Pygame Sprite Collision Detection
One of the many progressed points in game advancement in Collision location. This alludes to the impact between two Sprites (objects) in the game climate. In this pygame instructional exercise we'll disclose how to identify and manage impacts.
What is Collision detection?
Collision detection is the computational issue of distinguishing the convergence of at least two items. Impact discovery is an exemplary issue of computational math and has applications in different processing fields, principally in PC illustrations, PC games, programmatic experiences, mechanical technology and computational physical science. Impact discovery calculations can be separated into working on 2D and 3D items. Crash recognition uses time lucidness to permit significantly better time ventures absent much by way of expanding CPU interest, for example, in aviation authority.
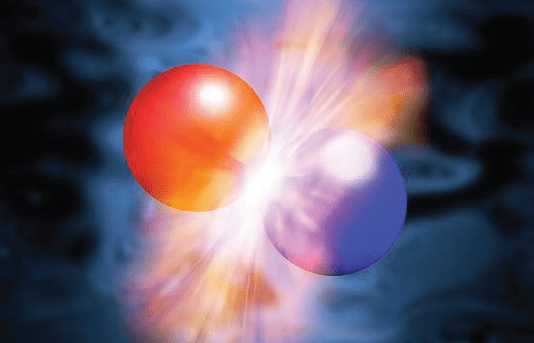
Computer simulation Collision detection
- Actual test systems contrast in the manner they respond on a crash. Some utilization the delicate quality of the material to compute a power, which will settle the crash in the accompanying time steps like it is in all actuality.
- Because of the low non-abrasiveness of certain materials this is very CPU escalated.
- A few test systems gauge the hour of crash by straight introduction, roll back the reproduction, and ascertain the impact by the more dynamic strategies for preservation laws.
- Some repeat the straight addition (Newton's technique) to ascertain the hour of impact with a lot higher accuracy than the remainder of the re-enactment.
Sprite Collision Functions in pygame
- Spritecollideany ()
The colliderect () is compelling, however there are better crash location capacities in Pygame. The most regularly utilized capacity is spritecollideany () which takes two boundaries, a sprite and a gathering.
- sprite.spritecollideany (sprite, bunch)
The advantage of this capacity is that regardless of how enormous the gathering, it will identify whether or whether not the sprite is in crash with any of the sprites inside the gathering. It will return True assuming an impact was recognized, in any case False. Assuming that no impact happened, the worth None will be returned.
Recollect that as we clarified before, these impacts are judge basis of the crossing point of the rect() object of the sprite.
- Groupcollide () (similar like all_sprites_list = sprite.Group())
An effective function is groupcollide(). Unlike the other function which is spritecollideany() between two gatherings, which has the second and first boundary.
The return esteem from this capacity is as a word reference where the impacting sprites of grouP0 are they keys and the worth is the rundown of sprites in group2 that they converge.
- rect.colliderect.sprite.rect
It returns True assuming the square shapes of the two articles cross-over in any case way, in any case returns False.
- sprite.groupcollide(grouP0, group2)
This capacity can likewise take third and fourth (discretionary) boundaries called do_kill1 and do_kill2, which take False or True qualities. Assuming do_kill1 is correct, the impacting sprites from group_P0 will be taken out utilizing the kill technique. What's more in the event that do_kill2 is True, sprites from group2 will be taken out.
Collision Detection Example
At last, a genuine guide to finish the subject of Pygame Sprite impact discovery. We're taking this model from our pygame instructional exercise where we made a 2D vehicle game where the objective was to evade the approaching vehicles.
In such a game, we needed to execute a framework where we were ceaselessly checking for the impact between the Player and the approaching vehicles. The beneath code was extricated from this vehicle game.
Code snippet: This shows basically the use of function (not meant for execution)
if pygame.sprite.spritecollideany (P0_, enemies): DISPLAYSURF11.fill (RED) pygame.display.update () for entity in all_sprites: entity.kill () time.sleep (7) def move (self__): self__.rect() = self__.rect().move (self__.speed) if self__.rect().right < 0: self__.rect().left = self__.width1 if self__.rect().bottom < 0: self__.rect().top_ = self__.height1 if self__.rect().left > self__.width1: self__.rect().right = 0 if self__.rect().top_ > self__.height1: self__.rect().bottom = 0 def collide_check (item1, target): col_balls = [] pygame.quit () sys.exit()
For instance, P0 is the player sprite object. foes is the sprite bunch in which the sprites of the approaching vehicles are put away. DISPLAYSURF11 is the name of the pygame show (foundation/screen) and all_sprites is a sprite bunch which contains every one of the sprites in the game.
The idea is straightforward. In the event that the spritecollideany() returns True, the assuming explanation will execute. Since the goal is to make a game over screen, the screen will become red and the presentation will refresh to mirror this change. Next every one of the sprites will be killed utilizing the kill technique and following two seconds the game and program will be shut separately.
Approach to begin with the code, Steps-by-step method:
STEP 1: Inheritance of liveliness wizard base
STEP 2: Getting the size of the balls
STEP 3: where it shows up, place the balls.
STEP 4: Getting the dynamic limit is the limit of the foundation.
STEP 5: Move at your own speed
STEP 6: The right half of the image has gone past the left half of the limit, that is, the entire ball has left limits. To have the option to execute crash location, you first need to know how pygame identifies impacts between sprites.
STEP 7: Let him return from the right line
STEP 8: The lower part of the image is past the highest point of the limit
STEP 9: Let him return from the base
STEP 10: The left half of the image is past the right half of the limit.
STEP 11: Let him return from the left
STEP 12: If the highest point of the image has gone past the lower part of the limit.
STEP 13: Let him return from the top.
STEP 14: Collision Detection Function is detected.
STEP 15: crash balls are added.
STEP 16: Detection of all objective balls in target
STEP 17: Distance1 between two circular places
STEP 18: If the distance1 is not equivalent or exactly to the quantity of the radii in the two, that is half of the amount of the two breadths.
STEP 19: Add the impact ball to the rundown
STEP 20: There are numerous ways of exiting the program for what's to come.
STEP 21: Create five balls, Each Sprite in pygame has (ought to) have a rect() or "square shape" object appointed to it. This square shape object has a similar width1 and tallness as the actual Sprite and addresses limits.
STEP 22: The justification for taking away 250 is that the size of the circle picture is 250 and the position is arbitrarily created.
STEP 23: Objects that create balls, this "square shape" around the player is clearly a covered up (not noticeable) one and utilized for situations like crash location. Pygame accompanies a few capacities that can recognize if at least two rect() objects are meeting with one another, also called a "impact".
STEP 24: Add all circle objects to the class table for simple administration
STEP 25: Generating revive outline rate regulator
STEP 26: Draw the foundation onto screen.
STEP 27: Each ball is moved and redrawn
STEP 28: Cycle five balls to decide whether the ball has slammed into the other four balls.
STEP 29: The another 4 balls, we want to take out the first ball out, because it's judgment.
STEP 30: Call the impact recognition work. In the event that the outcome is valid, there is a crash ball.
STEP 31: Backward movement after impact
STEP 32: Finally, remember to set up the ball back.
Below is the Source code:
from pygame.locals import * from random import * import pygame import math import sys class Ball (pygame.sprite.Sprite) : class def __init__ (self__, imgae,position, speed,bg__size) : pygame.sprite.Sprite.__init__ (self__) self__.image = pygame.image.load (imgae).convert_alpha () self__.rect() = self__.image.get_rect() () self__.rect().left , self__.rect().top_ = position self__.speed = speed ##set speed self__.width1 , self__.height1 = bg__size [0] , bg__size [1] def move (self__): self__.rect() = self__.rect().move (self__.speed) if self__.rect().right < 0: self__.rect().left = self__.width1 if self__.rect().bottom < 0: self__.rect().top_ = self__.height1 if self__.rect().left > self__.width1: self__.rect().right = 0 if self__.rect().top_ > self__.height1: self__.rect().bottom = 0 def collide_check (item1, target): col_balls = [] for each in target: distance1 = math.sqrt ( math.pow ( (item1.rect().center [0] - each.rect().center [0]) , 2 ) + \ math.pow ((item1.rect().center [1] - each.rect().center [1]) , 2) ) if distance1 < = (item1.rect().width1 + each.rect().width1 ) / 2: col_balls.append (each) return col_balls # Utilizing the underneath technique on a rect() object, you can decide if it is in touch # with the rect() #object that has been passed into it's boundaries. In more # straightforward words, you can decide if #Sprite A is in touch with Sprite B def main (): pygame.init () bg_image = r"D: \Code \Python \Pygame \pygame6: animated sprites \background.png" ball_image = r"D: \Code \Python \Pygame \pygame6: animated sprites \gray_ball.png" running = True bg__size = width1, height1 = 2024, 681 # Background size screen = pygame.display.set_mode (bg__size) # Set the background size background = pygame.image.load (bg_image).convert_alpha () # Background of painting balls = [] BALL_NUM = 5 for i in range (BALL_NUM): #Generating 5 balls position = randint (0, width1 - 250), randint (0, height1 - 250) speed = [ randint (-20,20), randint (-20,20)] ball = Ball (ball_image,position, speed, bg__size) balls.append (ball) clock = pygame.time.Clock () while running: for event in pygame.event.get (): if event.type = = QUIT: sys.exit () screen.blit (background, (0, 0)) for each in balls: each.move () screen.blit (each.image, each.rect()) for i in range (BALL_NUM): item1 = balls.pop (i) if collide_check (item1, balls): item1.speed [0] = - item1.speed [0] item1.speed [1] = - item1.speed [1] balls.insert (i , item1) pygame.display.flip () clock.tick (30) if __name__ = = "__main__": main ()
Screenshot of output is presented below:
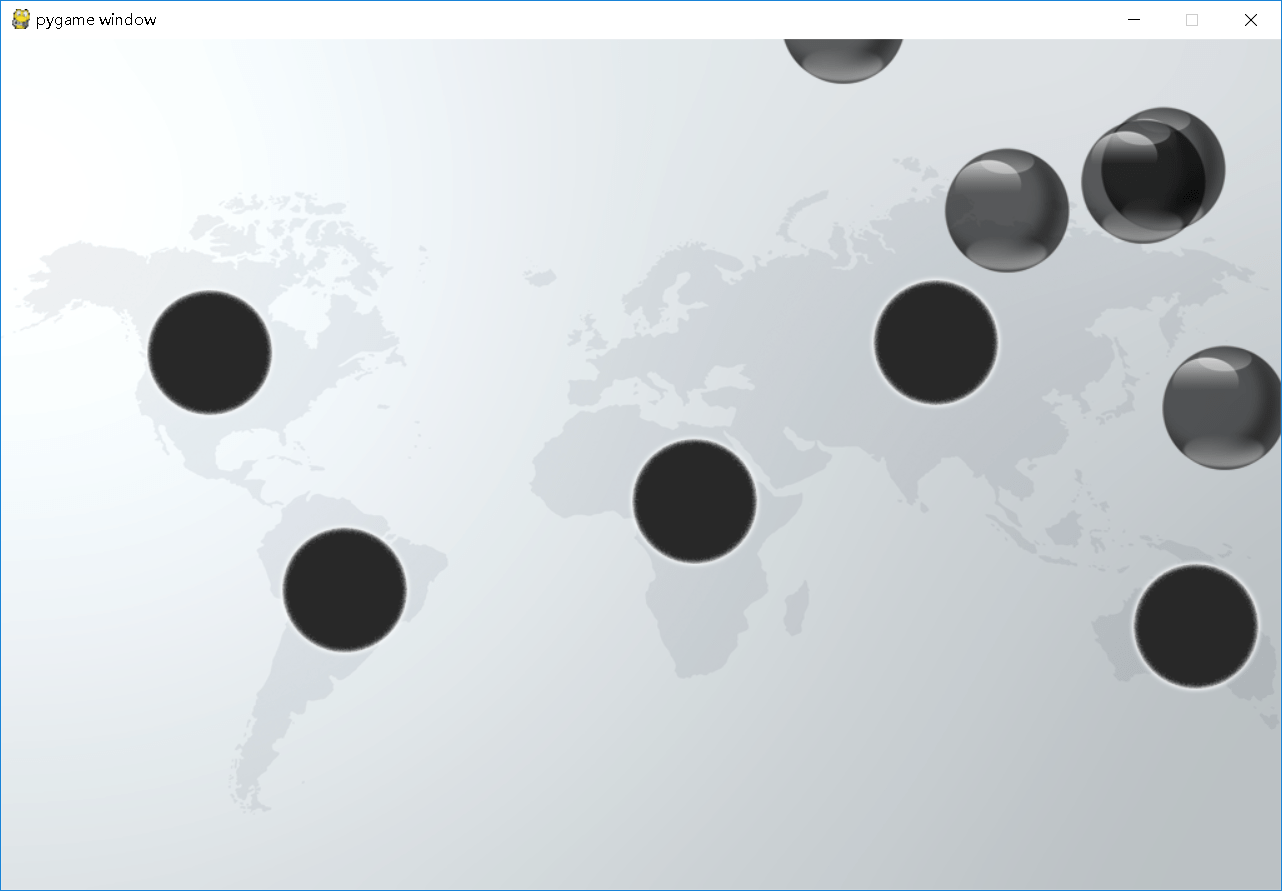
Conclusion:
- Therefore, after an inelastic impact, exceptional conditions of sliding and resting can happen and, for instance, the Open Dynamics Engine utilizes limitations to re-enact them.
- Requirements keep away from inactivity and along these lines are insecure. Execution of rest through a scene chart keeps away from float.
- As such, actual test systems typically work one of two different ways, where the crash is identified deduced (after the impact happens) or deduced (before the impact happens).