Hibernate Many-to-One Mapping
Many-to-One Hibernate Mapping with Example
A Many-to-One association mapping is the reverse of One-to-Many association mapping. For example, many (customers) are associated with one (vendor). In Hibernate, Many-to-One association mapping is applied from child class object to parent class object. It is an N to 1 relationship.
For example, there are many customers of a single vendor; it is just the reverse of one-to-many association mapping. Here, we need to take a collection property like Set, Map, Bag, and List in the Customer.java class.
Now, we are going to create an example, in which there are two persistent classes, i.e., Customers and Vendor. Here, we are taking a unidirectional mapping, so we need to define @ManyToOne and @JoinColumn annotations in the Customer class.
Following are the steps to create the example:
- Create all persistent classes
In this step, we are going to develop persistent classes, i.e., Vendor.java and Customer.java.
Vendor.java
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name="vend") public class Vendor { @Id @Column(name="vend_id") private int v_id; @Column(name="v_name") private String name; public int getV_id() { return v_id; } public void setV_id(int v_id) { this.v_id = v_id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Customer.java
import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.ManyToOne; import javax.persistence.Table; @Entity @Table(name="custo") public class Customer { @Id @Column(name="cust_id") private int c_id; @Column(name="cust_name") private String name; @ManyToOne(cascade=CascadeType.ALL) @JoinColumn(name="v_id",referencedColumnName="vend_id") private Vendor v; public int getC_id() { return c_id; } public void setC_id(int c_id) { this.c_id = c_id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Vendor getV() { return v; } public void setV(Vendor v) { this.v = v; } }
- Create the configuration file
In this step, we are going to create the configuration class (hibernate.cfg.xml) which contains the information of persistent class and the database.
hibernate.cfg.xml
update org.hibernate.dialect.MySQL5Dialect com.mysql.jdbc.Driver jdbc:mysql://localhost:3306/test root root
- Create the main class that stores the object of the persistent class
In this step, we are going to create a class which consists of main() method, and stores the objects of persistent classes.
App.java
import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class App { public static void main( String[] args ) { Configuration cfg= new Configuration(); cfg.configure("hibernate.cfg.xml"); SessionFactory factory= cfg.buildSessionFactory(); Session session= factory.openSession(); Vendor ven= new Vendor(); ven.setV_id(101); ven.setName("jahanvi"); Customer c= new Customer(); c.setC_id(201); c.setName("Nihal"); c.setV(ven); Customer c2 =new Customer(); c2.setC_id(202); c2.setName("Rajak"); c2.setV(ven); Transaction t= session.beginTransaction(); session.save(c); session.save(c2); t.commit(); session.close(); System.out.println("saved successfuly!!"); factory.close(); } }
- Output
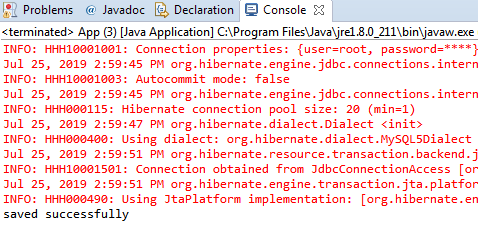
- Table
Custo table
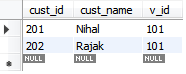
Vend table
