Struts 2 Form tag
In struts, web application various UI elements tag are used to create forms like HTML. Various struts UI tags like form tag, textfield tag, password tag, textarea tat, checkbox tag, and select tag as well as the radio tag are used to create forms to take input from the user. These tags are helpful when the user needs to give any input and it is to be retrieved later.
Struts Forms tag- Simple UI tags Example:
Create the index.jsp file showing the use of form tags like textfield, textarea, password, checkbox, select, and radio:
The index.jsp file displays the heading, Struts 2 form tag example. It contains one form with action to welcome containing textfield for name, password field for entering the password, textarea for entering long address and checkbox to check whether an update is received or not, select list to select the position of the person and radio button to select the gender. This page is used to take input from the user. All the employee information is then displayed with the help of the next jsp page.
<%@ page language= "java" contentType= "text/html; charset= ISO-8859-1"
pageEncoding= "ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head> <title> Index Page to take input from the user </title>
</head>
<body>
<h2> Struts 2 form tag example </h2>
<s:form action= "welcome" namespace= "/" >
<s:textfield name= "name" label= "User Name: " >
</s:textfield>
<s:password name= "password" label= "Password: " >
</s:password>
<s:textarea name= "addr" label= "Address: " cols= "20" rows= "3" wrap= "true">
</s:textarea>
<s:checkbox name= "receiveUpdates" fieldValue= "true" label= "Do you received updates: ">
</s:checkbox>
<s:select list= "{'Developer', ‘Team Lead’ , ‘Manager’}" name= "rolesSelect" multiple= "true">
</s:select>
<s:radio list= "{‘Male’, 'Female’}" name= " gender " multiple= " true ">
</s:radio>
<s:submit value="Submit">
</s:submit>
</s:form>
</body>
</html>
Create welcome.jsp page to display the result:
The welcome.jsp file is used to display name, password, gender, position, address of the employee entered by them. Using property tag in struts and value attribute it display value of each field.
<%@ page language= "java" contentType= "text/html; charset=US-ASCII" pageEncoding="US-ASCII"%> <%@ taglib uri="/struts-tags" prefix="s" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "https://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv= "Content-Type" content= "text/html; charset= US-ASCII">
<title> Welcome Page to show employee information </title>
</head>
<body>
<h3> Employees: </h3>
User Name of Employee: <s:property value="name">
</s:property>
<br>
<s:hidden name= "password">
</s:hidden>
Address: <s:property value= "addr">
</s:property>
<br>
Updates receiving status: <s:property value= "receiveUpdates">
</s:property>
<br> Position of person: <s:property value= "rolesSelect">
</s:property>
<br>
Gender: <s:property value= "gender">
</s:property>
<h4> Thank You </h4>
</body>
</html>
Create error.jsp file to denote failure:
The error.jsp page will display Sorry something went wrong !!! if something went wrong.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv= "Content-Type" content= "text/html; charset= ISO-8859-1">
<title> Error page to show error if occurs </title>
</head>
<body>
Sorry something went wrong !!!
</body>
</html>
Create the class to include execute method:
The FormTag class extends ActionSupport class and include execute() method. This method will return a success string on successful execution. It creates an object of the User class and use it to set and retrieve fields.
import com.opensymphony.xwork2.ActionSupport;
import com.opensymphony.xwork2.ModelDriven;
public class FormTag extends ActionSupport implements ModelDriven<User>
{
@Override
public String execute()
{
return SUCCESS;
}
private User user = new User();
@Override
public User getModel()
{
return user;
}
public User getUser()
{
return user;
}
public void setUser(User user)
{
this.user = user;
}
Construct User.java class to include getters and setters of all fields:
The user.java class contains name, password, address, receiveupdates, role, gender fields, and their getters and setters to set and retrieve the values. This is the bean class called in the action class to get one user object ready with all its value set.
public class User
{
private String name;
private String password;
private String addr;
private boolean receiveUpdates;
private String[] rolesSelect;
private String gender;
public String getName()
{
return name;
}
public void setName (String name)
{
this.name = name;
}
public String getPassword()
{
return password;
}
public void setPassword (String password)
{
this.password = password;
}
public String getAddr()
{
return addr;
}
public void setAddr (String addr)
{
this.addr = addr;
}
public boolean isReceiveUpdates()
{
return receiveUpdates;
}
public void setReceiveUpdates (boolean receiveUpdates)
{
this.receiveUpdates = receiveUpdates;
}
public String[] getRolesSelect()
{
return rolesSelect;
}
public void setRolesSelect (String[] rolesSelect)
{
this.rolesSelect = rolesSelect;
}
public String getGender()
{
return gender;
}
public void setGender (String gender)
{
this.gender = gender;
}
}
Construct struts.xml file:
In the struts.xml file, make the entry of the action class and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and error. On success it will redirect to welcome.jsp and on error it will redirect to error.jsp.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<constant name= "struts.convention.result.path" value= "/">
</constant>
<package name= " user " namespace= "/" extends= "struts-default">
<action name= " welcome " class= "FormTag">
<result name= " success "> /welcome.jsp </result>
<result name= " error "> /error.jsp </result>
</action>
</package>
</struts>
web.xml file is created inside WEB-INF folder in WebContent folder:
The web.xml file in the WEB-INF folder specify how elements are processed. The entry of FilterDispatcher or StrutsPrepareAndExecuteFilter is done in the web.xml file. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name> StrutsForm </display-name>
<welcome-file-list>
<welcome-file> index.html </welcome-file>
<welcome-file> index.htm </welcome-file>
<welcome-file> index.jsp </welcome-file>
<welcome-file> default.html </welcome-file>
<welcome-file> default.htm </welcome-file>
<welcome-file> default.jsp </welcome-file>
</welcome-file-list>
<filter>
<filter-name> struts2 </filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name> struts2 </filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. It will display index.jsp page result. This page display heading saying Struts form tag example. It contains one form with a label to enter the user name, password, and address of the employee. It asks the user whether the update is received or not, user can check or uncheck the checkbox. Then the select list is displayed with various roles for the employee. Users have to select one of the role Developer, Team lead, or manager. Then radio button is used to get the gender of the employee. All These elements are created with the help of form tags in struts. At the last of the form, one submit button is created. On clicking on submit button either it will redirect to welcome.jsp on success or error.jsp is something wents wrong. In the User class, all the values entered by the user are set to the fields. Using property tag with the value attribute, they can be retrieved further.
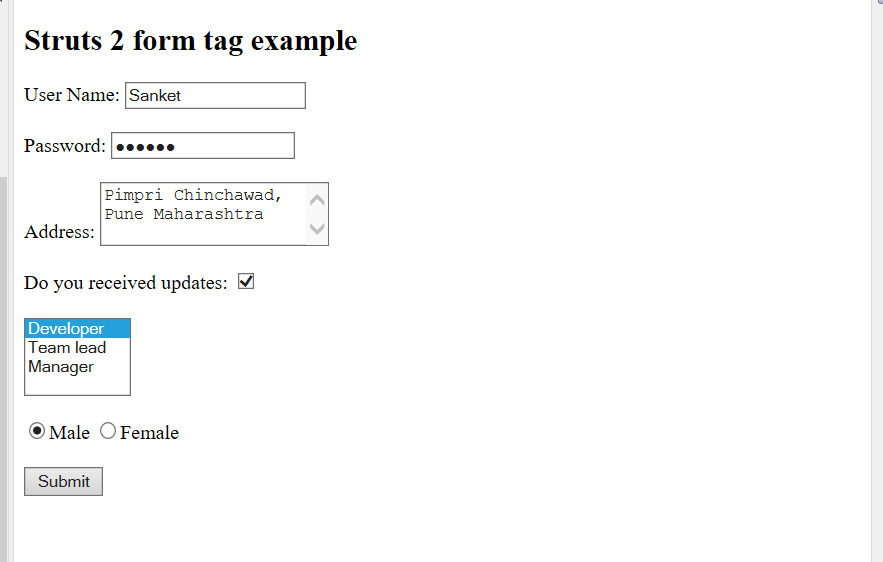
When execute method return success string, welcome.jsp page will get executed. When the user clicks on the submit button after entering all values, All the field values are set using the User Bean class with the help of setters. The welcome.jsp file displays username, address, updates receiving status, Position of person and gender of an employee using property tag and value attribute in struts web application. Lastly, it displays Thank you message.
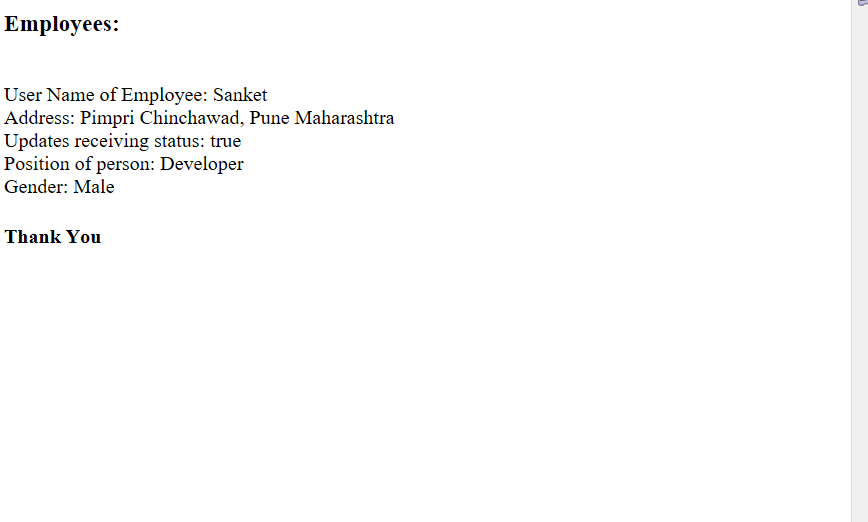