Hibernate Named Query using Annotation
In annotation-based named query, we use @NamedQuery annotation inside the POJO class.
@NamedQuery- It is used to describe a single named query. It consists of four attributes- two of which are compulsory, and two are optional. The two required attributes are name and query which defines the query name and the query string itself. The two optional attributes are lockMode and hints which provide the replacement for setLockMode() and setHint() methods.
Example of Named Query using annotation
Let us assume a table (students) containing three columns (Sid, S_name, and S_rno) are present in the database. There exist some records in the database table (students).
In this example, we are going to fetch an existing record using Named query. Following are the steps to create an example of a Named query using annotation.
- Add dependencies
For this example, you need to add some dependencies in pom.xml.
org.hibernate hibernate-core 5.4.0.Final mysql mysql-connector-java 8.0.16
- Create a POJO class
In this step, we are going to create a POJO class, i.e., Student.java.
Student.java
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; import org.hibernate.annotations.NamedQuery; @Entity @NamedQuery(name= "Student.byId", query="from Student where Sid=?0") @Table(name="students") public class Student { @Id @GeneratedValue(strategy=GenerationType.AUTO) @Column(name="Sid") private int id; @Column(name="S_name") private String name; @Column(name="S_rno") private int rollno; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getRollno() { return rollno; } public void setRollno(int rollno) { this.rollno = rollno; } }
- Create the configuration file.
The configuration file contains the information of mapping classes and database.
hibernate.cfg.xml
org.hibernate.dialect. MySQL5Dialect com.mysql.jdbc. Driver jdbc:mysql:// localhost:3306/test2 root root update
- Create the main class that stores the object of POJO class
In this step, we are going to create a main class (which contains the main method) that stores the object of the POJO class.
App.java
import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class App { public static void main( String[] args ) { Configuration cfg= new Configuration(); cfg.configure("hibernate.cfg.xml"); SessionFactory factory= cfg.buildSessionFactory(); Session session=factory.openSession(); session.beginTransaction(); Query query=session.getNamedQuery("Student.byId"); query.setInteger(0, 2); Liststd= (List ) query.list(); session.getTransaction().commit(); session.close(); for(Student student : std) { System.out.println(student.getName()); } System.out.println("successfull"); } }
OUTPUT

Database table
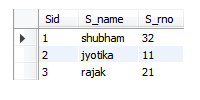