Spring Boot Starter Test
It is the main dependency of the test. It carries the bulk of the elements that are needed for testing. We already know that we have different types of tests that can test and automate the state of your application. Before you start testing, you need to integrate the testing framework.
While using Spring Boot, you have to add a starter to your project. For testing, add the spring-boot-starter-test dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<version>2.2.2.RELEASE</version>
<scope>test</scope>
</dependency>
Pulls all dependencies related to the test. Once added, you can create simple unit tests. You can build a Spring Boot project with the help of IDE or generate it using Spring Initializr.
Note: If you want to add the test dependencies manually, add them to the end of the pom.xml file.
Note that the above dependencies include the scope of the test <scope>test</scope>. All dependencies mentioned in the test scope will be disregarded if your application is bound up and ready for deployment. Test scope dependencies are only accessible when you run that in development mode and Maven test mode.
When creating a simple Spring Boot application, the pom.xml file contains the test dependencies, and the src/test/java folder contains the ApplicationNameTest.java file.
Now, we are going to create a simple maven project.
Spring Boot Starter Test Example
Step 1: Open SpringInitializr https://start.spring.io/.
Step 2: Enter the name of the Group and Artifact ID. Given group name com.tutorialandexample and Artifact as spring-boot-test-example.
Step 3: In this step, add a Spring Web dependency.
Step 4: We have to click the Generate button. Click the Generate button to download the JAR file to your local system, including all the specifications for your project.
Step 5: After completing the above steps, extract the downloaded JAR file.
Step 6: Import the folder into STS. Importing will take some time.
File --> Click on Import -->Select Existing Maven Project --> Select Folder spring-boot-test-example --> Exit
Once you import the project, it will display the project directory in the Package Explorer section of STS.
From the above directory, we can conclude that it has a test file named SpringBootTestExampleApplicationTest.java in the folder src/test/java.
package com.tutorialandexample.springboottestexample;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
class Example {
@Test
void contextLoads() {
}
}
Based on the above code, we have observed that it has implemented two annotations, @SpringBootTest and @Test, by default.
@SpringBootTest: This applies to test classes that run tests based on Spring Boot. In addition to the usual SpringTestContext Framework, it provides the following features:
- Use SpringBootContextLoader as the default ContextLoader if no special @ContextConfiguration (loader = ...) is described.
- Headily searches for @SpringBootConfiguration, if nested @Configuration is not utilized, and no explicit class is specified.
- Provides support for various Web Environment modes.
- Register the TestRestTemplate or WebTestClientBean for use in web tests that use the web server.
- Also enables application arguments to be defined with the args attribute.
Step 7: Now, in this step, open the SpringBootTestExampleApplicationTest.java and you have to run it as Junit Test as mentioned in the below image.

After running the above code, it depicts the following:
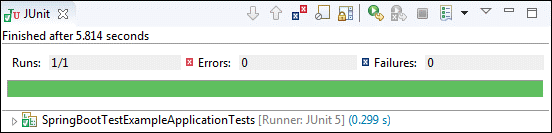
Spring Boot Production Starters
Name | Description |
spring-boot-starter-remote-shell | It is something used for the CRaSH remote shell, which monitors and manages the applications over SSH. It has been abolished since 1.5. |
spring-boot-starter-actuator | It is used for Spring Boot actuators. It can provide production-ready features that allow you to monitor and manage your applications. |
Spring Boot Technical Starters
Name | Description |
spring-boot-starter-undertow | Undertow generally uses it as a built-in Servlet container. Also the substitute to spring- boot-starter-tomcat. |
spring-boot-starter-jetty | Utilizes it as a built-in Servlet container. Also the substitute to spring- boot-starter-tomcat. |
spring-boot-starter-logging | Utilized for logging with the help of Logback. Default logging starter. |
spring-boot-starter-tomcat | Tomcat uses it as a built-in Servlet container. Spring-boot-starter has the default Servlet container launcher used by the web. |
spring-boot-starter-log4j2 | It is something utilized for Log4j2 for logging, which is an alternative to spring-boot-starter-logging. |
Spring Boot Starter Parent
We can define it as a project starter. It provides the default configuration for your application. When we talk about its use in Spring Boot, we have seen that it is utilized internally by all dependencies. Every projects of Spring Boot uses spring-boot-starter-parent as the parent of the pom.xml file.
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.4.0.RELEASE</version>
</parent>
Parent Poms allow you to manage the following for multiple child projects and modules:
- Configuration: This is an ability with the help of which you can maintain consistency of Java Version and other related properties.
- Default Java Version
- Dependency Management: It distinctively controls and be in charge of the versions of dependencies that helps to refrain from conflict.
- Helps to encode source
- Resource filtering: It's used under Maven. You can use Maven to perform variable substitutions on your project resources. If resource filtering is enabled, Maven scans the resource for property references enclosed in $ {and}.
- It is for the above projects and modules and controls the default plugin configuration.
Spring-boot-starter-parent dependency management is inherited from spring-boot dependencies. Just enter the version number of Spring Boot. In case you need more starters, you have to safely exclude the version number.
Spring Boot Starter Parent Internal
Spring Boot Starter Parent best describes Spring-boot-dependencies as parent POMs. There is a function to inherit dependency management from spring-boot -dependencies.
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>1.6.0.RELEASE</version>
<relativePath>../../spring-boot-dependencies</relativePath>
</parent>
Default Parent Pom
<properties>
<java.version>1.8</java.version>
<resource.delimiter>@</resource.delimiter>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<maven.compiler.source>${java.version}</maven.compiler.source>
<maven.compiler.target>${java.version}</maven.compiler.target>
</properties>
In the above code, the properties section defines the default values for your application. If you ask for the version, the default Java version is 1.8. In this, you can also override the Java version with the help of specifying the property <java.version>1.8</java.version> in the above project. The parent pom moreover holds up some other settings which is associated with encoding and source. The Spring Boot framework uses the mentioned default values if it is not defined in the application.properties file.
Plugin Management
With the help of plugin management, the spring-boot-starter-parent has the feature of specifying the default configuration for various plugins such as maven-fail-safe plugin, maven-jar-plugin, and maven-sure-fire-plugin. We can do it as mentioned below:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifest>
<mainClass>${start-class}</mainClass> <addDefaultImplementationEntries>true</addDefaultImplementationEntries>
</manifest>
</archive>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<includes>
<include>**/*Tests.java</include>
<include>**/*Test.java</include>
</includes>
<excludes>
<exclude>**/Abstract*.java</exclude>
</excludes>
</configuration>
</plugin>
Spring Boot Dependencies
We have already discussed before to understand the Spring Boot dependencies under this, and you must know that the spring-boot-starter-parent dependencies inherit from spring-boot dependencies and share all these properties. Therefore, Spring Boot maintains a list of dependencies as part of dependency management.
>4.1.2</httpasyncclient.version>
<httpclient.v<properties>
<activemq.version>5.13.4</activemq.version>
...
<ehcache.version>2.10.2.2.21</ehcache.version>
<ehcache3.version>3.1.1</ehcache3.version>
...
<h2.version>1.4.192</h2.version>
<hamcrest.version>1.3</hamcrest.version>
<hazelcast.version>3.6.4</hazelcast.version>
<hibernate.version>5.0.9.Final</hibernate.version>
<hibernate-validator.version>5.2.4.Final</hibernate-validator.version>
<hikaricp.version>2.4.7</hikaricp.version>
<hikaricp-java6.version>2.3.13</hikaricp-java6.version>
<hornetq.version>2.4.7.Final</hornetq.version>
<hsqldb.version>2.3.3</hsqldb.version>
<htmlunit.version>2.21</htmlunit.version>
<httpasyncclient.version ersion>4.5.2</httpclient.version>
<httpcore.version>4.4.5</httpcore.version>
<infinispan.version>8.2.2.Final</infinispan.version>
<jackson.version>2.8.1</jackson.version>
....
<jersey.version>2.23.1</jersey.version>
<jest.version>2.0.3</jest.version>
<jetty.version>9.3.11.v20160721</jetty.version>
<jetty-jsp.version>2.2.0.v201112011158</jetty-jsp.version>
<spring-security.version>4.1.1.RELEASE</spring-security.version>
<tomcat.version>8.5.4</tomcat.version>
<undertow.version>1.3.23.Final</undertow.version>
<velocity.version>1.7</velocity.version>
<velocity-tools.version>2.0</velocity-tools.version>
<webjars-hal-browser.version>9f96c74</webjars-hal-browser.version>
<webjars-locator.version>0.32</webjars-locator.version>
<wsdl4j.version>1.6.3</wsdl4j.version>
<xml-apis.version>1.4.01</xml-apis.version>
</properties>
<prerequisites>
<maven>3.2.1</maven>
</prerequisites>
Spring Boot Starter without Parent
Sometimes, you may not need to inherit spring-boot-starter-parent in your pom.xml file. To handle such use cases, Spring Boot provides the flexibility to continue to use dependency management without inheriting spring-boot-starter-parent.
< dependencyManagement>
<dependencies>
<dependency>
<!-- Import dependency management from Spring Boot -->
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.1.1.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
In the code above, you can see that we have used the tag for this. It is useful to use a different version for a particular dependency.
Spring Boot Packaging
When we talk about J2EE applications, the modules are packaged as JARs, WARs, and EARs. It is the compact folder format used by J2EE. J2EE has the three types of archives as follows:
- WAR
- JAR
- EAR
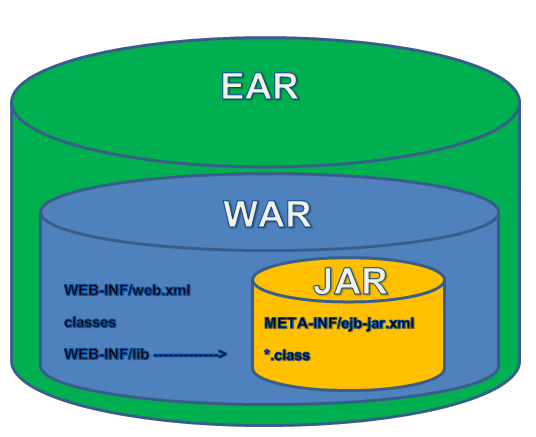
WAR
WAR can be described as Web Archiving. It represents a web application.
The web module contains Servlet classes, JSP files, Html files, javascript, etc. and is packaged as a jar file with an extension as (.war). WAR also holds up a particular directory known as WEB-INF.
The WAR module is loaded into the Java application server's web container. Java Application Server consists of two containers, one is a web container, and another is an EJB container.
The first is a web container that, hosts web applications depending on the Servlet API and JSP. It must be packaged as a WAR file for web containers. A web container is a special JAR file for the WAR file that contains the web.xmlv file in the WEB-INF folder.
Whereas EJB container hosts Enterprise Java Beans depending on the EJB API. You need to package the EJB module as a JAR file.
Note: The META-INF folder contains the ejbjar.xml file.
The pros of the WAR file are that it can be smoothly deployed to client machines in a web server environment. It would assist if you had a web server or web container to run the WAR file. For example, Weblogic, Websphere, Tomcat, etc.
JAR
JAR stands for Java Archive. Enterprise Java Beans (EJB) modules, including bean files which are class files, manifests, and Enterprise Java Beans deployment descriptors (XML files), are packaged as JAR files with the .jar extension. Software developers utilize it to allocate Java classes and various metadata.
Encapsulation of a file containing one or more Java classes manifests and descriptors is known as JAR file. Utilized in J2EE to package EJB and client-side Java applications. Easy to install.
EAR
EAR stands for Enterprise Archive. It represents an enterprise application. The above two files are packaged as JAR files with .ear extension. Deployed to the application server. You can include multiple EJB modules (JARs) and web modules (WARs). It is an unusual JAR that holds up an application.xml file in the META-INF folder.