Spring Tutorial
What is Spring Framework?
Spring is one of the most popular enterprise application framework used for developing Java applications. “Spring is a lightweight open-source framework. It allows Java developer to build simple, reliable, and scalable enterprise applications.” It was developed by Rod Johnson and was first released under the Apache 2.0 license in 2003. Most of the developers use the Spring framework to develop large scale applications. It also provides all the necessary tools to build complex applications.
Need of Spring Framework
The Spring framework has become popular in the Java community as an addition to, or replacement for the Enterprise JavaBeans (EJB). The EJB was not able to provide some important features such as transaction management, security, etc., which are required for developing secure applications. Also, developing an application with EJB was not easy; hence, Spring came with the solutions of all the complexities of the EJB.
The Spring framework uses new techniques such as Inversion of Control (IoC), Aspect-Oriented Programming (AOP), Plain Old Java Object (POJO), Dependency Injection (DI), and Transaction Management.
Advantages of Spring Framework
Following are the advantages of Spring framework:
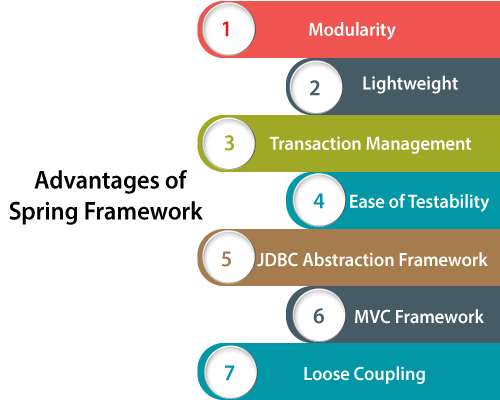
- Modularity – Spring is a flexible framework as it provides modularity to the developers. Spring framework provides many modules such as Spring Core Container, Spring AOP, Spring Test, Spring IoC, Spring MVC, Spring ORM, Spring OXM, and Spring Transactions which are used to develop complex applications.
- Lightweight – Spring is a lightweight framework. It also offers developers to use any of its modules selectively. It supports POJO applications, which makes Spring framework more lightweight.
- Transaction Management – Spring framework supports a unified abstraction layer for accessing the transaction services from various environments such as JTA, JDBC, JPA, and Hibernate. It also supports declarative transaction services similar to Java EE, and EJB.
- Ease of Testability – In Spring, testing of applications are easier because of dependency injection. Spring uses JavaBean style POJOs which makes the injection of test data simpler.
- JDBC Abstraction Framework – The Spring framework provides an abstraction layer which is used to solve problems such as Code duplication, Resource handling, and Exception handling.
- MVC Framework – Spring’s web framework is an attractive Web-MVC framework which simplifies the development of web applications in Java. Also, it is highly configurable and supports several view technologies like JSP, Tiles, etc.
- Loose Coupling – In Spring, the loose coupling can be achieved through a primary feature called Dependency Injection (DI) and IoC container (Inversion of Control). Dependency Injection keeps the Java classes independent from each other in order to achieve loose coupling between them.
Spring Modules
The Spring framework contains a lot of features which are further divided into twenty modules. These modules are grouped based on their features, such as:
- Spring Core Container
- Data Access/ Integration
- Web
- AOP and Instrumentation
- Test

Spring Core Container
The Spring Core Container layer consists of Beans, Core, Context, and ExpressionLanguage or SpEL modules. These modules are described below:
Bean & Core - The Bean & Core modules provide the fundamental parts of the framework. It is used to wrap the application and manage the objects/ instances in a wrapper that is called the ApplicationContext or BeanFactory. The BeanFactory allows a user to decouple the configuration and specification of dependencies from program logic. The BeanFactory interface is available in org.springframework.beans.factory package.
Context - The Core Container provides the Context module. It inherits its features from the Bean module. The context module offers a way to access objects in a framework-style manner which resembles a JNDI-registry. The Context module also supports some Java EE features like EJB, JMX, and Remote Management.
Expression Language (SpEL) - It provides a powerful expression language for querying and manipulating an object graph at runtime. This module supports some essential features like setting and getting of persistent values, property assignment, method invocation, accessing the array contexts, named variables, and retrieval of objects by name from Spring’s IoC Container.
Data Access/ Integration
This module layer allows a developer to use persistence APIs, like JDBC and Hibernate. These APIs are used to store persistent data in the database. It also enables a developer to write code to easily access persistent data throughout the application. It works with the relational database management system on the Java platform using JDBC and ORM (Object-Relational Mapping) tools.
The Data Access/ Integration layer contains the following modules:
JDBC– It provides a JDBC abstraction layer that removes the need to do monotonous coding and provides a solution for database-vendor specific codes.
ORM– It provides an integration layer for Object-Relational Mapping (ORM), such as JPA, JDO, etc. Through the ORM module, we can use all O/R-mappers with all of the Spring features, such as the simple declarative transaction management.
JMS–It provides support for Java Messaging Service (JMS) in the Spring framework. It can be used for both producing and receiving messages. It allows a user to send messages asynchronously.
OXM–It provides an abstraction layer for using Object/XML Mapping (OXM) implementations. The OXM supports technologies like JAXB, Castor, XMLBeans, and XStream.
Transaction –It helps in handling transaction management of an application without affecting its code. The transaction management module serves a consistent programming model across different transaction APIs such as Java Transaction API (JTA), Java Persistence API (JPA), Hibernate, and JDBC.
Web
The Web layer consists of Spring Web, Servlet, and Portlet modules:
Spring Web - It provides some web-oriented features like file-uploading functionality, the initialization of the IoC container through servlet listeners, and the application context. It also contains some parts of Spring’s remoting system.
Web-Servlet –It provides the Model-View-Controller (MVC) implementation for web applications. The Model-View-Controller (MVC) is an architectural pattern commonly used for organizing the application code. It divides the code into three interconnected sections, a Model, View, and Controller. The MVC gives an outline of the code.
Web-Portlet - It provides the MVC implementation used in the portlet environment.
Aspect-Oriented Programming (AOP) and Instrumentation
One of the key features of the Spring framework is Aspect-Oriented Programming (AOP). The AOP module is used to break down the application into aspects or concerns, and also known as modularization. It is also useful for implementing features like security, logging, transaction, and provides basic AOP features.
A separate Aspects module is available, which provides the integration with AspectJ.
There is a module Instrumentation which provides the class instrumentation support and classloader implementation, which is used in application servers.
Test
It contains the Test Framework which supports the testing of Spring components using JUnit or TestNG. It contains several Mock objects which are useful to test the code. We can also make use of the Integration test by creating an Application Context.
Spring Framework Tutorial
- Spring Framework Introduction
- Spring Inversion of Control (IoC)
- Spring Dependency Injection (DI)
- Spring Bean Scopes
- Spring Bean Lifecycle
Spring Annotations
- Spring Annotations
- Spring Inversion of Control using annotations
- Spring bean scopes using annotations
- Spring bean lifecycle using annotations
Autowiring
- Autowiring
- Autowiring by Constructor Injection
- Autowiring by Setter Injection
- Autowiring by Field Injection
Spring Configuration
- Spring configuration using @Configuration
- Spring beans using @Bean
- Injecting values from Property Files
Spring AOP
- Before Advice
- Pointcut Expressions
- AfterReturning Advice
- AfterThrowing Advice
- After Advice
- Around Advice
Spring MVC
- Spring MVC Tutorial
- Spring MVC Example
- Spring MVC Example – Reading HTML form data
- Spring Model Interface
- Spring @RequestParam Annotation
Spring MVC Form Tag Library
- Spring MVC Form Tags
- Form Text field Example
- Form Drop-down Example
- Form Radio Button Example
- Form Checkboxes Example