary object in the document in the PouchDB, we use putAttachment() method. This method needs the parameters: document id, MIME type with the attachment, and attachment id. We can also pass an optional callback function.
Syntax is as below:
1. db.putAttachment( document_id, attachment_Id, attachment_to_add, type, [callback] );
To create the attachment with the documents, we use the object of Nuffer or Blob. And call the function named Buffer. from(). We use the object of Blob when we work along with the browser, and for Node.js, we use Buffer.
2. db.putAttachment( document_id, attachment_Id, rev, attachment_to_add, type, [callback] );
If the document already exists, then we need to pass _rev in the method. If the document is not present, then it will create an empty document and add an attachment.
Lets see and example we will use Node js that’s why we will be using Buffer
In the example we have created a new database named New_Db and attaching the newly created attachment. It will create an empty document and add attachment to it.
package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('New_Db');
//Preparing the attachment
var my_attachment = new Buffer.from(['Hello PouchDB......'], {type:'text/plain'});
//Adding attachment to the document
db.putAttachment('001', 'attachment_new.txt', my_attachment, 'text/plain', function(err, res) {
if (err) {
return console.log(err);
} else {
console.log(res+ "Attachment added !!")
}
});
Store the above code snippet into the file, and name it as addattach.js into the folder named "PouchDB_examples".
Next, open the command prompt and run the file and run the following command.
C:\Users\ACER\Documents\PouchDB_Examples>node addattach.js
Output:
[object Object]Attachment added !!

Verification
We can verify if the attachment is added successfully or not We need to execute the following code and check the output.
package we need, by using require method
package
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('New_Db');
//Reading the Document
db.get('001',{attachments: true}, function(err, doc) {
if (err) {
return console.log(err);
} else {
console.log(doc);
}
});
Store the above code snippet into the file, and name it as verifyattachment.js into the folder named "PouchDB_examples".
Next, open the command prompt and run the file and run the following command.
C:\Users\ACER\Documents\PouchDB_Examples>node verifyattachment.js
Output:
{
_attachments: {
'attachment_new.txt': {
content_type: 'text/plain',
revpos: 1,
digest: 'md5-k7iFrf4NoInN9jSQT9WfcQ==',
data: 'AA=='
}
},
_id: '001',
_rev: '1-c0bfd1fcd0cdf4ac83806411fc4d831f'
}
Lets see an example with the existing document, We will add a attachment to the existing document and pass the _rev in the putAttachemnt() method.
package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('New_Db');
//Adding attachment to existing document
var my_attachment1 = new Buffer.from (['Hello PouchDB database......'], {type: 'text/plain'});
rev = '1-c0bfd1fcd0cdf4ac83806411fc4d831f';
db.putAttachment('001', 'attachment_new.txt', rev, my_attachment1, 'text/plain', function(err, res) {
if (err) {
return console.log(err);
} else {
console.log (res + "Attachment added!!")
}
});
Store the above code snippet into the file, and name it as addattach_exist.js into the folder named "PouchDB_examples".
Next, open the command prompt and run the file and run the following command.
C:\Users\ACER\Documents\PouchDB_Examples>node addattach_exist.js
Following sentence will be displayed:
[object Object]Attachment added!!

To verify we can execute following code and check:
package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('New_Db');
//Reading the contents of a Document
db.get('001', function(err, doc) {
if (err) {
return console.log(err);
} else {
console.log(doc);
}
});
It will return the output:
{
_attachments: {
'attachment_new.txt': {
content_type: 'text/plain',
revpos: 2,
digest: 'md5-k7iFrf4NoInN9jSQT9WfcQ==',
length: 1,
stub: true
}
},
_id: '001',
_rev: '2-ea1d82ecaf130399c9154bc4c3f9a737'
}
Add Attachment to a Remote Database
PouchDB allows us to add an attachment on the remotely stored Database. To perform this, we don't need to pass the database name; instead, we need to pass the Database path where we wish to add an attachment to the document.
Let's see an example:
We are attaching to the document in the remote Database named remote_db.
Which exists already and looks like follows:
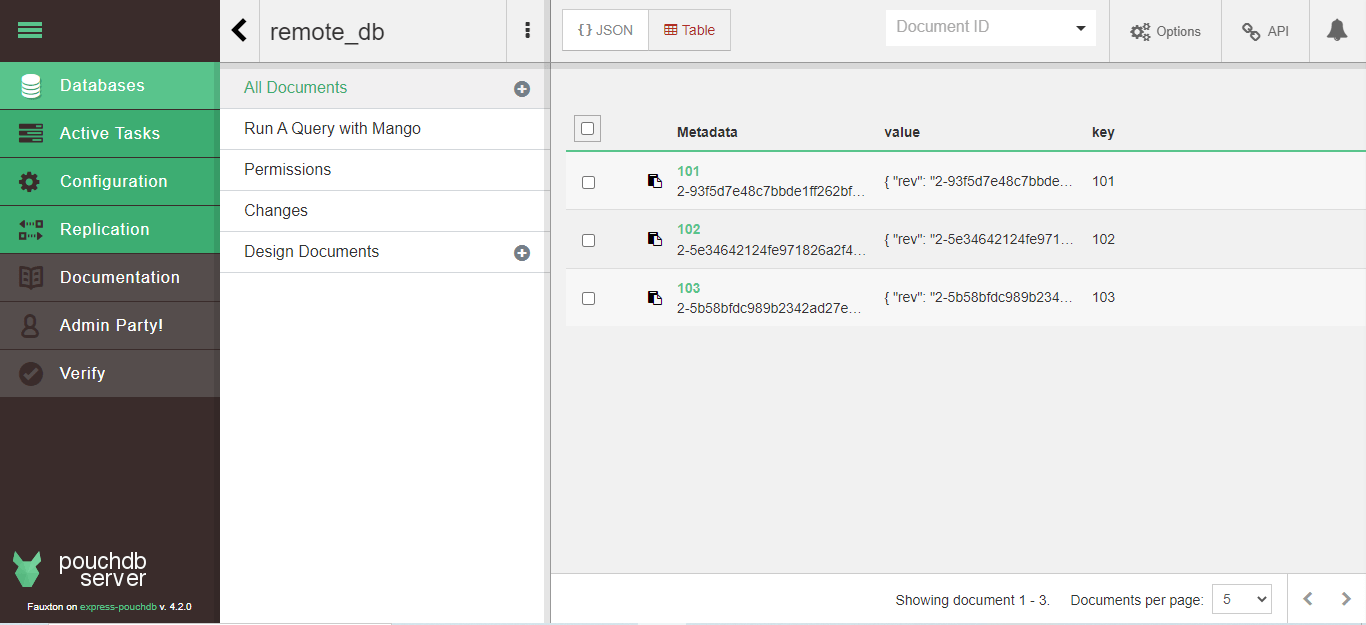
package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('http://localhost:5000/remote_db');
//Adding attachment to existing document
var my_attachment = new Buffer.from (['Hello PouchDB remote DB...'], {type: 'text/plain'});
rev = "2-93f5d7e48c7bbde1ff262bf146894fef";
db.putAttachment('101', 'attachment_1.txt',rev, my_attachment, 'text/plain', function(err, res) {
if (err) {
return console.log(err);
} else {
console.log (res+ "Attachment remotely added!!")
}
});
Store the above code snippet into the file, and name it remote_createBatch.js into the folder named "PouchDB_examples".
Next, open the command prompt and run the file and run the following command.
C:\Users\ACER\Documents\PouchDB_Examples>node addattach_remote.js
Output:
[object Object]Attachment remotely added!!

Verification
We can verify it by going to the URL http://127.0.0.1:5000/_utils/
This will show the following, where we can see the Database named "remote_db.

We can see attachment is added.