Spring MVC Tutorial
Spring MVC is a web Model-View-Controller framework built on Servlet API. It is used to build web applications. The MVC framework works around central servlet called DispatcherServlet. It dispatches the requests to the controllers and offers other functionalities used to develop web-applications. The main purpose of MVC is to separate the different aspects of the application into various logics (Input, Business, and UI Logic), besides providing loose-coupling between the objects.
Model (M) – The Model contains the data or information of the application. It is responsible for managing the application’s data, business logic, and business rules. The Model is used for shipping data between various parts of Spring MVC in the application.
View (V) – The View is used for displaying information or data in the form of text or graph to the user. In Spring, the most common view template we use is JSP (JavaServer Pages) + JSTL (Java Standard Tag Library). The JSP page is used to read the model data and then display them to the user.
Controller (C) – The Controller is the code that is created by the developer. It contains the business logic of the application. Also, it handles the web request, stores the data into the database, and retrieves data from a web service/ database.
Front Controller – The DispatcherServlet acts as a front controller in the Spring MVC framework. It is also responsible for the workflow of the Spring MVC.

DispatcherServlet
The DispatcherServlet is a central dispatcher that is used to handle an HTTP request, or it acts as a front Controller for Spring based web applications. The DispatcherServlet dispatches a web request to the registered request handlers for processing the request and provides convenient mapping and exception handling facilities.
The DispatcherServlet is a very flexible class as it works around the JavaBeans configuration mechanism. We can define multiple DispatcherServlet in the web application. The DispatcherServlet class is defined in the org.springframework.web.servlet package.
Work Flow of Spring Web MVC
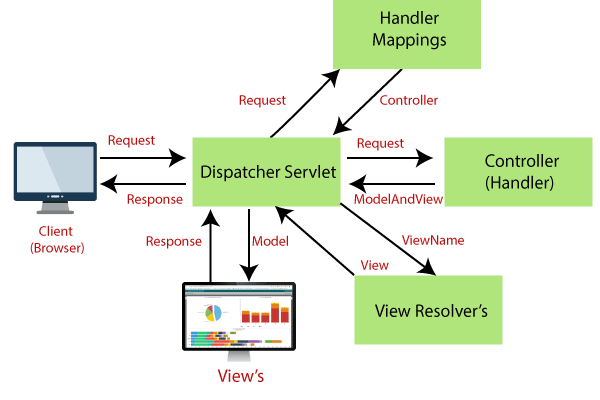
Step 1: The DispatcherServlet receives the web request.
Step 2: With the help of handlerMappings, DispatcherServlet, it finds the Controller associated with the given request and then returns the selected Controller to the DispatcherServlet.
Step 3: The request is transferred to the Controller. The Controller will process the request by executing appropriate methods and return the ModelAndView (holds Model data and View name) objects back to the DispatcherServlet.
Step 4: The DispatcherServlet sends the Model object to the View Resolver to get the actual View page associated with the View.
Step 5: In this step, the View page is used to display the result.
Benefits of Spring Web MVC
The Spring MVC framework is a robust, flexible, and well-designed framework for developing a web application. The benefits of the Spring MVC module are similar to other modules of the Spring framework.
The following figure shows the benefits of Spring MVC.
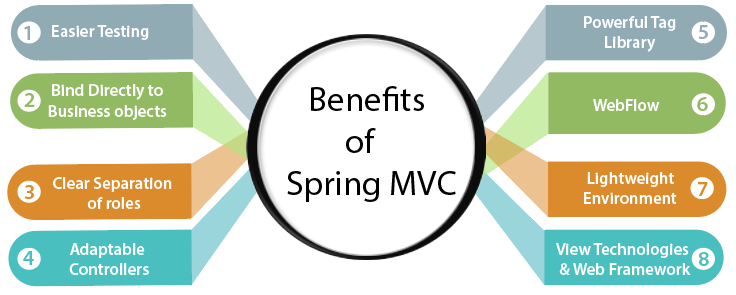
- Easier Testing: Most of the Spring classes are designed as JavaBeans that enables us to inject the test data using the setter method of that particular class. It also provides mock classes to produce Java HTTP objects, that makes testing of the web layer more comfortable.
- Bind directly to business objects: Spring MVC does not require business classes to extend any special class. It enables us to reuse our business objects by binding them with the HTML forms field. The controller class is the only one that is required to extend Spring classes.
- Clear separation of roles: Spring MVC provides the clear separation of roles played by various components of the framework. For example, the Spring controller has different functionalities as compared to the others.
- Adaptable controllers: Spring MVC provides adaptability, flexibility as we can define the required number of Controller method signature using one of the parameter annotations such as @RequestParam, @RequestHeader, @PathVariable, etc.
- Powerful tag library: Spring MVC framework provides a powerful tag library. For example, Spring MVC uses JSP Expression Language (EL) for arguments to <spring:bind> tag. The JSP tag library is also known as the Spring tag library.
- Web flow: Spring MVC is a sub-project and is not wrapped with the Spring core module. Spring web flow is a part of the web development stack, that includes Spring MVC. It is a powerful controller. It is used when the application requires complex controlled navigations.
- Lightweight environment: Like Spring, Spring MVC also supports POJO applications, that makes the Spring MVC more lightweight. The environment setup of Spring MVC can be more straightforward and less expensive as we can develop and deploy our applications using a lightweight Servlet container.
- View technologies & Web frameworks: Spring supports many view technologies such as JSP, Apache Velocity, FreeMarker, etc. We are going to use the JSP view technology in our tutorial. Spring also provides integration support for Apache Struts, Apache Tapestry, and Open Symphony’s WebWork.
Spring MVC
- Spring MVC Tutorial
- Spring MVC Example
- Spring MVC Example – Reading HTML form data
- Spring Model Interface
- Spring @RequestParam Annotation
Spring MVC Form Tag Library
- Spring MVC Form Tags
- Form Text field Example
- Form Drop-down Example
- Form Radio Button Example
- Form Checkboxes Example