Django REST Framework
Django REST Framework (DRF)is an open-source, powerful, and flexible toolkit used to build RESTful web APIs. It is built upon the Django framework. DRF increases the development speed. Django and some basic knowledge of REST is a prerequisite. DRF allows the developer to define the URL structure. It includes a built-in API browser for testing newly developed API.
DRF built API is rich in features like a wide range of media type support, authentication and permission policies. For more complex functionality, standard function-based views or granular with powerful class-based views are used.
API
API stands for Application Programming Interface, allows two different applications to communicate, and acts as a mediator. For Web applications, communication takes place over a network or internet. This makes developers easy to certain technologies by providing predefined functionality. The API receives data usually in JSON format from other application and provide to the backend.
RESTful API
REST stands for Representational State Transfer. It is an architecture Web applications are developed on. Web services using REST architecture are called RESTful Web Services. RESTful API acts as a translator between two machines communicating over a Web Service through HTTP. URLs(endpoints) define the structure of API and how a user will access data from an application using HTTP methods like GET, POST, PUT, DELETE. RESTful API returns JSON files that a variety of devices can interpret. Ex., Facebook API, Google API, etc. are REST APIs
Create an API using DRF
Step 1:- Create a Django project and run the following command after moving inside the project directory
python manage.py startapp first_api
Step 2:- Install the Django REST Framework by executing the command below
pip install djangorestframework==3.10
Step 3:- Install rest_framework and basic_api inside the variable name INSTALLED_APPS the settings.py file.
Step 4:- Create models.py
from django.db import models
Rate = [
('excellent', 2),
('good', 1),
('bad',0 )
]
class DRFPost(models.Model):
name = models.CharField(max_length = 50)
author = models.CharField(max_length = 50)
rating = models.CharField(choices = Rate, default = 'good', max_length = 50)
def __str__(self):
return self.name
Step 5:- Register model with Django-admin admin.py.
from django.contrib import admin
from first_api.models import DRFPost
# DataFlair
admin.site.register(DRFPost)
Step 6 :- Run server
python manage.py runserver
Step 7:- Create the 3 layers of DRF API
Basic Architecture
The Django REST Framework API consists of 3 layers:
- Serializer
It converts the information stored in the databases or Django Model instances as complex structures into formats like JSON or XML, which API can easily transmit. Whenever a user submits a piece of information through the API serializer receives that data and then validates it and converts it into a form that can be put into a Model instance. Likewise, when a user accesses a piece of information via API, the instance is fed to the serializer, which parses them into JSON format to the user. The following code is saved as serializer.py in the api directory of the project
from rest_framework import serializer
from first_api.models import DRFPost
class DRFPostSerializer(serializer.ModelSerializer):
class Meta:
model = DRFPost
fields = (‘name’, )
Serializer class is imported from the rest_framework, which is the DRF used.
The serializer class is created, which has model and field attributes. The model will specify the model for the serializer. Fields specify which fields are accessible using the serializer. Instead of field exclude can be set which include all model except the model specified.
- ViewSets
It is where available operations like Read, Create, Update, Delete are defined via API. The ModelViewSet has the following in-built operations:
- Create instance – create()
- Retrieve instance – retrieve()
- Update instance – update() or partial_update()
- Destroy instance – destroy()
- List instance – list()
The following code is saved as views.py in the api directory of the project
from first_api.serializer import DRFPostSerializer
from first_api.models import DRFPost
class DRFViewSet(viewsets.ModelViewSet):
queryset = DRFPost.objects.all()
serializer_class = DRFPostSerializer
We will import the model and serializer we created. We created ViewSet class which contains queryset and serialozer_class attributes. The queryset provides the model instance to operate on. In the serializer_class, we specify the serializer class to create a JSON model.
For customization, we can use generic viewsets, which will provide us with the classes which will make it easy to deal with RESTful requests and send JSON data.
- Routers
Provides URL that will provide access to each viewsets.
from django.urls import path
from rest_framework.urlpatterns import format_suffix_patterns
from first_api import views
router = routers.DefaultRouter()
router.register(r’drf’, first_api.DRFViewSet)
urlpatterns = [
path('basic/', views.DRFVieSet.as_view()),
path('basic/<int:pk>/', views.DRFViewSet.as_view()),
]
urlpatterns = format_suffix_patterns(urlpatterns)
The format_suffix_patters will generalize our urls. The views we created is imported. In view function name of the class is written accompanied by as_view().
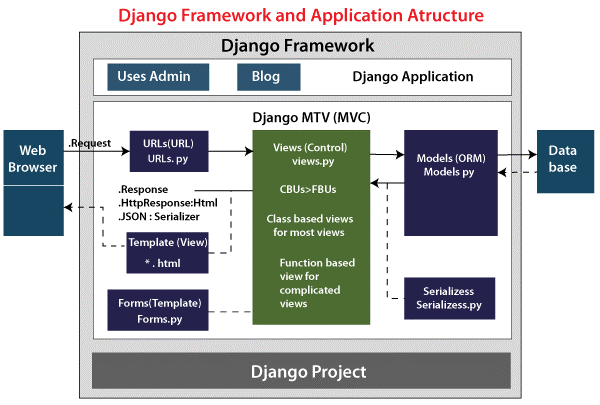
Advantages of REST Framework
- Simplicity
- Flexibility
- Serialization compatible with ORM and non-ORM data source
- Easy to customize
- Classed based Views are cleaner and simpler
- Pluggable authentication like OAuth1, OAuth2, etc.
- HTTP response handling
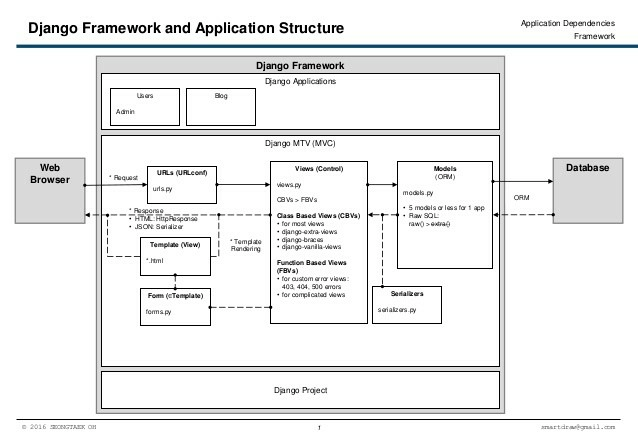