Class in ES6
Class in ES6: The Class is a basic term in object-oriented programming (OOPs). A class helps to determine the prototype for modeling in real-world objects. It is used to formulate the program code into logical parts and make it reusable.
The definition of a class only contains the functions and constructors.
A class consists of the constructor used to allot the memory blocks to the class objects.
A class also involves the function used to perform the operations to the objects.
Syntax of class expression
var variable_name = new Class_name{ }
Class Declaration
The class declaration is referred to declare the class name with class attributes such as the parent class of the class. The declaration process must contain the class keyword.
Syntax of class declaration
class Class_name { }
Here, we have an example to understand the class expression and class declaration.
Example 1
var Employee = class // Class Expression { constructor (name, age) { this.name = name; this.age = age; } }
Example 2
class Employee // Class Declaration { constructor (name, age) { this.name = name; this.age = age; } }
Important Point: The class body contains the methods instead of data properties.
Object creation from the class
We can easily create or install an object from a class with the help of new keyword.
Syntax
var object_name =new class_nam([arguments])
Example
var emp = new employee (‘Jackson’, 25)
Function Accessing
We can access the functions and attributes of a class with the help of an object. The notation dot (‘.’) is used to access the class data members.
Syntax
Object function_name();
Example
Here, we have an example to illustrate the concept of function accessing.
‘use strict’ class Employee { constructor (name, age) { this.name = name; this.age = age; } } Emp() { console.log (“The Employee name is: ”, this.name) console.log (“The age of Employee is: ”, this.age) } var EmpObj = new Employee (‘Robin’, 23); EmpObj.Emp;
Output
After the execution of the above code, we got the following output:
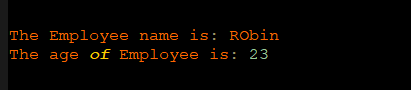
Important Point: The constructor definition is necessary for the class because, by default, every class contains constructor.
The Static Keyword
The Static keyword is used to create a static function inside the class. The static functions are always declared with the name of the class.
Example
‘use strict’ class Sample { static show () { Console.log (“Static Function”); } } Sample.show() // call the static method
Output
After the execution of the above example, we got the following output:

The Class Inheritance
ES6 introduced the class inheritance using super and extends keywords. Before the ECMAScript 5, the execution of class inheritance took various steps. The ES6 clarifies the concept of class inheritance.
The term inheritance can be defined as an object’s ability to access the methods and other properties of other objects. In JavaScript, the inheritance works by using prototypes. The particular form of inheritance is known as prototypal inheritance. The class, we extended to create another class is called Parent/Superclass and the newly created class is called Child/Sub class.
We can easily inherit a class from another class with the help of extends keyword. The child class can easily inherit the properties from the parent class except the constructor.
Syntax
class sub_class_name extendes super_class_name { }
We can easily inherit a class from another class with the help of extends keyword.
Example
‘use strict’ class Employee { constructor (p) { this.name = p; } } class User extends Employee { show() { console.log (“The name of employee is:” +this.name) } } var object = new User(‘Robinson); object.show ()
Output
After the execution of the code, we got the following output:
