Pygame Controling Sprites
Now we will examine how to control the sprite, such as pushing ahead, in reverse, slow, or speed up, and a portion of the properties that sprite ought to have. We will add occasion overseers to our program to react to keystroke occasions, when the player utilizes the bolt keys on the console we will call our unadulterated strategies to move the article on the screen.
Capacities Used in our examples
- moveRight(): This technique takes contention pixels, which implies the number of pixels an article ought to be moved in the correct bearing. It is essentially adding pixels to the current x direction of the item.
- moveLeft(): This strategy takes contention pixels, which implies the number of pixels an item ought to be moved in the left heading. It is fundamentally deducting pixels to the current x direction of the item.
- moveForward(): This strategy takes a contention speed, which implies by the number of variables the speed will increment. It is essentially speeding up with the component of n in the y-heading of the article.
- moveBackward(): This technique takes a contention speed, which implies by the number of elements the speed will diminish. It is essentially diminishing velocity with the variable of n in the y-course of the article.
Creating sprite class
First step is to create sprite class, so allow us first to check out the execution of a Sprite class, which assists us with making an article on our PyGame surface, and alongside added 4 techniques that will assist us with pushing ahead, in reverse, both ways.
Example: Below is the Source code for just creating sprite class
- Step: Importing pygame module
- Step: Setting global variables like color, surface color, width, height.
- Step: Creating class sprite
- Step: Initializing class with arguments
- Step: Calling parent class init() method by using super keyword
- Step: Using parent class methods and variables.
- Step: Drawing window rectangle screen.
import pygame # GLOBAL VARIABLES COLOR = (256, 150, 89) SURFACE_COLOR = (167, 256, 150) WIDTH1 = 550 HEIGHT1 = 550 # Object class class Sprite (pygame.sprite.Sprite): def __init__ (self_, color, HEIGHT1, WIDTH1): super ().__init__ () self_.image = pygame.Surface ([WIDTH1, HEIGHT1]) self_.image.fill (SURFACE_COLOR) self_.image.set_colorkey (COLOR) pygame.draw.rect (self_.image, color, pygame.Rect (0, 0, WIDTH1, HEIGHT1)) self_.rect = self_.image.get_rect () def moveRight (self_, pixels): self_.rect.x + = pixels def moveLeft (self_, pixels): self_.rect.x - = pixels def moveForward (self_, speed): self_.rect.y + = speed * speed/15 def moveBack (self_, speed): self_.rect.y - = speed * speed/15
Explanation of code:
Presently we will perceive how we have control of our principle program circle to deal with the sprites. The initial segment of the circle will react to the occasions, for example, cooperations when the client utilizes the mouse or the console. Afterward, on above techniques for occasion dealing with on our article will be dealt with. Every occasion controller will call the pertinent technique from the Sprite class.
In this piece of code, we have control of i.e., our item is an article according to our given headings, assuming we press the right bolt key, it will move that way and the equivalent with all the bolt keys. Here, we use pygame.KEYDOWN strategy to introduce the technique to involve the bolt keys for controlling the articles, later on, we need to control the particular strategy to trigger the particular key to play out the specific activity.
For example, on the off chance that we have a right bolt key, we need to call pygame.K_RIGHT technique to move towards solidly toward the article, and comparable for pyagme.K_DOWN strategy which is utilized for the move up toward the item.
Example: Controlling sprite
At the point when you plan a game, it is generally expected a smart thought to attempt to make every sprite answerable for as its very own lot conduct as possible. That way, your fundamental program doesn't need to do much by any means.
In the model, assuming you bat, ball, blocks and dividers all take care of themselves, your primary program barely needs to do anything. It simply needs delay until either every one of the blocks are gone (Win!) or the ball tumbles to the lower part of the screen (Lose!).
Planning the game like that additionally makes it simpler to add new elements.
Approach to begin with the code, Steps-by-step method:
- Step: Initialize the screen window
- Step: we start pygame with pygame.init ( ), instate pygame and the screen
- Step: instate the text dimension and shading
- Step: Grouping spirites sprite.Group ()
- Step: Setting the image rect = self_.image.get_rect ()
- Step: make a text style object in Comin Sans MS
- Step: then, at that point, we make the clock object to control the edge rate
- Step: we make the SURFACE object
- Step: we give the window a title with set_caption( ) technique for show à display.set_caption ("Creating Sprite")
Below is the Source code:
import pygame # Global Variables COLOR = (256, 150, 89) SURFACE_COLOR = (167, 256, 150) WIDTH1 = 550 HEIGHT1 = 550 # Object class class Sprite (pygame.sprite.Sprite): def __init__ (self_, color, HEIGHT1, WIDTH1): super ().__init__ () self_.image = pygame.Surface ([WIDTH1, HEIGHT1]) self_.image.fill (SURFACE_COLOR) self_.image.set_colorkey (COLOR) pygame.draw.rect (self_.image, color, pygame.Rect (0, 0, WIDTH1, HEIGHT1)) self_.rect = self_.image.get_rect () def moveRight (self_, pixels): self_.rect.x + = pixels def moveLeft (self_, pixels): self_.rect.x - = pixels def moveForward (self_, speed): self_.rect.y + = speed * speed/15 def moveBack (self_, speed): self_.rect.y - = speed * speed/15 pygame.init () RED = (256, 0, 0) size = (WIDTH1, HEIGHT1) screen = pygame.display.set_mode (size) pygame.display.set_caption ("Creating Sprite") class Ball(pg.sprite.Sprite): def __init__(self, pos): super(Ball, self).__init__() self.image = pg.image.load(os.path.join('resources', 'ball.png')) self.rect = self.image.get_rect() self.rect.center = pos def update(self): pass all_sprites_list = pygame.sprite.Group () playerCar = Sprite (RED, 20, 30) playerCar.rect.x = 200 playerCar.rect.y = 300 all_sprites_list.add (playerCar) exit = True clock = pygame.time.Clock () running = True while running: for event in pg.event.get(): if event.type = = pg.QUIT: running = False screen.fill ( (0, 0, 0)) group.draw (screen) pg.display.flip () while exit: for event in pygame.event.get (): if event.type = = pygame.QUIT: exit = False elif event.type = = pygame.KEYDOWN: if event.key = = pygame.K_x: exit = False keys = pygame.key.get_pressed () if keys[pygame.K_LEFT]: playerCar.moveLeft (15) if keys[pygame.K_RIGHT]: playerCar.moveRight (15) if keys[pygame.K_DOWN]: playerCar.moveForward (15) if keys[pygame.K_UP]: playerCar.moveBack (15) all_sprites_list.update () screen.fill (SURFACE_COLOR) all_sprites_list.draw (screen) pygame.display.flip () clock.tick (60) pygame.quit ()
Code explanation:
- Fills the screen surface with shading (0, 0, 0), which addresses dark. (It can attract itself on the screen the right position.)
- Calls group.draw to draw the gathering of sprites onto the screen surface. bunch is the RenderDraw object we made before. Our gathering of sprites just holds back one sprite, the ball we made before.
- Considers flip that moves the things we drew on the screen surface into the video support so we can really see it (see beneath).
- The outcome is a window with the ball picture drawn at position (100, 200).
Screenshot of output is presented below:
First snap of resulted output of moving sprite
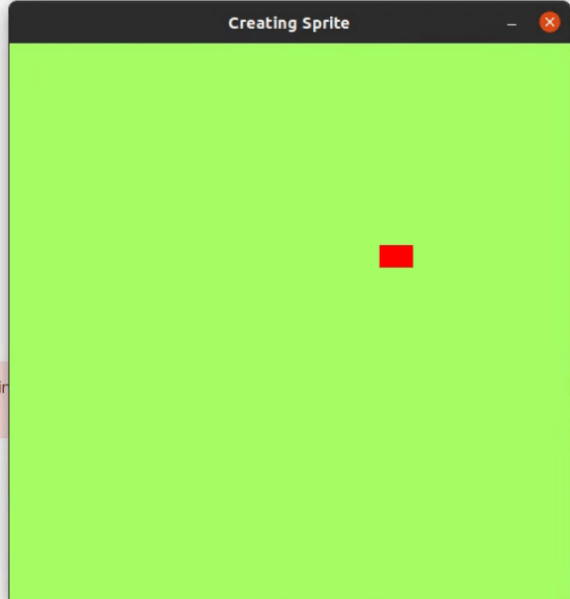
Second snap of resulted output of moving sprite
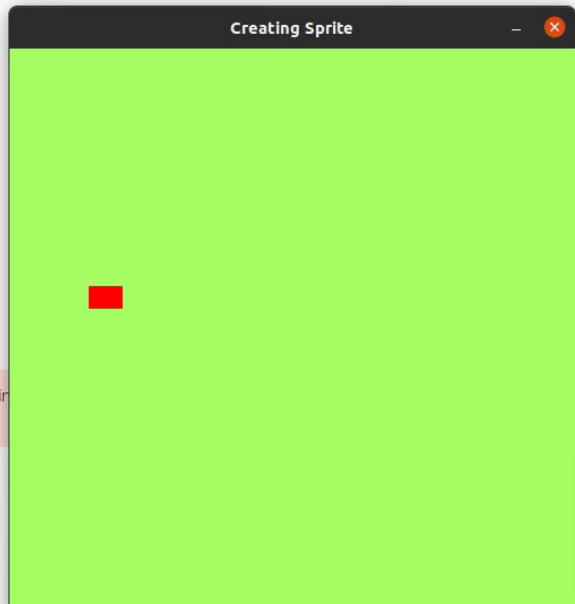
Conclusion
Therefore, to sum up the capabilities of pygame sprites, each sprite is a self-contained object. It has many intrinsic capabilities:
- It knows how to move. For instance, the ball will move in an orderly fashion except if it hits something.
- It knows what to do assuming it slams into another sprite. For instance, the ball will ricochet off anything it slams into.
- It can annihilate itself. For instance, a block will annihilate itself assuming it crashes into the ball (or all the more precisely, on the off chance that the ball crashes into the block).
- It can change its appearance. For instance, you may have a few extra solid blocks that don't get annihilated until the ball has hit them multiple times. The block may look increasingly more harmed each time it is hit, until it is obliterated.