Pygame flip image
In this tutorial, we will perceive the way pictures can be flipped utilizing Pygame.
Pygame is a cross-stage sets of Python libraries aimed for composing video games. It has computer illustration and sounds libraries aimed to be utilized with the programming language python.
Pygame utilizes the Simple DirectMedia Layer (SDL) library, fully intent on permitting ongoing PC game improvement without the low-level mechanics of the C programming language and its subordinates. This depends with the understanding that the most costly capacities inside games can be disconnected from the game rationale, making it conceivable to utilize an undeniable level programming language, like Python, to structure the game.
Different highlights that SDL has incorporate vector math, crash recognition, 2D sprite scene chart the board, MIDI help, camera, pixel-exhibit control, changes, separating, progressed freetype textual style backing, and drawing.
Applications utilizing Pygame can run on Android telephones and tablets with the utilization of Pygame Subset for Android Sound, vibration, console, and accelerometer are upheld on Android.
Flip the image program
To flip the picture we really want to utilize pygame.transform.flip (Surface, x1bool, y1bool) technique which is called to flip the picture in vertical course or even heading as indicated by our requirements.
Syntax:
pygame.transform.flip (Surface, x1bool, y1bool)
Vertical direction Flipping image
In this, we need to flip the picture in an upward bearing. We will utilize pygame.transform.flip () to show the picture in vertical. Pass y1bool as False and x1bool as True, so the picture is flipped upward.
Input used:
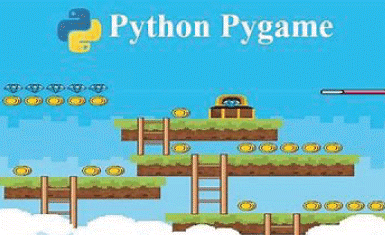
Step 1: importing all the modules like pygame and sys.
Code snippet (part of the Source code):
import pygame
import sys
from pygame.locals import *
Step 2: pygame.init () function will initialize all important required imported module.
Code snippet (part of the Source code):
pygame.init ()
pygame.display.set_caption ('Tutorial')
screen1 = pygame.display.set_mode ( (655, 455), 0, 35)
Step 3: screening size will display on screen
Step 4: pygame.image.load () function will return the object that has picture.
Code snippet (part of the Source code):
img1 = pygame.image.load ('images.png')
Step 5: picture Background colour is set
Step 6: picture copy is performed
Code snippet (part of the Source code):
while True:
screen1.fill ( (256, 256, 256))
img_copy1= img.copy ()
Step 7: pygame.transform.flip () will flip the picture.
Code snippet (part of the Source code):
img_with_flip = pygame.transform.flip (img_copy, True, False)
screen1.blit (img_with_flip, (55 + 1 * 125, 115))
Step 8: surface.blit () function draw a source picture
Step 9: Surface function onto this Surface picture.
Code snippet (part of the Source code):
for event in pygame.event.get ():
if event.type = = QUIT:
pygame.quit ()
Step 10: event listener to quit screen and update the frame per second
Code snippet (part of the Source code):
pygame.quit ()
sys.exit ()
pygame.display.update ()
The complete consolidated source code:
import pygame
import sys
from pygame.locals import *
pygame.init ()
pygame.display.set_caption ('Tutorial')
screen1 = pygame.display.set_mode ( (655, 455), 0, 35)
img1 = pygame.image.load ('images.png')
while True:
screen1.fill ( (256, 256, 256))
img_copy1= img.copy ()
img_with_flip = pygame.transform.flip (img_copy, True, False)
screen1.blit (img_with_flip, (55 + 1 * 125, 115))
for event in pygame.event.get ():
if event.type = = QUIT:
pygame.quit ()
sys.exit ()
pygame.display.update ()
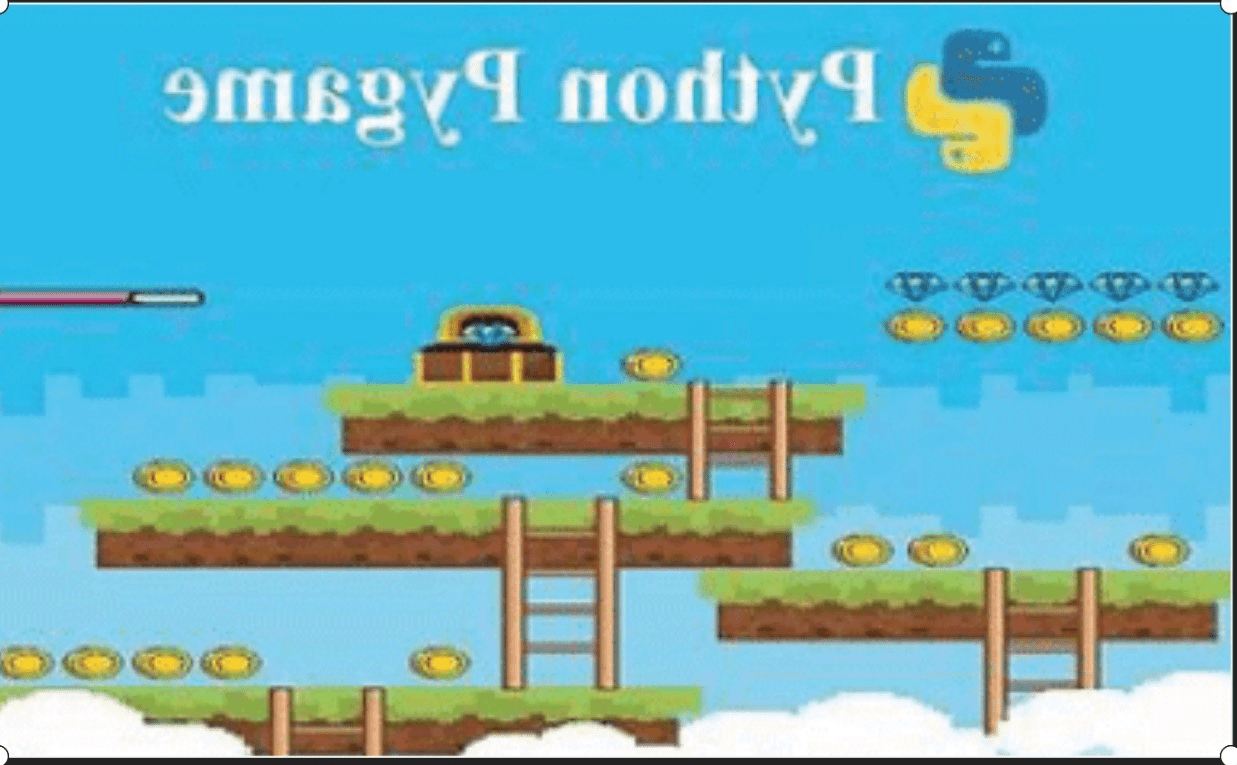
Horizontal Direction Flipping image
In this we have to flip the image in horizontal direction. For this x1bool is passed as False and y1bool as True, to flip it horizontally.
Step 1: importing all the modules like pygame and sys.
Code snippet (part of the Source code):
import pygame
import sys
from pygame.locals import *
Step 2: pygame.init () function will initialize all important required imported module.
Code snippet (part of the Source code):
pygame.init ()
pygame.display.set_caption ('Tutorial')
screen1 = pygame.display.set_mode ( (655, 455), 0, 35)
Step 3: screening size will display on screen
Step 4: pygame.image.load () function will return the object that has picture
Code snippet (part of the Source code):
img1 = pygame.image.load ('images.png')
Step 5: picture Background colour is set
Step 6: picture copy is performed.
Code snippet (part of the Source code):
while True:
screen1.fill ( (256, 256, 256))
img_copy1= img.copy ()
Step 7: pygame.transform.flip () will flip the picture.
Code snippet (part of the Source code):
img_with_flip = pygame.transform.flip (img_copy1, False, True)
screen1.blit (img_with_flip, (55 + 1 * 125, 115))
Step 8: surface.blit () function draw a source picture
Step 9: Surface function onto this Surface picture.
Code snippet (part of the Source code):
for event in pygame.event.get ():
if event.type = = QUIT:
pygame.quit ()
Step 10: event listener to quit screen and update the frame per second
Code snippet (part of the Source code):
pygame.quit ()
sys.exit ()
pygame.display.update ()
The complete consolidated source code:
# importinf the pygame and sys module
import pygame
import sys
from pygame.locals import *
pygame.init ()
pygame.display.set_caption ('Tutorial')
screen1 = pygame.display.set_mode ( (655, 455), 0, 35)
img1 = pygame.image.load ('images.png')
while True:
screen1.fill ( (256, 256, 256))
img_copy1= img.copy ()
img_with_flip = pygame.transform.flip (img_copy1, False, True)
screen1.blit (img_with_flip, (55 + 1 * 125, 115))
for event in pygame.event.get ():
if event.type = = QUIT:
pygame.quit ()
sys.exit ()
pygame.display.update ()
Screenshot of output is presented below
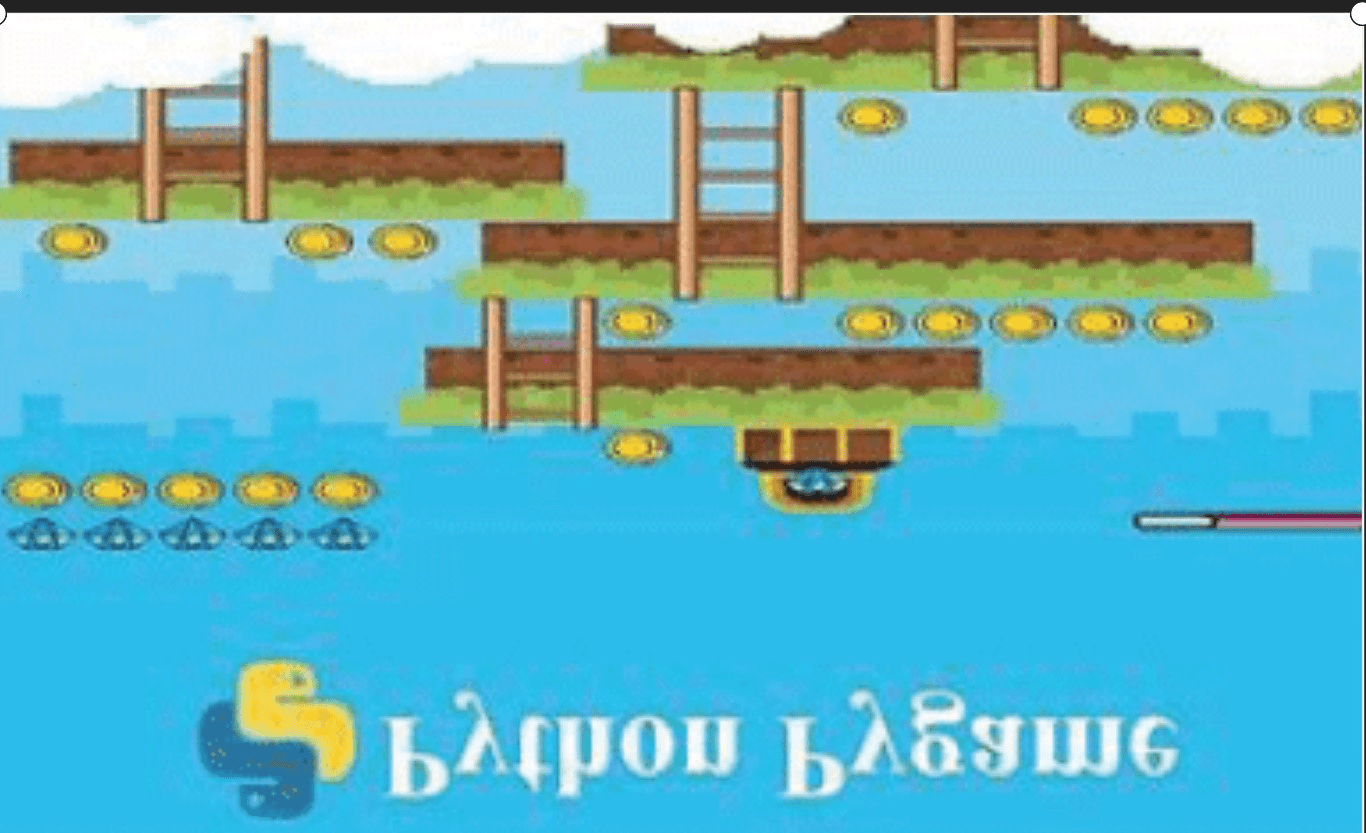