Convert float to int Python Pandas
Python is a vast language to use for any platform. By using this language, we can convert the data types from one form to other. For these conversions, we mainly use one of the most used python libraries, Pandas.
Pandas is one of the best libraries in python used to work with data sets containing functions for analysing, cleaning, exploring, and manipulating the given data. The definition of "Pandas" has a reference to both "Panel Data" and "Python Data Analysis" and was created by Wes McKinney in 2008.
Python with Pandas is used in many fields, including academic and commercial, finance, economics, Statistics, analytics, etc.
Now let us see how we convert a float into an integer using the python Pandas library.
So, two different methods are used to convert a float into an integer. Let us discuss this with some sample codes, where we use the astype() method, and it can also be done by using apply() method.
Method 1: DataFrame.astype() method
This method will create a data frame and then use the astype() method to convert the created data type into the required one. This can method can also be used to convert many other data types. Now let us look at an example code and understand it better.
Example
# importing pandas
import pandas as pd
# create a DataFrame
list = [['ben', 10, 75.1, 54280.20],
['rach', 37, 74.31, 34280.30],
['pheebs', 32, 70.5, 84280.50],
['monica', 35, 80.3, 44280.80],
['bing', 41, 100.3, 45280.30],
['joey', 34, 72.9, 70280.25],
['ross', 38, 85.8, 25280.75]]
df = pd.DataFrame(list, columns =['Name', 'Age', 'Weight', 'Salary'])
print(df)
Output
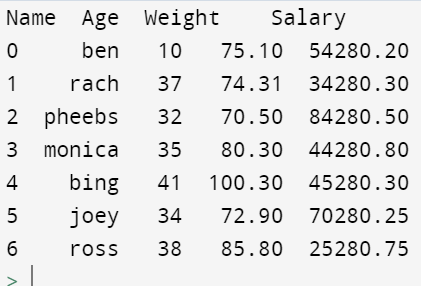
Now, as we created a data frame containing some values, we select column-wise and then convert them using the astype() method. So after the last line in the previous code, we continue to it as follows
Code continuation
# importing pandas
import pandas as pd
# create a DataFrame
list = [['ben', 10, 75.1, 54280.20],
['rach', 37, 74.31, 34280.30],
['pheebs', 32, 70.5, 84280.50],
['monica', 35, 80.3, 44280.80],
['bing', 41, 100.3, 45280.30],
['joey', 34, 72.9, 70280.25],
['ross', 38, 85.8, 25280.75]]
df = pd.DataFrame(list, columns =['Name', 'Age', 'Weight', 'Salary'])
print(df)
#display the datatypes before conversion
print(df.dtypes)
# convert weight column from float to int
df['Weight'] = df['Weight'].astype(int)
# displaying the datatypes after conversion
print(df.dtypes)
Output
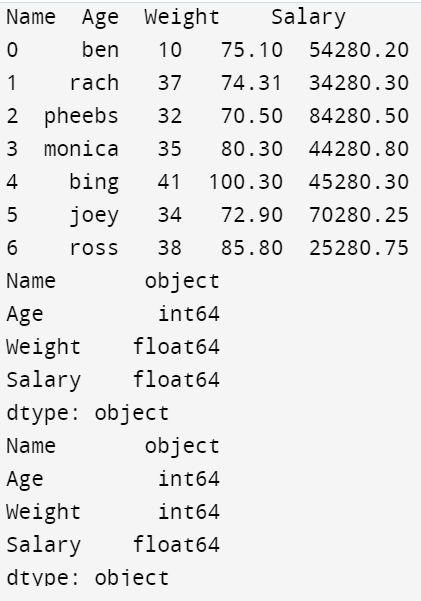
As you can see there, we converted the weight column from float to int using the astype() method. So we first created a data frame, displayed the original data types, used the astype() method, and then again printed the data types after conversion. We can also change multiple columns based on the requirement. And we got the required final output by printing the final data type display.
Now let us look at the second method for converting a float into an integer using pandas.
Method 2: DataFrame.apply() method
Even in this method, we use the apply() method to convert the present data type into the required data type. Here first create a data Frame and then convert the values into the required ones by using apply() and convert the values.
Let us understand this by using an example given below:
# importing pandas module
import pandas as pd
# create a DataFrame
list = [[10, 2.5, 10.22], [30, 6.5, 20.21],
[15, 5.6, 16.55], [35, 5.9,25.86],
[20, 6.7, 7.99], [40, 6.3, 9.25],
[25, 2.8, 11.90]]
df = pd.DataFrame(list, columns = ['Field.1', 'Field.2', 'Field.3'],
index = ['A', 'B', 'C', 'D', 'E', 'F', 'G'])
print(df)
Output
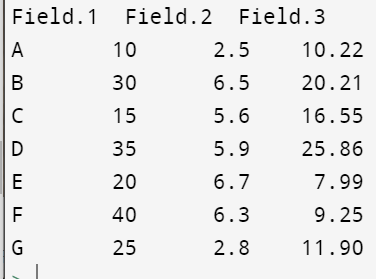
So here we created the required data set, and now we have to change the data type by the following code:
Code continuation
# importing pandas module
import pandas as pd
# importing numpy
import numpy as np
# create a DataFrame
list = [[10, 2.5, 10.22], [30, 6.5, 20.21],
[15, 5.6, 16.55], [35, 5.9,25.86],
[20, 6.7, 7.99], [40, 6.3, 9.25],
[25, 2.8, 11.90]]
df = pd.DataFrame(list, columns = ['Field.1', 'Field.2', 'Field.3'],
index = ['A', 'B', 'C', 'D', 'E', 'F', 'G'])
print(df)
# importing numpy
import numpy as np
# displaying the datatypes before
print(df.dtypes)
# converting 'Field.2' to the required type
df['Field.2'] = df['Field.2'].apply(np.int64)
# displaying the datatypes after
print(df.dtypes)
Final output
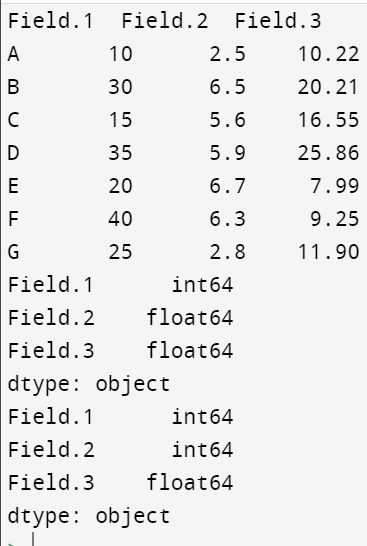
This data type got changed into an integer from a float. In the following few lines of code, we imported NumPy, which is required to manipulate the number data, and then applied the method apply(). Finally, the selected data frame got converted into the necessary datatype, in this way, not just one column. You can change multiple columns using this method. So, the output gives the complete data frame and the original data types of the particular fields and then the converted fields.
Conclusion
In these ways, you can convert float into integers using the Pandas library. This conversion gets very useful while dealing with big data.