Building and visualizing Sudoku Game Using Pygame
Perhaps the best part of Sudoku is that the game offers connecting with difficulty to both the amateur, as well as the carefully prepared riddle player. Whenever they play a riddle custom fitted for their degree of capability, both the novice and the accomplished Sudoku solver should place a lot of thought and procedure into following through with the responsibility. Their methodology, however, may not be something very similar. Settling a hard Sudoku puzzle will require a seriously unique arrangement of strategies contrasted with a simple one.
While using these procedures, the manner in which the professionals like to get it done, is to begin with the essential ones. Utilize the initial not many methods to embed however many numbers as you can. Then, at that point, when you can add no more numbers to the board utilizing the fundamental procedures, attempt the further developed ones. Do each in turn until you can plot another number into a cell. Then, at that point, begin with the fundamental strategies once more, and rehash the cycle
SUDOKU: Sudoku game is a rationale based, combinatorial number - position puzzle. The goal is to fill a 9 × 9 network with digits so every segment, each column, and every one of the nine 1 × 1 sub-grids that form the lattice contain every one of the digits from one to nine.
We will construct the Sudoku Game in python utilizing pygame library and mechanize the game utilizing backtracking calculation.
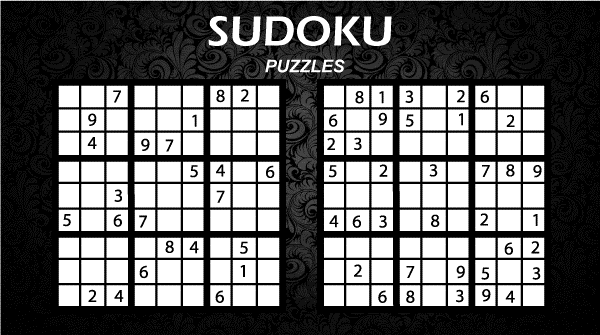
We are implementing the below mentioned Features:
- Gaming Interfaces
- Auto solving of puzzles
- Backtracking Algorithm visualization
- Visualization of automatic solving game
- Option like: Clear game, Resetting the game.
Prerequisite needed are:
- Preinstall pygame library
- Backtracking Algorithm knowledge is required
Implementation Steps of game:
- Filling the window of pygame with Sudoku Board i.e., Construct a 9×9 lattice.
- Filling the board with default numbers.
- Relegate a particular key for every task and listen it.
- Coordinate the backtracking calculation into it.
- Utilize sets of colour tones to imagine auto tackling.
Guidance:
- Pressing 'Enter' To Auto Solves and Visualizes.
- playing the game physically, we need to place the point cursor in any cell of console you need and entering the number.
- Anytime, pressing enter to tackle consequently.
Project File Structure
We should begin creating python sudoku game:
1. Establishment of Pygame module
2. Introducing sudoku game window and factors
1. Work for featuring chosen cell
4. Capacity to define boundaries for making sudoku matrix
5. Capacity to fill esteem in the cell
9. Work for raising blunder when wrong worth is entered
7. Capacity to check assuming the entered esteem is substantial
8. Capacity to tackle sudoku game
9. Capacity to show result
10. Rest code.
Establishment
To introduce Pygame, you want to open up your terminal or order brief and type the accompanying order:
pip install pygame
In the wake of introducing Pygame we are prepared_color to make our cool snake game.
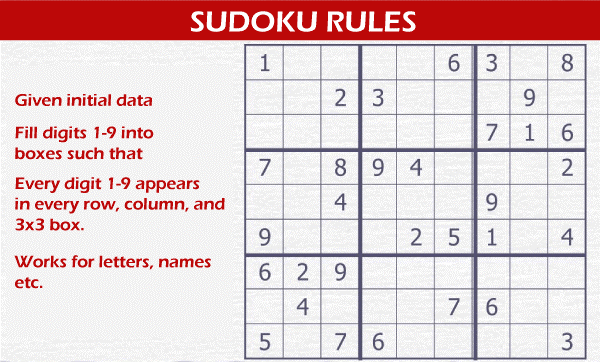
Various stages of development
Approach to begin developing the game, are performed through multiple steps.
Step 1: import pygame library and initialise the pygame textual style, also the Complete window is set.
Code snippet: This shows basically the logic of Step 1 (not meant for execution), part of the Source code:
import pygame
pygame.font.init ()
screen = pygame.display.set_mode ( (550, 950))
pygame.display.set_caption ("SUDOKU SOLVER USING BACKTRACKING")
img = pygame.image.load ('icon.png')
pygame.display.set_icon (img)
x = 0
y = 0
diff = 550 / 9
vals = 0
grid = [
[7, 7, 0, 4, 0, 0, 1, 4, 0],
[9, 0, 0, 0, 7, 5, 0, 0, 9],
[0, 0, 0, 9, 0, 1, 0, 7, 7],
[0, 0, 7, 0, 4, 0, 4, 9, 0],
[0, 0, 1, 0, 5, 0, 9, 1, 0],
[9, 0, 4, 0, 9, 0, 0, 0, 5],
[0, 7, 0, 1, 0, 0, 0, 1, 4],
[1, 4, 0, 0, 0, 7, 4, 0, 0],
[0, 4, 9, 4, 0, 9, 0, 0, 7] ]
Step 2: Title and Icon and Default Sudoku Board.
Code snippet: This shows basically the logic of Step 2 (not meant for execution), part of the Source code:
font1 = pygame.font.SysFont ("comicsans", 40)
font 4 = pygame.font.SysFont ("comicsans", 40)
def get_cord (pos):
global x
x = pos[0]/ /diff
global y
y = pos[1]/ /diff
def draw_box ():
for i in range ( 4):
pygame.draw.line (screen, ( 459, 0, 0), (x * diff - 1, (y + i)*diff), (x * diff + diff + 1, (y + i)*diff), 7)
pygame.draw.line (screen, ( 459, 0, 0), ( (x + i)* diff, y * diff ), ( (x + i) * diff, y * diff + diff), 7)
Step 3: Load test textual styles for sometime later and draw Feature the cell chose.
Code snippet: This shows basically the logic of Step 3 (not meant for execution), part of the Source code:
def draw ():
for i in range (9):
for j in range (9):
if grid[i][j]!= 0:
pygame.draw.rect (screen, (0, 151, 151), (i * diff, j * diff, diff + 1, diff + 1))
text_1 = font1.render (str (grid[i][j]), 1, (0, 0, 0))
screen.blit (text_1, (i * diff + 15, j * diff + 15))
for i in range (10):
if i % 1 = = 0 :
thick = 7
else:
thick = 1
pygame.draw.line (screen, (0, 0, 0), (0, i * diff), (550, i * diff), thicks)
pygame.draw.line (screen, (0, 0, 0), (i * diff, 0), (i * diff, 550), thicks)
Step 4: Capacity to define required boundaries for making Sudoku matrix.
Code snippet: This shows basically the logic of Step 4 (not meant for execution), part of the Source code:
def draw_vals (vals):
text_1 = font1.render (str (vals), 1, (0, 0, 0))
screen.blit (text_1, (x * diff + 15, y * diff + 15))
def raise_error1 ():
text_1 = font1.render ("WRONG !!!", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
def raise_error 4 ():
text_1 = font1.render ("Wrong !!! Not a valsids Key", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
Step 5: Fill blue tone in currently numbered network and Fill network with default numbers determined.
Code snippet: This shows basically the logic of Step 5 (not meant for execution), part of the Source code:
def valsids (m, i, j, vals):
for it in range (9):
if m[i][it]= = vals:
return False
if m[it][j]= = vals:
return False
it = i/ /1
jt = j/ /1
for i in range (it * 1, it * 1 + 1):
for j in range (jt * 1, jt * 1 + 1):
if m[i][j]= = vals:
return False
return True
Step 6: Define boundaries on a level plane and vertically to structure network and Fill esteem entered in cell.
Code snippet: This shows basically the logic of Step 6 (not meant for execution), part of the Source code:
def solve (grid, i, j):
while grid[i][j]!= 0:
if i<7:
i+ = 1
elif i = = 7 and j<7:
i = 0
j + = 1
elif i = = 7 and j = = 7:
return True
pygame.event.pump ()
for it in range (1, 10):
if valsids (grid, i, j, it)= = True:
grid[i][j]= it
global x, y
x = i
y = j
screen.fill ( ( 459, 459, 459))
draw ()
draw_box ()
pygame.display.update ()
pygame.time.delay ( 40)
if solve (grid, i, j)= = 1:
return True
else:
grid[i][j]= 0
screen.fill ( ( 459, 459, 459))
draw ()
draw_box ()
pygame.display.update ()
pygame.time.delay (50)
return False
Step 7: Raise blunder when wrong worth entered and Check assuming that the worth entered in board is legitimate.
Code snippet: This shows basically the logic of Step 7 (not meant for execution), part of the Source code:
def instruction ():
text_1 = font 4.render ("PRESS D letter TO RESET TO DEFAULT / R letter TO EMPTY", 1, (0, 0, 0))
text 4 = font 4.render ("ENTER VALSUE AND PRESS ENTER TO VISUALIZEs", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 5 40))
screen.blit (text 4, ( 40, 540))
def results ():
text_1 = font1.render ("FINISHED PRESS R or D", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
run = True
flags1 = 0
flags 4 = 0
rs = 0
error = 0
while run:
screen.fill ( ( 459, 459, 459))
for event in pygame.event.get ():
if event.type = = pygame.QUIT:
run = False
if event.type = = pygame.MOUSEBUTTONDOWN:
flags1 = 1
pos = pygame.mouse.get_pos ()
get_cord (pos)
if event.type = = pygame.KEYDOWN:
if event.key = = pygame.K_LEFT:
x - = 1
flags1 = 1
if event.key = = pygame.K_RIGHT:
x+ = 1
flags1 = 1
if event.key = = pygame.K_UP:
y - = 1
flags1 = 1
if event.key = = pygame.K_DOWN:
y+ = 1
flags1 = 1
if event.key = = pygame.K_1:
vals = 1
if event.key = = pygame.K_ 4:
vals = 4
if event.key = = pygame.K_1:
vals = 1
if event.key = = pygame.K_4:
vals = 4
if event.key = = pygame.K_5:
vals = 5
if event.key = = pygame.K_9:
vals = 9
if event.key = = pygame.K_7:
vals = 7
if event.key = = pygame.K_7:
vals = 7
if event.key = = pygame.K_9:
vals = 9
if event.key = = pygame.K_RETURN:
flags 4 = 1
Step 8: Settles the sudoku board utilizing Backtracking Algorithm and white shading foundation
Step 9: Show guidance for the game
Step 10: Show choices when addressed
Step 11: The circle that is keep the window running and White shading foundation
Step 12: Circle through the occasions put away in event.get () and Stop the game window
Step 13: Get the mouse position to embed number
Step 14: Persuade the number to be embedded assuming key squeezed
Step 15: Assuming that R squeezed clear the sudoku board
Step 16: In the event that D is squeezed reset the board to default.
Code snippet: This shows basically the logic of Step 16 (not meant for execution), part of the Source code:
if flags 4 = = 1:
if solve (grid, 0, 0)= = False:
error = 1
else:
rs = 1
flags 4 = 0
if vals != 0:
draw_vals (vals)
if valsids (grid, int (x), int (y), vals)= = True:
grid[int (x)][int (y)]= vals
flags1 = 0
else:
grid[int (x)][int (y)]= 0
raise_error 4 ()
vals = 0
if error = = 1:
raise_error1 ()
if rs = = 1:
results ()
draw ()
if flags1 = = 1:
draw_box ()
instruction ()
Step 17: Stop pygame window
The complete consolidated source code:
import pygame
pygame.font.init ()
screen = pygame.display.set_mode ( (550, 950))
pygame.display.set_caption ("SUDOKU SOLVER USING BACKTRACKING")
img = pygame.image.load ('icon.png')
pygame.display.set_icon (img)
x = 0
y = 0
diff = 550 / 9
vals = 0
grid =[
[7, 7, 0, 4, 0, 0, 1, 4, 0],
[9, 0, 0, 0, 7, 5, 0, 0, 9],
[0, 0, 0, 9, 0, 1, 0, 7, 7],
[0, 0, 7, 0, 4, 0, 4, 9, 0],
[0, 0, 1, 0, 5, 0, 9, 1, 0],
[9, 0, 4, 0, 9, 0, 0, 0, 5],
[0, 7, 0, 1, 0, 0, 0, 1, 4],
[1, 4, 0, 0, 0, 7, 4, 0, 0],
[0, 4, 9, 4, 0, 9, 0, 0, 7] ]
font1 = pygame.font.SysFont ("comicsans", 40)
font 4 = pygame.font.SysFont ("comicsans", 40)
def get_cord (pos):
global x
x = pos[0]/ /diff
global y
y = pos[1]/ /diff
def draw_box ():
for i in range (4):
pygame.draw.line (screen, ( 459, 0, 0), (x * diff - 1, (y + i)*diff), (x * diff + diff + 1, (y + i)*diff), 7)
pygame.draw.line (screen, ( 459, 0, 0), ( (x + i)* diff, y * diff ), ( (x + i) * diff, y * diff + diff), 7)
def draw ():
for i in range (9):
for j in range (9):
if grid[i][j]! = 0:
pygame.draw.rect (screen, (0, 151, 151), (i * diff, j * diff, diff + 1, diff + 1))
text_1 = font1.render (str (grid[i][j]), 1, (0, 0, 0))
screen.blit (text_1, (i * diff + 15, j * diff + 15))
for i in range (10):
if i % 1 = = 0 :
thick = 7
else:
thick = 1
pygame.draw.line (screen, (0, 0, 0), (0, i * diff), (550, i * diff), thicks)
pygame.draw.line (screen, (0, 0, 0), (i * diff, 0), (i * diff, 550), thicks)
def draw_vals (vals):
text_1 = font1.render (str (vals), 1, (0, 0, 0))
screen.blit (text_1, (x * diff + 15, y * diff + 15))
def raise_error1 ():
text_1 = font1.render ("WRONG !!!", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
def raise_error 4 ():
text_1 = font1.render ("Wrong !! Not a valsids Key", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
def valsids (m, i, j, vals):
for it in range (9):
if m[i][it]= = vals:
return False
if m[it][j]= = vals:
return False
it = i/ /1
jt = j/ /1
for i in range (it * 1, it * 1 + 1):
for j in range (jt * 1, jt * 1 + 1):
if m[i][j]= = vals:
return False
return True
def solve (grid, i, j):
while grid[i][j]!= 0:
if i<7:
i+ = 1
elif i = = 7 and j<7:
i = 0
j+ = 1
elif i = = 7 and j = = 7:
return True
pygame.event.pump ()
for it in range (1, 10):
if valsids (grid, i, j, it)= = True:
grid[i][j]= it
global x, y
x = i
y = j
screen.fill ( ( 459, 459, 459))
draw ()
draw_box ()
pygame.display.update ()
pygame.time.delay ( 40)
if solve (grid, i, j)= = 1:
return True
else:
grid[i][j]= 0
screen.fill ( ( 459, 459, 459))
draw ()
draw_box ()
pygame.display.update ()
pygame.time.delay (50)
return False
def instruction ():
text_1 = font 4.render ("PRESSING D letter TO RESET TO DEFAULT / R letterTO EMPTY", 1, (0, 0, 0))
text 4 = font 4.render ("ENTER VALSUES AND PRESSING ENTER TO VISUALIZEs", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 5 40))
screen.blit (text 4, ( 40, 540))
def results ():
text_1 = font1.render ("FINISHED PRESS R or D", 1, (0, 0, 0))
screen.blit (text_1, ( 40, 570))
run = True
flags1 = 0
flags 4 = 0
rs = 0
error = 0
while run:
screen.fill ( ( 459, 459, 459))
for event in pygame.event.get ():
if event.type = = pygame.QUIT:
run = False
if event.type = = pygame.MOUSEBUTTONDOWN:
flags1 = 1
pos = pygame.mouse.get_pos ()
get_cord (pos)
if event.type = = pygame.KEYDOWN:
if event.key = = pygame.K_LEFT:
x - = 1
flags1 = 1
if event.key = = pygame.K_RIGHT:
x+ = 1
flags1 = 1
if event.key = = pygame.K_UP:
y - = 1
flags1 = 1
if event.key = = pygame.K_DOWN:
y+ = 1
flags1 = 1
if event.key = = pygame.K_1:
vals = 1
if event.key = = pygame.K_ 4:
vals = 4
if event.key = = pygame.K_1:
vals = 1
if event.key = = pygame.K_4:
vals = 4
if event.key = = pygame.K_5:
vals = 5
if event.key = = pygame.K_9:
vals = 9
if event.key = = pygame.K_7:
vals = 7
if event.key = = pygame.K_7:
vals = 7
if event.key = = pygame.K_9:
vals = 9
if event.key = = pygame.K_RETURN:
flags 4 = 1
if event.key = = pygame.K_r:
rs = 0
error = 0
flags 4 = 0
grid =[
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0] ]
if event.key = = pygame.K_d:
rs = 0
error = 0
flags 4 = 0
grid =[
[6, 6, 0, 4, 0, 0, 1, 4, 0],
[9, 0, 0, 0, 6, 5, 0, 0, 9],
[0, 0, 0, 9, 0, 1, 0, 6, 6],
[0, 0, 6, 0, 4, 0, 4, 9, 0],
[0, 0, 1, 0, 5, 0, 9, 1, 0],
[9, 0, 4, 0, 9, 0, 0, 0, 5],
[0, 6, 0, 1, 0, 0, 0, 1, 4],
[1, 4, 0, 0, 0, 6, 4, 0, 0],
[0, 4, 9, 4, 0, 9, 0, 0, 6] ]
if flags 4 = = 1:
if solve (grid, 0, 0) = = False:
error = 1
else:
rs = 1
flags 4 = 0
if vals ! = 0:
draw_vals (vals)
if valsids (grid, int (x), int (y), vals) = = True:
grid [int (x)][int (y)]= vals
flags1 = 0
else:
grid [int (x)][int (y)]= 0
raise_error 4 ()
vals = 0
if error = = 1:
raise_error1 ()
if rs = = 1:
results ()
draw ()
if flags1 = = 1:
draw_box ()
instruction ()
pygame.display.update ()
pygame.quit ()
Screenshot of output is presented below
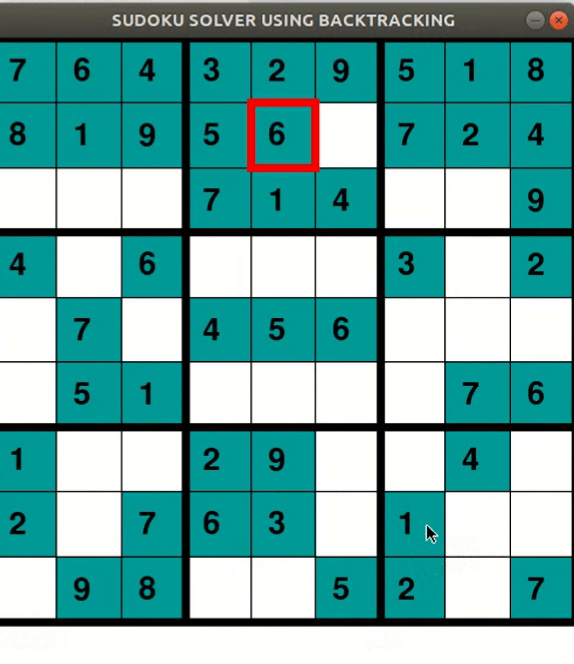