Map Object in ES 6
Map Object in ES 6
ES 6 introduced a new feature that is included in JavaScript. In the previous versions of ECMAScript, if we want to perform mapping on values and keys, we mostly use the objects. The object allows us to map any values and keys.
In ES 6, it is an additional feature to work with a new set of types known as a map. It is used to store the key pair, in which we can use any kind of key or values.
The map object recalls the keys insertion order. The value and keys in the map can be treated as an object or primitive. The map object often used to return an empty or new map. The maps are defined as an ordered form so that they can traverse the elements.
Syntax
Here, we have the syntax to create a map.
var map = new map([iterables]); // map() accepts iterable objects
Methods of Maps
The term map object contains various methods. They are given below in the tabular form-
Sr. No | Method Name | Description |
1 | Map.prototype.delete(key) | This method is used for deleting the entries. |
2 | Map.prototype.clear() | This method is mostly used to delete value pairs and keys from the map object. |
3 | Map.protoype.entries() | This method is used to return the iterator object, which contains an array with key values pairs. The elements in Map are always in the insertion order. |
4 | Map.prototype.has(value) | It is used to check that the corresponding keys present in the Map object or not. |
5 | Map.prototype.keys() | This method is used to return an iterator for all keys involved in the Map object. |
6 | Map.prototype.values() | This method is used to return an iterator for all the values stored in the map. |
7 | Map.prototype.forEach(callbackFn[ thisArgs]) | This method is used to execute the callback function at single time. The function callback executes for each value of a map object. |
Map Properties
Map.prototype.size
This property is used to return the various values in the Map object.
Syntax
Map.size
Example
var map = new Map (); map.set (‘David’, ‘Writer’); map.set (‘Harris’, ‘Director’); map.set (‘Bob’, ‘Actor’); map.set (‘Willson’, ‘Designer’); console.log (map.size);
Output
After the execution of the code, we got the following output:
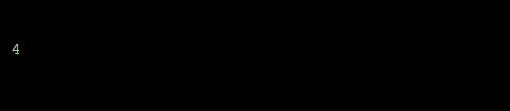
Weak Maps
In ES 6, the weak maps are the same as regular maps. But, the key difference is that the keys are considered as the object in weak maps. It means the map key is treated as an object, and the value is arbitrary value. The weak maps are used to store the elements in the form of key-value pairs with the weak referenced keys.
The object in the weak map always has an object type key. We don’t have any method to find the list of keys, so the keys are not treated as enumerable. The objects iterate the elements in an insertion manner in a weak map. If the key objects are without the reference, they will move into the garbage collection. The weak map object includes get(key), set(value, key), has(key), and delete (key).
Here, we have an example to understand this concept.
Example
‘use strict’ let abc = new Weakmap(); let object = {}; console.log (abc.set(objact, “Hello ES6”)); console.log (abc.has(object));
Output
After the execution of the code, we got the following output:

Iterator and Map
The iterator is also called an object. It is used to determine the manner and returns result during the termination. We can access only a single set of object at a time. The Set and Map are used to return the iterators as output. The iterator object is associated with the next method (). If we call the next method (), it returns an object with value and done properties.
Here, we have some examples to understand the iterator associated with the map object.
Example 1
‘use strict’ var rainbow = new Map ([ [‘1’, ‘Blue’], [‘2’, ‘Orange’], [‘3’, ‘Purple’], [‘4’, ‘Black’] ]); var iterator = rainbow.values(); console.log (iterator.next()); console.log (iterator.next()); console.log (iterator.next());
Output
After the execution of the above code, we got the following output:

Example 2
‘use strict’ var rainbow = new Map ([ [‘1’, ‘Blue’], [‘2’, ‘Orange’], [‘3’, ‘Purple’], [‘4’, ‘Black’] ]); var iterator = rainbow.entries(); console.log (iterator.next()); console.log (iterator.next()); console.log (iterator.next());
Output
After the execution of the code, we got the following output:

Example 3
‘use strict’ var rainbow = new Map ([ [‘1’, ‘Blue’], [‘2’, ‘Orange’], [‘3’, ‘Purple’], [‘4’, ‘Black’] ]); var iterator = rainbow.keys(); console.log (iterator.next()); console.log (iterator.next()); console.log (iterator.next());
Output
After the execution of the code, we got the following output:
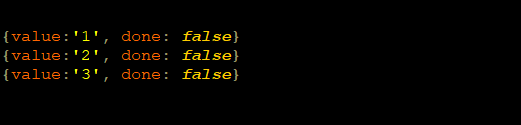
Weak Maps with for…of Loop
In the Weak Maps, for…of loops are used to perform the iterations on the values and keys of Map object.
We have an example to understand the weak map with a for…of loop.
Example
‘use strict’ var rainbow = new Map ([ [‘1’, ‘Blue’], [‘2’, ‘Orange’], [‘3’, ‘Purple’], [‘4’, ‘Black’] ]); for (let rain of rainbow.values()) { console.log (rain); } console.log (“ ”) for (let rain of rainbow.entries()) console.log (‘${rain[0]}: ${rain[1]}’);
Output
After the execution of the above example, we got the following output:
