Hibernate One-to-One Mapping Example
One-to-One Hibernate Mapping
In One-to-One association mapping, one object of a persistent class is related to one object of another persistent class. Here, we are going to create an example of one-to-one mapping using annotation. In this case, we are using bidirectional mapping, and no foreign key will be created in the primary table.
In this example, we will take two persistent classes, i.e., Student and Address. We are using bidirectional mapping, so that, one student can have one address and vice-versa. Here, we need to define @OneToOne annotation in the persistent classes.
Following are the steps to create the example:
- Create all POJO classes
In this step, we are going to create persistent classes, i.e., Student.java and Address.java. Both the classes contain the reference of each other.
Student.java
import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.OneToOne; import javax.persistence.Table; @Entity @Table(name="students") public class Student { @Id @Column(name="sid") private int id; @Column(name="sname") private String name; @Column(name="crs") private String course; @OneToOne(targetEntity=Address.class,cascade=CascadeType.ALL) private Address address; public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getCourse() { return course; } public void setCourse(String course) { this.course = course; } }
Address.java
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.OneToOne; import javax.persistence.Table; @Entity @Table(name="address") public class Address { @Id @Column(name="Aid") private int addressid; @Column(name="add") private String address; @Column(name="state") private String state; @Column(name="pin") private int pincode; @OneToOne(targetEntity=Student.class) private Student student; public int getAddressid() { return addressid; } public void setAddressid(int addressid) { this.addressid = addressid; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getState() { return state; } public void setState(String state) { this.state = state; } public int getPincode() { return pincode; } public void setPincode(int pincode) { this.pincode = pincode; } public Student getStudent() { return student; } public void setStudent(Student student) { this.student = student; } }
- Create the configuration class
In this step, we are going to create the configuration class (hibernate.cfg.xml) which contains the information of persistent class and the database.
hibernate.cfg.xml
update org.hibernate.dialect.MySQL5Dialect com.mysql.jdbc.Driver jdbc:mysql://localhost:3306/hibernate root root
- Create the main class which stores the object of the persistent class
In this step, we are going to create a class which consists of main() method, and stores the objects of persistent classes.
App.java
import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class App { public static void main( String[] args ) { Configuration cfg= new Configuration().configure("hibernate.cfg.xml"); SessionFactory factory= cfg.buildSessionFactory(); Session session= factory.openSession(); Transaction t= session.beginTransaction(); Student s= new Student(); s.setName("Jyotika Sharma"); s.setCourse("BCA"); Address a= new Address(); a.setAddress("E-50 chhatarpur extn"); a.setState("New Delhi"); a.setPincode(110074); s.setAddress(a); a.setStudent(s); session.persist(s); t.commit(); session.close(); System.out.println("saved successfully"); } }
OUTPUT
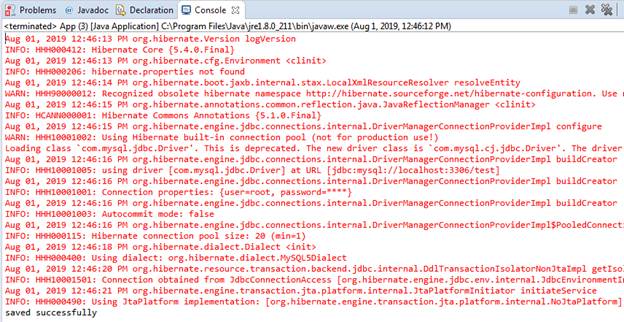
TABLE- students
