Pygame Sprites
What are sprites?
Sprites are defined same as objects, with various properties like tallness, width, shading, and so forth, and techniques like moving right, left, all over, bounce, and so on. In this article, we are hoping to make an item in which clients can handle that article and push it ahead, in reverse, up, and down utilizing bolt keys.
For instance, in a round of breakout, you may observe the accompanying sprite objects:
- The ball is a sprite that moves around the screen, skipping off things and obliterating blocks.
- The bat is a sprite that the client controls with the console/mouse/joystick.
- Every block is a sprite. Blocks don't generally move, however they are obliterated when the ball hits them.
- The dividers may likewise be comprised of sprites. They don't normally move or get obliterated, yet the ball skips off them.
Creating sprite example
Let first gander at our five star i.e., the class where our sprite is characterized, we will call that class Sprite. This Sprite class characterizes its positions (x and y arranges), aspect of an item, shading, and so forth To begin with, we will call our __init__() strategy. It is known as a constructor for a class.
Below is the Source code for just creating sprite class:
- Step: Importing pygame module
- Step: Setting global variables like color, surface color, width, height.
- Step: Creating class sprite
- Step: Initializing class with arguments
- Step: Calling parent class init() method by using super keyword
- Step: Using parent class methods and variables.
- Step: Drawing window rectangle screen.
import pygame
COLOR = (256, 150, 89)
SURFACE_COLOR = (167, 256, 150)
WIDTH1 = 550
HEIGHT1 = 550
# GLOBAL VARIABLES
# Object class
class Sprite (pygame.sprite.Sprite):
def __init__ (self_, color, HEIGHT1, WIDTH1):
super ().__init__ ()
self_.image = pygame.Surface ([WIDTH1, HEIGHT1])
self_.image.fill (SURFACE_COLOR)
self_.image.set_colorkey (COLOR)
pygame.draw.rect (self_.image, color, pygame.Rect (0, 0, WIDTH1, HEIGHT1))
self_.rect = self_.image.get_rect ()
Presently, that the class has been made, we can make objects from the class. It empowers us to make however many articles as we really want utilizing a similar class. Presently we will make an item utilizing our Class Sprite.
Syntax:
object = Sprite (RED,WIDTH,HEIGHT)
Naturally, the item will be on position (0,0) i.e., upper left of the screen. We can change the x and y properties of the item.
Syntax:
object.rect.x = esteem
object.rect.y = esteem
We can characterize n of sprites that we need to make, yet to comprehend, we should rearrange. Here we have made a square shape sprite of specific aspects, on which we can perform various tasks to perform on sprites like push ahead, in reverse, hop, slow, speed up, and so on
Tasks to perform on sprites like push ahead, in reverse etc.
There might be different sprites, for instance gold coins or bombs may fall from time to time that the player needs to attempt to get or stay away from with the bat. In the event that your game shows the current score and the quantity of lives left, they may likewise be sprites.
Approach to begin with the code:
- Step: Initialize the screen window
- Step: we start pygame with pygame.init ( ), instate pygame and the screen
- Step: setting global variables
- Step: Grouping spirites sprite.Group ()
- Step: Setting the image rect = self_.image.get_rect ()
- Step: setting height, length, surface color.
- Step: then, at that point, we make the clock object to control the edge rate
- Step: setting clock timer
- Step: we give the window a title with set_caption( ) technique for show à display.set_caption ("Creating Sprite")
Below is the Combined (sprite class + task code) Source code:
import pygame
import random
# GLOBAL VARIABLES
# Object class
COLOR = (256, 150, 89)
SURFACE_COLOR =(167, 256, 150)
WIDTH1 = 550
HEIGHT1 = 550
class Sprite (pygame.sprite.Sprite):
def __init__ (self_, color, HEIGHT1, WIDTH1):
super ().__init__ ()
self_.image = pygame.Surface ([WIDTH1, HEIGHT1])
self_.image.fill (SURFACE_COLOR)
self_.image.set_colorkey (COLOR)
pygame.draw.rect (self_.image,color,pygame.Rect (0, 0, WIDTH1, HEIGHT1))
self_.rect = self_.image.get_rect ()
pygame.init ()
RED = (256, 0, 0)
size = (WIDTH1, HEIGHT1)
screen = pygame.display.set_mode (size)
pygame.display.set_caption ("Creating Sprite")
all_sprites_list = pygame.sprite.Group ()
object_ = Sprite (RED, 20, 30)
object_.rect.x = 200
object_.rect.y = 300
all_sprites_list.add (object_)
exit = True
clock = pygame.time.Clock ()
while exit:
for event in pygame.event.get ():
if event.type = = pygame.QUIT:
exit = False
all_sprites_list.update ()
screen.fill (SURFACE_COLOR)
all_sprites_list.draw (screen)
pygame.display.flip ()
clock.tick (60)
pygame.quit ()
Explanation:
As we have created global variables, to regulate those, means we have to order them with the help of group class. The Group class is only a basic compartment. Like the sprite, it has an add() and eliminate() technique which can change which sprites have a place with the gathering. You additionally can pass a sprite or rundown of sprites to the constructor (__init__() technique) to make a Group example that contains a few beginning sprites.
The Group has a couple of different strategies like vacant() to eliminate all sprites from the gathering and duplicate() which will return a duplicate of the gathering with overall similar individuals. Likewise the has() technique will rapidly check in the event that the Group contains a sprite or rundown of sprites.
The other capacity you will utilize regularly is the sprites() strategy. This profits an item that can be circled on to get to each sprite the gathering contains. As of now this is only a rundown of the sprites, yet in later form of python this will probably utilize iterators for better execution.
Screenshot of output is presented below:
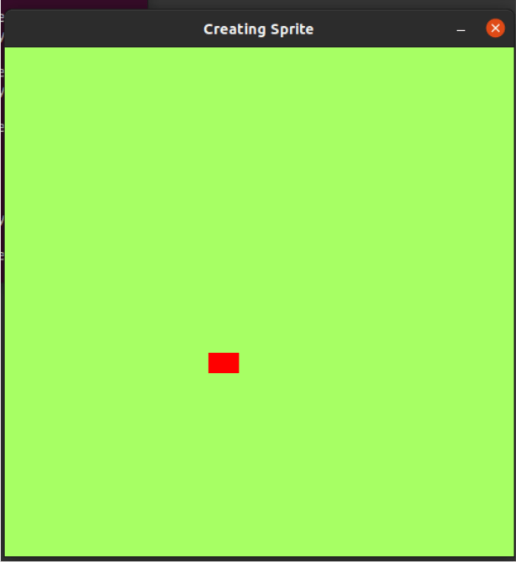