How to Convert String to Integer in Pandas DataFrame
There are two ways to convert a String into an integer in Pandas library. Pandas is one of the best Python libraries used to work on with data sets containing functions for analyzing, cleaning, exploring, and manipulating the given data. The definition of "Pandas" has a reference to both "Panel Data" and "Python Data Analysis" and was created by Wes McKinney in 2008.
Python with Pandas is used in many fields, including academic and commercial, finance, economics, Statistics, analytics, etc.
There are two different methods to convert a string to int in pandas. So, in this article, we will learn about those methods with example codes.
First method: Series.astype() method
This method is one of the best astype() methods in python. We generally use this method to convert a data type into another datatype. In this method, the columns are taken as a data frame created by a CSV file after the data type is converted by itself. For example, a marks column may be inserted as a string, but we have to convert it into int to complete the operations, and then we use this method to convert the data.
Syntax used for this method is Series.astype(dtype,copy=True,errors=’raise’)
- dtype is where you must insert the data type to convert the series you want.
- For a copy, it makes a copy of the data frame provided.
- For Errors, this indicates the conversion of any invalid data type.
- For return, it returns the converted data type.
Let us look at an example code
# import pandas
import pandas as pd
# data frame dictionary
Data = {'Name': ['hello world','pandas'],
'Unique ID': ['500','250']}
# creating a dataframe object
df = pd.DataFrame(Data)
# converting String into an integer
df['Unique ID'] = df['Unique ID'].astype(int)
# showing the dataframe
print (df)
print("-"*25)
# showing the data types in each column
print (df.dtypes)
Output:
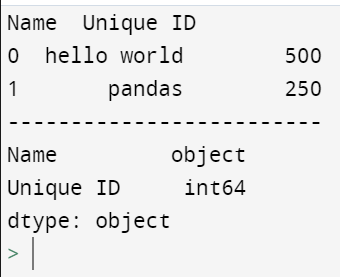
In the above example, we first imported the Pandas library and then gave the data in the form of a dictionary and made it into a data frame. As we have to convert that String into an integer, we converted it by using that ".astype()" and first entered the original data frame, and next, we gave the data frame to get converted into. Then we have to show the data frame in each column.
With this, we are done with the first method and now move on to the second one.
Second method: pandas.to_numeric() method
Pandas.to_numeric() is the primarily used method when one wants to convert an argument into an integer using pandas.
The syntax we use for this method is
“pandas.to_numeric(arg, errors=’raise’, downcast=None)”
- In the place of arg, we have to enter our String, a dictionary, or a tuple.
- For errors, we have three parameters (ignore, raise and coerce).
- If raise is used, there is an error in parsing and an exception on it.
- If ignore is used, then the invalid parsing will return the input of it.
- If coerce is used, the invalid parsing will be set as NaN.
- For Downcast, the default is always none. Instead of none, then there a data is already set to a numerical datatype downcast that results in data to be the smallest numerical datatype accordingly:
- If it's an integer or signed, then the smallest signed int datatype is resulted.
- If it's unsigned, then the smallest unsigned datatype is resulted.
- If it's a float, then the smallest float datatype is resulted.
- For Returns, the data gets successfully converted and returns the new datatype, which will depend on the given input.
Let us get this by looking at an example code
# import pandas
import pandas as pd
# dictionary
Data = {'Name': ['hello world','conversions'],
'Unique ID': ['800','500']}
# creating a dataframe object
df = pd.DataFrame(Data)
# converting an integer into a string
df['Unique ID'] = pd.to_numeric(df['Unique ID'])
# showing the dataframe
print (df)
print("-"*30)
# showing the data type of each column
print (df.dtypes)
Output
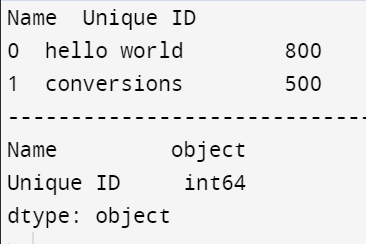
We first imported the pandas library in the above code and then inserted a dictionary containing data. Then we created a data frame object, converted that integer into a string, and printed the converted data type.
Conclusion
With this, we covered the methods where we can convert a string to an integer in the panda’s library of python.