Django and React
This article will discuss the overview of Django and React, their advantages, applications, and most importantly, how to implement react in Django.
What is Django?
Django is a free and open-source web framework, which uses Python as its programming language. It is based on Model View Template (MVT) architecture. Django is known for its rapid development, clean design, and secure websites. A framework is a collection of all the applications needed for web development. It takes care of most of the basics of web development to not have to write code from scratch every time. It was first created in 2003 by two web programmers called Adrian Holovaty and Simon Willison. It was publicly released in 2005 under the BSD license. The final version of Django was released in 2008, and a newly formed organization, Django Software Foundation, was announced to maintain Django in the future.
Pros and Cons
Pros of Django
- It is swift, there are already very helpful frameworks, and it can reduce the development time.
- It provides very secure websites which are protected from different attacks such as SQL injections etc.
- Django has an outstanding community and documentation that can help developers.
- One of the significant advantages of Django is that it is immensely scalable and can handle the traffic of more than 350 million users. It also provides very cheap hosting.
Cons of Django
- In Django, if we have to specify URLs, then we have to use Regex for that. It can easily become very complex and complicated.
- Django has got a substantial structure and too many functionalities for a minor project. It is an intensive coding framework and requires a lot of processing time. So if the project is not that big, think about it before doing all the hard work.
- Django has a major disadvantage of processing only one request at a time which makes it hard for developers to send API requests.
- Django has a monolithic framework. All the modules in Django have to be compatible backward, which slows down the evolution.
What is React?
ReactJS is used to build UI components. It is a JavaScript library. React is a component-based library, and just like Django, it is also.
-source. React is not a framework but just a library. React can be considered as V, i.e., View for MVC (Model-View-Control) architecture. React gives better performance by changing the Virtual DOM instead of the actual DOM. Reacts follow the one-way data flow. React is heavily used to create large apps because of its components structure.
Pros and Features
- Beginner-friendly:ReactJS is considered to be very beginner-friendly and easy to use. React uses the JSX syntax, which is very similar to JS and HTML. Since React is an open-source library, constant improvement and modifications are done to it regularly.
- Virtual DOM: The virtual DOM is more efficient than normal DOM, and also it is faster. The problem with the real DOM is that when even a small update is done in a UI component, the whole DOM tree is rewritten. This process consumes time and is not efficient. On the other hand, the virtual DOM just updates the component, which is updated by the user, unlike real DOM, which updates the whole tree. Modern websites have a really large DOM tree, so using the virtual DOM is much more efficient.
- Reusable Components: React uses the component structure to build applications. These components can be re-used to build multiple UI and pages for the application. This reusability reduces the production time for developing the application.
- One-way data flow: Unlike other JavaScript applications, React uses the one-directional data flow. In the multi-directional data flow, when a child component is changed, it also changes its parent components which are not ideal and can be hard to debug. React resolves this problem by providing a one-way data flow so the parent component cannot change after changing the child component.
Cons:
- React is the View part of the MVC architecture. So to use React, we still have to find the Model and Controller for the View.
- Documentation is one of the cons of React. React is so fast and quickly developing that its documentation is lagging. The documentation is not friendly for beginners and not extensive.
- React JSX is not considered to be good by some of the React community members. JSX has a syntax that is a mixture of HTML and JavaScript. It also has some advantages to it.
Implementing React with Django
We will want to connect a frontend to the backend to do CURD or similar operations or fetch the database.
First, we will set up our application inside a virtual environment.
virtualenvnewenv
cdnewev
source bin/activate
django-admin.pystartprojectmyproject
cdmyproject
Django-adminstartapp core
Now let's define our model in our models.py file. We will store the name and detail of the student.
Models.py
fromDjango.db import models
# Create your models here.
classStudent(models.Model):
name = models.CharField(max_length=30)
detail = models.CharField(max_length=500)
Now connect the model to the database through the migrations. To connect to the database, run the below command in the terminal:
python manage.py makemigrations
python manage.py migrate
The later command applies the change to the database.
The next step is to define the serializers. Serialization is the act of changing the object into another data format. They are used to render the data in Python easily.
fromrest_framework import serializers
from .models import *
classSerializer(serializers.ModelSerializer):
class Meta:
model = Student
fields = ['name', 'detail']
Now let us create the views.py for the GET, PUT, POST, and DELETE operations. Copy the following code in core/views.py:
fromDjango.shortcuts import render
fromrest_framework.views import APIView
from .models import *
fromrest_framework.response import Response
from .serializer import *
# Create your views here.
classStudent_View(APIView):
ser_class = Serializer
def get(self, request):
detail = [ {"name": detail.name,"detail": detail.detail}
for detail in Students.objects.all()]
return Response(detail)
def post(self, request):
serializer = Serializer(data=request.data)
ifserializer.is_valid(raise_exception=True):
serializer.save()
return Response(serializer.data)
Now use the following code in urls.py:
fromDjango.contrib import admin
fromDjango.urls import path
from students import views
fromDjango.conf.urls import url
urlpatterns = [
path('admin/', admin.site.urls),
path(‘std/’,Student_View.as_view()),
]
Now just make some changes in settings.py.
Add the app coreto the INSTALLED_APPS.
Install the cors middleware and add it to the MIDDLEWARE section.
Now just run the server by using the below command:
After successfully running the server, go to the localhost:8000/std, and you will see something like this:
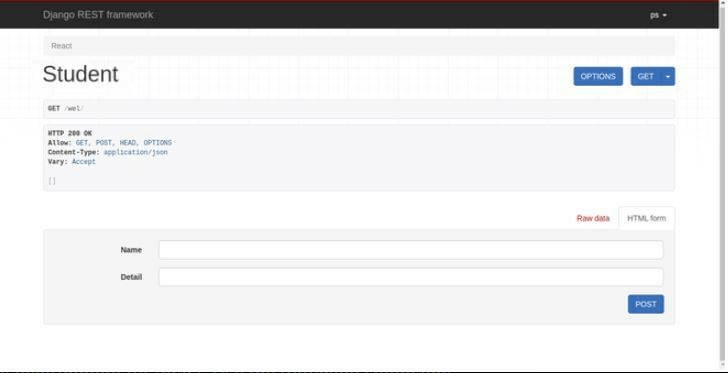
Building React App
Let us set up the react project by these commands:
Npx create-react-app studentslist
Cd students list
Axios is used to connect the frontend and backend.
After creating the project you will see a App.js file in your main directory, copy the following code in app.js :
import React from 'react';
class App extends React.Component {
render() {
return(
<div>
<h1>Student List</h1>
/div>);
}
}
export default App;
Now we have to fetch the data every time we render the react app. We do this by using Axios.
import React from 'react';
importaxios from 'axios';
class App extends React.Component {
state = {
details : [],
}
componentDidMount() {
vardata ;
axios.get('http://localhost:8000/std/')
.then(res => {
data = res.data;
this.setState({
details : data
});
})
.catch(err => {})
}
render() {
return(
<div>
{this.state.details.map((detail, id) => (
<div key={id}>
<div> <h1>{detail.detail} </h1>
<div class = “text”>
---
{detail.name}
</div>
</div>
</div>
)
}
</div>
);
}
}
export default App;
Now add some data to the student model and run the react server by running this command in command prompt and browse to the localhost:300 in your browser.
Npm start
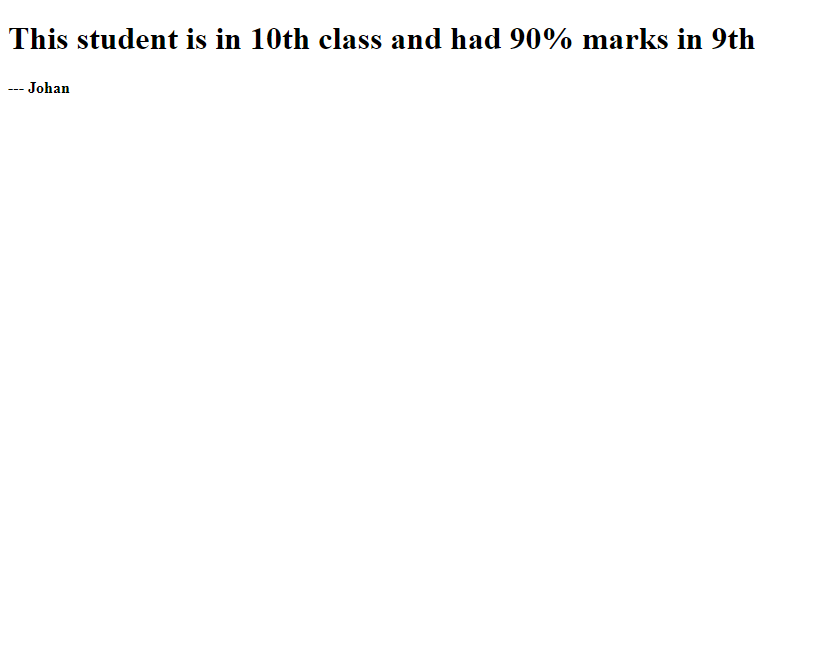
This is just the beginning, and you can expand this application by adding many features to it, like a form for the user to add some new students to the model.