PouchDB creating and reading document
In PouchDB, we can create a document using the db. put() method.
The syntax is as follows.
We can store the Document we wish to create into the variable and pass it as a parameter in the method. Additionally, in the put method, we can also pass a callback function as a parameter; it is optional.
db.put (doucment_var, callback)
Lets see an example:
The document we are creating is in JSON format which is a set of key-value pairs which are separated by comma and are nested in curly braces.
// package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('Second_Database');
//Preparing the document
doc = {
_id : '101',
name: 'Anuj',
age : 25,
designation : 'Developer'
}
//adding Document
db.put(doc, function(err, response) {
if (err) {
return console.log(err);
} else {
console.log("Document created Successfully");
}
});
Store the above code snippet in the file named creating_doc.js.
Open the command prompt and execute the above JS file in node as follows:
C:\Users\ACER\Documents\PouchDB_Examples>node create_doc.js
This command creates the provided Document into the PouchDB database, named "Second_Database," and resides locally. And the below message is shown on the console.
Document created Successfully
Output:

Let's see one more example, which will be created in the database named my_new_database with the content mentioned in the file as follows:
// package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('my_new_database');
//Preparing the document
doc = {
_id : '001',
product_name: 'Refrigerator',
product_modal : MHK121,
Manufacturer : 'Sone'
}
//Adding Document
db.put(doc, function(err, response) {
if (err) {
return console.log(err);
} else {
console.log("Document created Successfully");
}
});
Open the command prompt and execute the above JS file in node as follows:
C:\Users\ACER\Documents\PouchDB_Examples>node create_docc.js
Uploading a document to a remote database:
PouchDB allows us to insert a document into the database, which is stored remotely on the server.
To perform this, we have to pass the database path we wish to create documents in instead of the database name.
Let's see an example:
In the following example, we are creating a Document in the database named employees. We can verify the databases present using the URL http://127.0.0.1: 5000/_utils/
// package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('http://localhost:5000/employees');
//Preparing the document
doc = {
_id : '001',
name: 'Ajeet',
age : 28,
designation : 'Developer'
}
//adding Document
db.put(doc, function(err, response) {
if (err) {
return console.log(err);
} else {
console.log("Document created Successfully");
}
});
Store the above code snippet in the file named remote_doc.js.
Open the command prompt and execute the above JS file in node as follows:
C:\Users\ACER\Documents\PouchDB_Examples>node remote_doc.js
The above line will create a document in the PouchDB database named employees and show the following message.
Document created Successfully
Output:

Verification
We can recheck the document creation by visiting the URL http://127.0.0.1: 5000/_utils/ and creating the "employees" database.

Read the Document created
By the use of db.get(), we can read or retrieve the Document on PouchDB.
Following is the syntax to use the method:
db.get(document, callback)
we can send the document id and callback function to the method as a parameter where the callback function is optional
Let's see an example:
In the following example we will be reading the contents of the document by using get() method
// package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('Second_Database');
//Reading the data of a Document
db.get('101', function(err, doc) {
if (err) {
return console.log(err);
} else {
console.log(doc);
}
});
Store the above code snippet in the file named readdoc.js.
Open the command prompt and execute the above JS file in node as follows:
C:\Users\ACER\Documents\PouchDB_Examples>node readdoc.js
It will read the contents from the Document which is present in the second_database database store locally. The following information will be shown on the console
{
name: 'Anuj',
age: 25,
designation: 'Developer',
_id: '101',
_rev: '1-6e663abd96c11b07742a36f9494043d4'
}

Reading a Document from a Remote Database
We can also read the document form the database, which is stored remotely on the server.
To perform this, we have to pass the path of the database we wish to read documents from.
Let's see an example where there is a database named employees remotely. We can verify it by accessing the following URL the URL http://127.0.0.1: 5000/_utils/
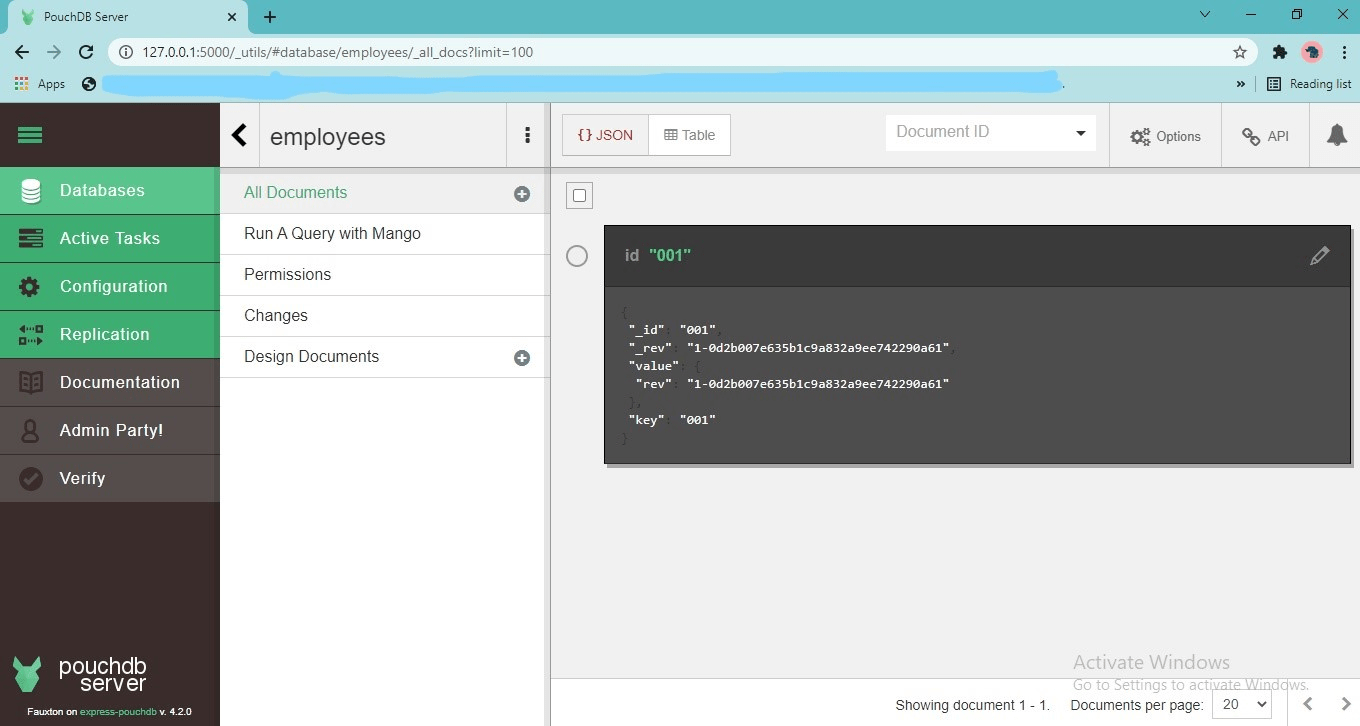
We can observer the database has the document with the id 001.
So while writing the code we have to mention and check for the id “001” in the database named employees and return the contents of it
// package we need, by using require method
var PouchDB = require('PouchDB');
//Creating the object of database
var db = new PouchDB('http://localhost:5000/employees');
//Reading the contents of a document
db.get('001', function(err, doc) {
if (err) {
return console.log(err);
} else {
console.log(doc);
}
});
Store the above code snippet in the file named remote_read.js.
Open the command prompt and execute the above JS file in node as follows:
C:\Users\ACER\Documents\PouchDB_Examples>node remote_read.js
This reads the contents of the given Document that exists in the database named employees, which is stored in CouchDB.
The following information will be shown on the console.
{
name: 'Ajeet',
age: 28,
designation: 'Developer',
_id: '001',
_rev: '1-0d2b007e635b1c9a832a9ee742290a61'
}
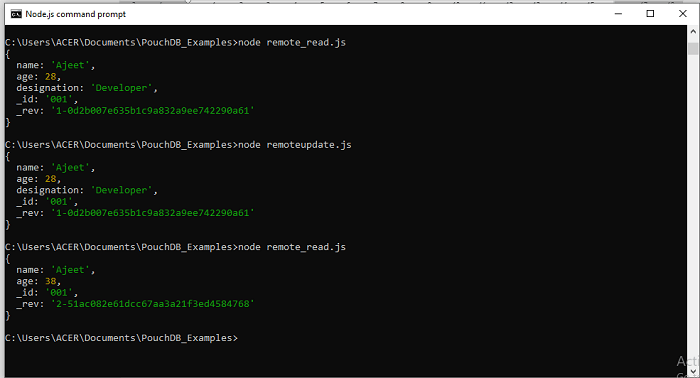