Spring Boot DevTools
Spring Boot 1.3 delivers one more module known as Spring Boot DevTools, an abbreviation for Developer Tool. The purpose of this module is to improve development time when using Spring Boot applications. Spring Boot DevTools applies the changes and restarts the application.
You can implement DevTools in your project by providing the subsequent dependency to the pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime<scope >
</dependency>
Features
Delivers the features as mentioned below:
- Property Default
- AutoReload
- LiveReload
- Remote Debug Tunneling
- Remote Update and Reboot
Property Default: Spring Boot provides the template technology Thymeleaf with the property spring.thymeleaf.cache. Disables the cache so it can restore the page without restarting the application. However, setting these properties during development always causes some problems.
Using the spring-boot-devtools module, you don't need to set any properties during development. The Thymeleaf, Freemarker, and Groovy template caches are automatically disabled.
Note: If you don't want to apply property defaults to your application, you can set configprop: spring.devtools.addproperties [] to false in the application.properties file.
Autorestart: Auto-restart means the Java class is reloaded and configured on the server side. After the server-side changes are modified, they are dynamically deployed, a server restart occurs, and the modified code is loaded. It is primarily used in microservices-based applications. Spring Boot utilises the following kinds of ClassLoader:
- The entire class (ThirdJars) is loaded into the base ClassLoader.
- Rebooting the ClassLoader will load the class you are vigorously developing.
When you resume the application, the restart class loader is destroyed, and the new class loader fills up. Thus, the base ClassLoader is consistently functional and full.
To disable automatic server restart, make spring.devtools.restart.enabled property to false.
Remember:
- DevTools constantly monitors classpath resources. There is a single way to update the classpath to trigger a reboot.
- DevTools needed another application class loader to perform adequately. By default, Maven branches the application process.
- Autoreboot works fine with LiveReload.
- DevTools depend on the application context's shutdown hook to shut the application context during a reboot.
LiveReload: This module contains an embedded server called LiveReload. It permits your application to automatically initiate a browser update when you make changes to a resource. It is also known as auto-refresh.
Note: You can disable LiveReload by setting the spring.devtools.livereload.enabled property to false.
Delivers browser extensions for Chrome, Firefox and Safari. By default, LiveReload is enabled. LiveReload works with the paths as mentioned below:
- /META-INF/maven
- /META-INF/resources
- /resources
- /static
- /public
- /templates
You can even disable auto-refresh in your browser by excluding the above path.
For Example:
spring.devtools.restart.exclude=public/**, static/**, templates/**
You can use the property to see other additional paths by using the property spring.devtools.restart.additional-paths.
For example:
spring.devtools.restart.additional-paths=/path-to-folder
Use the spring.devtools.restart.additional-exclude property to exclude additional paths and keep the default values.
Example:
spring.devtools.restart.additional-exclude=styles/**
There is a way to execute the trigger file in your application by adding the property spring.devtools.restart.trigger-file. The file can be internal or external.
Note:
You can only run a single LiveReload server at a time.
Make sure no additional LiveReload server is executing before launching the application.
When launching multiple applications from the IDE, exclusively the first LiveReload is kept.
Remote Debug Tunneling: Spring Boot can excavate JDWP (Java Debug Wire Protocol) straight to your application over HTTP. Even application delivery works with internet cloud providers that only expose ports 80 and 443.
Remote Update and Reboot: DevTools provides one more trick. Reinforces remote application updates and restarts. Monitor file transforms with your local classpath and compels them to the remote server. It will restart the remote server. You can also utilise this characteristic in a blend with LiveReload.
Utilising Trigger Files
Automatic restarts can restrict development time because of frequent restarts. Utilise a trigger file to fix this issue. Spring Boot monitors the trigger file and detects changes in this file. Restart the server and reload the changes made earlier.
You can implement a trigger file in your application by adding the spring.devtools.restart.triggerfile property. The file can be internal or external.
Example:
spring.devtools.restart.trigger-file=c:/workspace-sts-3.9.9.RELEASE/restart-trigger.txt
Spring Boot DevTools example
Step 1: Create a Maven project using Spring Initializr https://start.spring.io/.
Step 2: Enter the group name and artifact ID. Provided the group name com.javatpoint and the Artifact ID spring-boot-devtools-example.
Step 3: Add the following dependencies: spring-boot-starter-web and spring-boot-devtools
Step 4: Click the Generate button. Download the project JAR file.
Step 5: Extract the JAR file.
Step 6: Import the folder into STS. Importing will take some time.
Go to File -> Click Import -> Select Existing Maven Projects -> Move to Browse -> Select the folder spring-boot-devtools-example -> Finish
If the project is successfully imported, you will see the directories in the Package Explorer section of STS.
Step 7: Now, we will open the SpringBootDevtoolsExampleApplication.java and run it as a Java Application.
Then make changes to the application (edit or delete the file or code) and save those changes. When you save your changes, the server will restart to apply your changes.
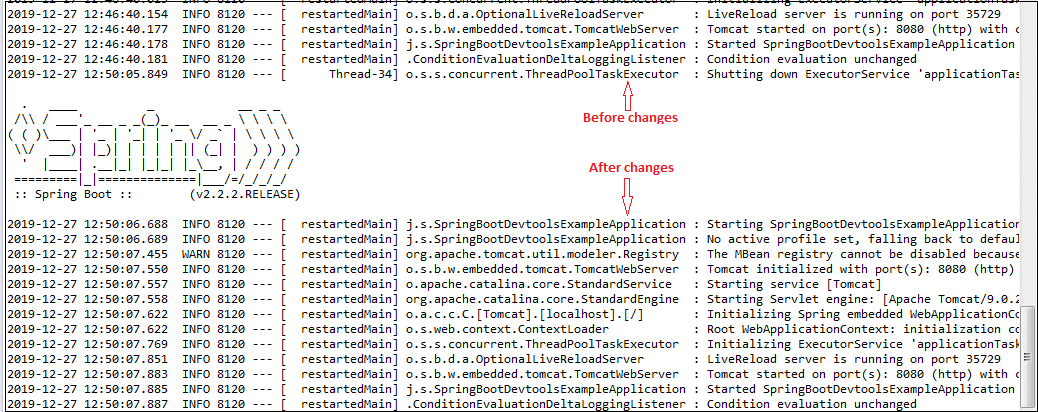