Hibernate first Example
Now we are going to create the first example in Hibernate. We will proceed step by step to develop the first Java application in Hibernate. The steps are listed below:-
- Create the POJO /Persistent/Bean class
The encapsulation of many objects into a single object is done by POJO class or Bean class.
All the variables of bean class are set as private with public setter and getter methods, and it must contain a no-arg constructor.
Let us create Bean class- Student.java
public class Student { private int rollno; private String name,course; public int getRollno() { return rollno; } public void setRollno(int rollno) { this.rollno = rollno; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getCourse() { return course; } public void setCourse(String course) { this.course = course; } }
2. Create a mapping file for Hibernate bean class
The format of the mapping class should be <classname>.hbm.xml file. For example, if our POJO class name is Student then the name of mapping class should be Student.hbm.xml. Hibernate mapping file contains many elements such as <hibernate-mapping></hibernate-mapping>, <class></class>, <id></id>, <generator></generator>, <property></property> and many more.
Let us create a Student.hbm.xml mapping file.
http://hibernate.sourceforge.net/hibernate-mapping-5.3.dtd">
3. Create a Configuration file
The name of the configuration file should always be hibernate.cfg.xml. It contains information about the mapping file and relational database.
Let us create a Configuration file named hibernate.cfg.xml
update org.hibernate.dialect.MySQL5Dialect com.mysql.jdbc.Driver jdbc:mysql://localhost:3306 /test2 root root
4. Create the Application class
It is a class which contains the main() methodused to run the application.
Let’s create Application.java.
import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class App { public static void main( String[] args ) { Configuration cfg= new Configuration(); cfg.configure("hibernate.cfg.xml"); SessionFactory fact = cfg.buildSessionFactory(); Session session = fact.openSession(); session.beginTransaction(); Student student= new Student(); student.setRollno(111); student.setName("jyotika"); student.setCourse("BCA"); session.save(student); session.getTransaction().commit(); System.out.println("saved successfully "); session.close(); } }
Output

Database Table- student01
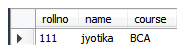