Struts 2 param tag
The param tag in struts is used to set values of the variable in other tags like include and bean tag. It is used to parametrize other tags. Struts param tag is not only used with bean tag but it is used with many tags to set parameter values.
Struts Data tags- Param tag Example:
Create the index.jsp file as first jsp file:
The index.jsp page is created to set values using param tag. It will display the heading Struts 2 param tag example. It will first set the value of the fruit name in the Fruit bean class. Then it will set name, age, and favorite fruit values for Person bean class. Then using the list it will print the name of the person, age of the person, and favorite fruit of that person using the value attribute.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title> Index page </title>
</head>
<body>
<h3> Struts 2 param tag example </h3>
<s:bean name="Fruit" var="fruitBean">
<s:param name=" fruitName "> Mango </s:param>
</s:bean>
<s:bean name="Person" var="personBean">
<s:param name="name"> Sanket </s:param>
<s:param name="age"> 19 </s:param>
<s:param name="fruit" value="#fruitBean"></s:param>
</s:bean>
<h2> Person Information</h2>
<ol>
<li>Name of the Person : <s:property value="#personBean.name" /> </li>
<li>Age of the person : <s:property value="#personBean.age" /> </li>
<li>Favorite Fruit : <s:property value="#personBean.fruit" /> </li>
</ol>
</body>
</html>
Create the class to include execute method:
ParamTag class is used to include execute() method which will return a success string if everything is executed with no error.
import com.opensymphony.xwork2.ActionSupport;
public class ParamTag extends ActionSupport
{
public String execute()
{
return SUCCESS;
}
}
Create Bean class Person:
The bean class person is created with a name, age, and fruit fields in it. Getters and setters are included in this class to set and retrieve values. Also, the getter for getting fruit name is included in this class.
public class Person
{
private String name;
private int age;
private Fruit fruit;
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public int getAge()
{
return age;
}
public void setAge(int age)
{
this.age = age;
}
public Fruit getFruit()
{
return fruit;
}
public void setFruit(Fruit fruit)
{
this.fruit = fruit;
}
public String getFruitName()
{
return this.fruit.getFruitName();
}
}
Create Bean class Fruit:
The bean class for fruit is created to set and retrieve fruit name values.
public class Fruit
{
private String fruitName;
public String getFruitName() {
return fruitName;
}
public void setFruitName(String fruitName) {
this.fruitName = fruitName;
}
}
Construct struts.xml file:
In the struts.xml file, make the entry of the action class and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and error. On success it will redirect to index.jsp and on error it will redirect to error.jsp.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" namespace="/" extends="struts-default">
<action name="paramAction"
class="ParamTag" >
<result name="success"> /index.jsp </result>
<result name="error"> /error.jsp </result>
</action>
</package>
</struts>
web.xml file is created inside WEB-INF folder in WebContent folder:
The web.xml file in the WEB-INF folder specify how elements are processed. The entry of FilterDispatcher or StrutsPrepareAndExecuteFilter is done in the web.xml file. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name> StrutsParam </display-name>
<welcome-file-list>
<welcome-file> index.html </welcome-file>
<welcome-file> index.htm </welcome-file>
<welcome-file> index.jsp </welcome-file>
<welcome-file> default.html </welcome-file>
<welcome-file> default.htm </welcome-file>
<welcome-file> default.jsp </welcome-file>
</welcome-file-list>
<filter>
<filter-name> struts2 </filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name> struts2 </filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. After that run the url, http://localhost:8080/StrutsParam/paramAction.action. It will show the content of the index.jsp page, Struts 2 param tag example and Person Information. It will show name of the person, age of the person and favourite fruit. Using Fruit bean, we have set the fruit name and then we have set name, age and fruit for the person. Then using value attribute of property tag, we have retrieved and printed values for name, age and favourite fruit of the person.
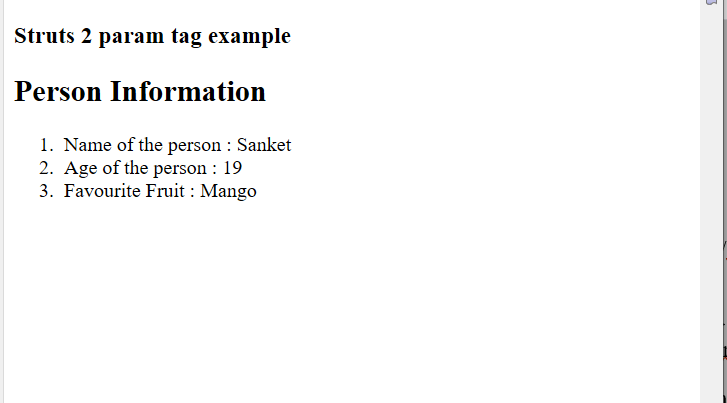