Struts 2 url tag
The url tag in struts is used to create url as a text. It creates url as a text string.
Struts Data tags- url tag Example:
Create the index.jsp file showing the use of url tag:
The index.jsp page displays Struts 2 URL tag example. It also shows the list of items. The first item shows the image url as the image link is specified. The second item shows the link to google as the url is http://www.google.com. The third item creates an action url with the id parameter.
<%@ page language= "java" contentType= "text/html; charset= ISO-8859-1"
pageEncoding= "ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head> <title> Index page </title>
</head>
<body>
<h2> Struts 2 URL tag example </h2>
<ol>
<li>
<img src= "<s:url value= "/images/flower.jpg"/>" />
</li>
<li>
<a href= "<s:url value="http://www.google.com" />" target= "_blank"> Google </a>
</li>
<li>
<s:url action="url.action" >
<s:param name="id"> 1001 </s:param>
</s:url>
</li>
</ol>
</body>
</html>
Create error.jsp file to denote failure:
The error.jsp page will display Sorry something went wrong !!! if something went wrong.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv= "Content-Type" content= "text/html; charset= ISO-8859-1">
<title> Error page </title>
</head>
<body>
Sorry something went wrong !!!
</body>
</html>
Create the class to include execute method:
The URLTag class extends ActionSupport class and include execute() method. This method will return a success string on successful execution.
import com.opensymphony.xwork2.ActionSupport;
public class URLTag extends ActionSupport
{
public String execute()
{
return SUCCESS;
}
}
Construct struts.xml file:
In the struts.xml file, make the entry of the action class and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and error. On success it will redirect to index.jsp and on error it will redirect to error.jsp.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" namespace="/" extends="struts-default">
<action name="url"
class="URLTag" >
<result name="success"> /index.jsp </result>
<result name="error"> /error.jsp </result>
</action>
</package>
</struts>
web.xml file is created inside WEB-INF folder in WebContent folder :
The web.xml file in the WEB-INF folder specify how elements are processed. The entry of FilterDispatcher or StrutsPrepareAndExecuteFilter is done in the web.xml file. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name> StrutsURL </display-name>
<welcome-file-list>
<welcome-file> index.html </welcome-file>
<welcome-file> index.htm </welcome-file>
<welcome-file> index.jsp </welcome-file>
<welcome-file> default.html </welcome-file>
<welcome-file> default.htm </welcome-file>
<welcome-file> default.jsp </welcome-file>
</welcome-file-list>
<filter>
<filter-name> struts2 </filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name> struts2 </filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. After that run the url, http://localhost:8080/StrutsURL/url.action. It will show the content of the index.jsp page, Struts URL tag example and show flower image as the url mentioned is <img src= "<s:url value= "/images/flower.jpg"/>" /> Second item will display link to www.google.com as url specified is <a href= "<s:url value="http://www.google.com" />" target= "_blank"> Google </a>. Third item display /StrutsURL/url.action?id=1001 as url action mentioned is url.action and using param tag id is set to 1001.
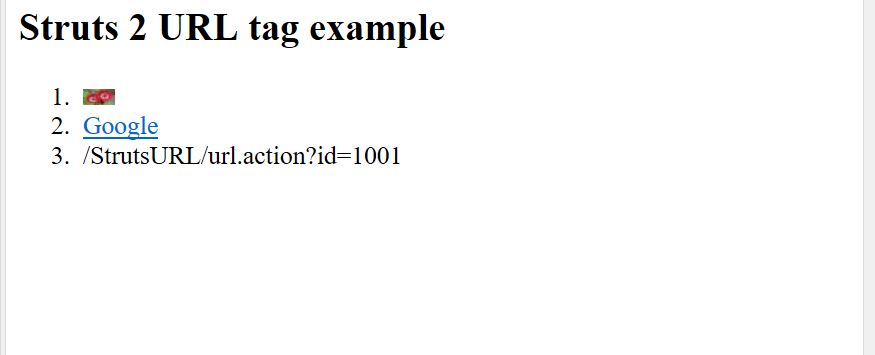