Loops in ES6 | ECMAScript 6
Loops in ECMAScript 6
A Loop statement can be defined as a group of instructions that are always repeated. In the computer programming language, the loops help us to execute a collection of instructions that repeats again and again till the condition is true. In the loop statement, the repetition of a statement is defined as an iteration.
Classification of Loops
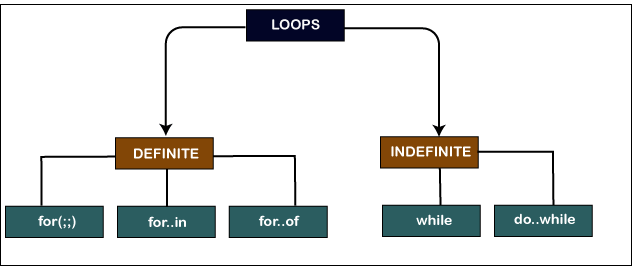
Now, we are going to discuss each category of the loop in detail.
Definite Loops
This type of loop has a definite/ fixed/ constant number of iterations. There are the following three types of definite loops used in ECMAScript 6.
Loop | Description |
For (; ;) loop | The for loop is used to execute the block code for a particular number of times within a termination condition. |
For…in loop | The for…in loop is used to execute the block code by using the properties of an object. |
For…of loop | The for…of loop is used to execute the iterables (string, array) instead of object literals. |
Now, we are going to discuss each loop with syntax and example.
The For (; ; ) loop
The for loop helps us to execute the block code for a particular number of times. It means if we have a constant number of iterations, then we will use a for loop.
Syntax
For (initialization; conditions; increment/ decrement) { // code statement to be executed }
The for (; ;) loop contains the following elements-
Initialization: It is a process to initialize the variables and also used for existing initialized variables. Initialization is optional to use.
Condition: It always executes to check the loop condition. It always returns True/False (Boolean value). It executes until the loop condition is false. In ECMAScript, it is optional to use.
Increment/ Decrement: It is used to increase or decrease the value of a variable by 1(+1 /-1). It is also optional to use.
Flowchart of for (; ;) loop
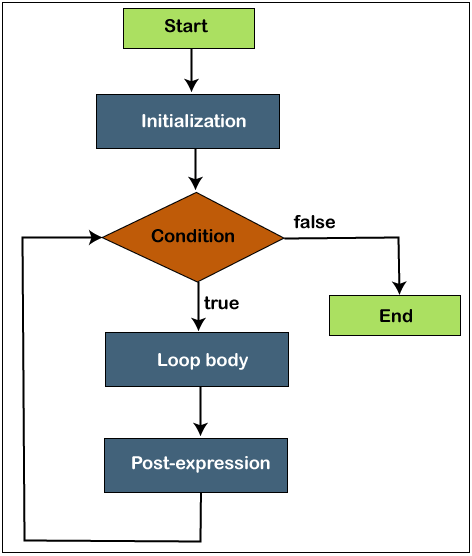
There are some examples given below, which represents the various kind of for loop.
Example 1
Here is an example of a simple for loop.
Var a; For (a=1; a<=10; a++) { Console.log (“4 x ” + a + “ = ”, 4 * a ); }
Output

Here, we will try to illustrate the code of for loop with multiple expressions.
Example 2
The below-given code helps us to understand the concept of for loop with multiple expressions.
“use strict” // Directive for Strict mode For (let variable, p = 0, q = 1; q<100; variable = p, p = q, q = p + variable) { Console.log (q); }
Output
After the execution of the above code, we will get the following output

In the program code, we can easily merge the multiple expressions and assignments within a single loop by using comma (,).
Example 3
Here is an example of infinite for loop.
for (; ;) { Console.log (“Infinite loop”); // this loop will run infinite time until we use break keyword }
Output
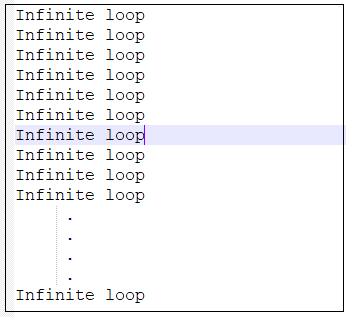
If we want to terminate this infinite loop then we will use Ctrl + C.
The For…in Loop
The for…in loop is similar to for loop, but it iterates the block code by using the properties of an object (The object keys). We can use a for…in loop when we are dealing with objects where the index order is not necessary.
In each iteration of the for…in loop, one property is allotted to the variable name. The loop executes the condition until the object have at least one property left. The for…in loop comes under the category of definite loops.
Syntax
for (variable_name in object) // in is used as a keyword { // Block code to be executed }
Example
var employee = {“Emp_name” : “Daniel”, “Emp_id”: “D01”, “Emp_age” : 30}: for (var props in employee) { Console.log (props+ “ : ” + employee [props]); }
Output
After execution of the code, we will get the following output.
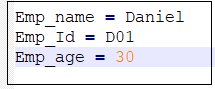
If we pass a function as the properties of the object then we will get the whole function as the output. We will try to implement it with the following example.
function car = (model_no) { this. model =model_no; this. color = “Black”; this. transmission = “Manual”; this. price = function price () // This function will return as the output { Console.log (this. model + “price =500000”); } } var Toyota = new car (“Fortuner”); for (var props in Toyota) { Console.log (props+ “ : ” + Toyota[props]); }
Output
After execution of the code, we will get the following output.

In the code mentioned above, the function price () is returned as the output.
The For…of loop
The for…of loop is used to execute the iterables (array, string) instead of object literals.
In each iteration of for…of loop, one property is allotted to the variable name. The loop executes the condition until the object has at least one iteration left. The for…of loop comes under the category of definite loops.
Syntax
for (variable_name of the object) // of is used as a keyword { // Block code to be executed }
Example
Var mobile = [‘Samsung’, ‘Micromax’, ‘Redmi’, ‘Nokia’]; for (let value of mobile) { console.log (value); }
Output
After the execution of the above example, we will get the following output.
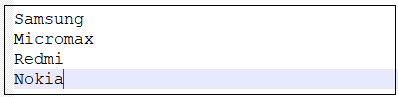
Indefinite Loop
The Indefinite loop has an unknown or transitional number of iterations inside the loop. In the Indefinite loop, we have an infinite number of iterations. There are following two types of indefinite loops listed below:
Loop | Description |
While loop | This loop is used to execute the condition until a particular condition is true. The condition classifies before the code statement is executed. |
Do…while loop | It is similar to a while loop, but the difference is; the do…while loop executes the code statement at one time without checking the condition. |
Now, we are going to discuss each loop with syntax and example.
While Loop
The while loop is considered as a control statement. It is used to execute the program code in a repeated way based on the given Boolean condition. This loop is also known as “the pre-test loop.” In the while loop, we can check the condition before the execution of the block code.
The while loop contains; condition/ expression and block of code. The while loop comes under the category of Indefinite loop.
Syntax
while (condition/ expression) { // Code or expression to be executed }
Flowchart of While loop

Example
Here, we discuss the example of while loop.
var r = 0; while (r < 10) { console.log (r); r++; }
Output
After the successful execution of the example, we will get the following output.

Note-
- When the condition is true, then the loop will be executed.
- The condition/ expression is necessary to execute the loop.
- If the condition is true, then the loop always executes, but if the condition is false, then the loop will terminate.
Do…while Loop
The Do…while loop is a control statement. It is used to execute the code block at least once before condition check; if the condition is true, then the loop executes continuously; otherwise, the loop will be terminated. The Do…while loop is also known as “the post-test loop”. This loop comes under the category of Indefinite loop.
Syntax
do { // code block to be executed; } while (condition/ expression);
Flowchart of Do…while loop
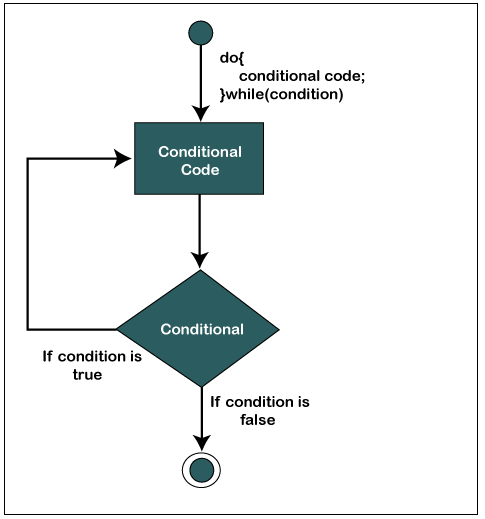
Example
Here, we discuss an example of Do…while loop.
let result = “”; let a = 0; do { a = a + 1; result = result + a; } while (a < 10); console.log (result);
Output
After the execution of the above code, we will get the following output.
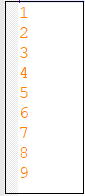
Difference between While and Do…while loop
While Loop | Do…while Loop |
It checks the conditions before the execution. | It checks condition after the execution. |
It executes the code block if and only if the condition is true. | It executes the code block at least once; even the condition is false later. |
It is defined as an entry-controlled loop. | It is defined as an exit-controlled loop. |
We can use it when the condition is important. | We can use it when the process is more important. |
The semicolon (;) is not compulsory after the end of the while loop. | The semicolon (;) is compulsory after the end of the do…while loop. |
Syntax while (condition) { // statement } | Syntax do { // statement } while (condition) |