Struts 2 Database Access
The database can be connected with struts applications to store and retrieve data using JDBC.
Struts Database Access example:
Create the database and create the table in it:
Create database stuser; // create the database with any name.
use stuser; // select the created database.
Create table strutsuser(Name varchar(20), Password varchar(20), Email varchar(20), Gender varchar(20), Country varchar(20)); // Create the table in database.
desc strutsuser; // desc command is used to check table structure.
To get input from the user create the index.jsp file:
The index.jsp page takes the input from the user. The user will enter the name, password, email, gender, and country. Then after clicking on the Submit button, it will redirect to the next resource.
<%@ taglib uri="/struts-tags" prefix="s" %>
<s:form action="register">
<s:textfield name="name" label="Enter the UserName"> </s:textfield>
<s:password name="password" label="Enter the Password"> </s:password>
<s:textfield name="email" label="Enter the Email"> </s:textfield>
<s:radio list="{'male','female'}" name="gender"> </s:radio>
<s:select cssStyle="width:155px;" list="{'India','England','UK','Bhutan'}" name="country" label="Country"> </s:select>
<s:submit value="Register"> </s:submit>
</s:form>
Create success.jsp file to denote success:
The success.jsp page is used to display Welcome message when the method returns the status value greater than 0.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib uri="/struts-tags" prefix="s" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
Welcome
<s:property value="name"/>
</body>
</html>
Create error.jsp file to denote failure:
The error.jsp page is used to display error message when the method returns the status value less than 0.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Error page</title>
</head>
<body>
Sorry wrong password
</body>
</html>
Create the class to establish a connection with the database:
Using JDBC establish a connection with the database
import java.sql.*;
public class RegisterDao {
public static int save (RegisterAction r)
{
int status = 0;
try
{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection("jdbc:mysql://localhost:3306/stuser","root","");
PreparedStatement ps=con.prepareStatement("insert into strutsuser values(?,?,?,?,?)");
ps.setString (1,r.getName());
ps.setString (2,r.getPassword());
ps.setString (3,r.getEmail());
ps.setString (4,r.getGender());
ps.setString (5,r.getCountry());
status = ps.executeUpdate();
}
catch (Exception e)
{
e.printStackTrace();
}
return status;
}
}
Create the action class RegisterAction.java:
The Action class RegisterAction.java contains name, password, email, gender, country fields with their getter and setters. It contains the execute method. On success, it will go to the success.jsp page and on error, it will redirect to error.jsp page.
public class RegisterAction
{
private String name, password, email, gender, country;
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public String getPassword()
{
return password;
}
public void setPassword(String password)
{
this.password = password;
}
public String getEmail()
{
return email;
}
public void setEmail(String email)
{
this.email = email;
}
public String getGender()
{
return gender;
}
public void setGender(String gender)
{
this.gender = gender;
}
public String getCountry()
{
return country;
}
public void setCountry(String country)
{
this.country = country;
}
public String execute()
{
int i=RegisterDao.save(this);
if(i>0)
{
return "success";
}
return "error";
}
}
Construct struts.xml file:
In the struts.xml file, make the entry of the action class and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and error.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts
Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<package name="default" extends="struts-default">
<action name="register" class="RegisterAction">
<result name="success">success.jsp</result>
<result name="error">error.jsp</result>
</action>
</package>
</struts>
web.xml file is created inside WEB-INF folder in WebContent folder:
The web.xml file in the WEB-INF folder specify how elements are processed. The entry of FilterDispatcher is done in the web.xml file. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>StrutsDatabase </display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. It will show a label for entering the name, password, email, gender, and country and the Register button.
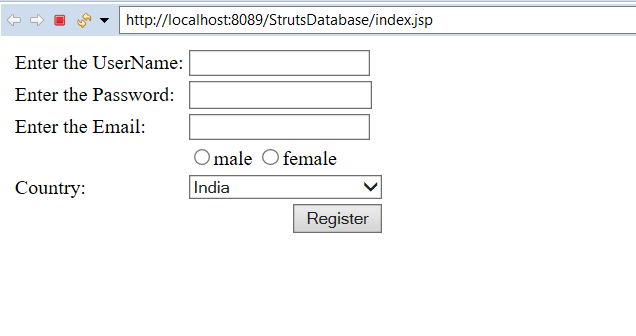
Enter the username, password, email, gender, and country, and click on the register button.
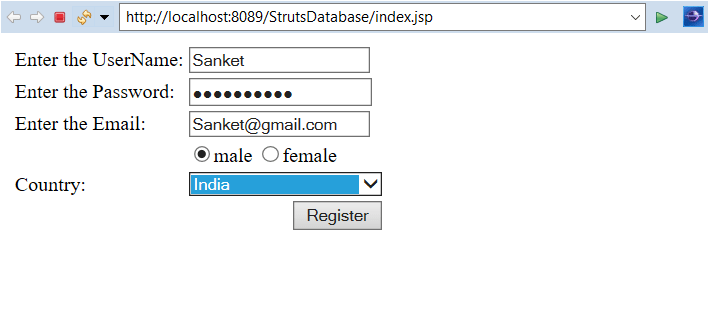
As the username, password, email, gender, and the country is entered correctly it will redirect to success.jsp displaying the welcome message.
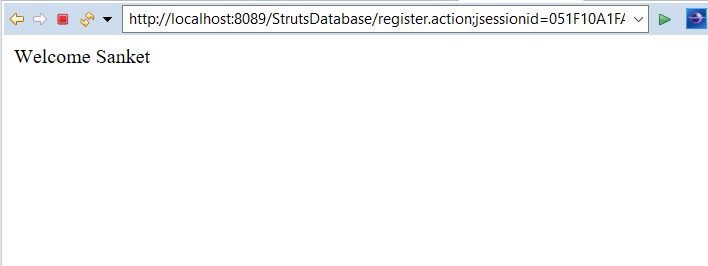