Struts 2 push tag
Struts Data tags- Push tag Example:
The push tag in struts2 is used to set the value and maintain it on top of the stack. It makes the value easier to push and access.
Create the index.jsp file showing the use of push tag:
The index.jsp file displays the heading struts push tag example. Then it displays Setting the value using bean tag in struts: It uses bean tag and using the var attribute with the help of the property tag the first name and the last name of the programmer is displayed. Also using the push tag with the person bean the value for first name and last name is displayed by using the property tag.
<%@ page language= "java" contentType= "text/html; charset= ISO-8859-1"
pageEncoding= "ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head> <title> Index page </title>
</head>
<body>
<h2> Struts 2 push tag example </h2>
<h2> Setting the value using bean tag in struts: </h2>
<s:bean name="Person" var="personBean" />
First name of the programmer : <s:property value="#personBean.firstName" />
<br/>
Last name of the programmer : <s:property value="#personBean.lastName" />
<br/>
<h2> Setting the value using push tag in struts: </h2>
<s:push value="#personBean" >
First name of the programmer : <s:property value="firstName" />
<br/>
Last name of the programmer : <s:property value="lastName" />
<br/>
</s:push>
</body>
</html>
Create error.jsp file to denote failure:
The error.jsp page will display Sorry something went wrong !!! if something went wrong.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title> Error page </title>
</head>
<body>
Sorry something went wrong !!!
</body>
</html>
Create the class to include execute method:
The PushTag class is created to include execute method. The execute method will return the success string if all the things are executed properly.
import com.opensymphony.xwork2.ActionSupport;
public class PushTag extends ActionSupport
{
public String execute() throws Exception
{
return SUCCESS;
}
}
Create Bean class Person:
The Person bean class is created with two fields first name and last name. For the first name and last name, getters are created to retrieve the values.
public class Person
{
private String firstName = " Sanket ";
private String lastName = " Chaudhari ";
public String getFirstName()
{
return firstName;
}
public String getLastName()
{
return lastName;
}
}
Construct struts.xml file:
In the struts.xml file, make the entry of the action class and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and error. On success it will redirect to index.jsp and on error it will redirect to error.jsp.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<constant name="struts.devMode" value="true" />
<package name="default" namespace="/" extends="struts-default">
<action name="push"
class="PushTag" >
<result name="success"> /index.jsp </result>
<result name="error"> /error.jsp </result>
</action>
</package>
</struts>
web.xml file is created inside WEB-INF folder in WebContent folder:
The web.xml file in the WEB-INF folder specify how elements are processed. The entry of FilterDispatcher or StrutsPrepareAndExecuteFilter is done in the web.xml file. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name> StrutsPush </display-name>
<welcome-file-list>
<welcome-file> index.html </welcome-file>
<welcome-file> index.htm </welcome-file>
<welcome-file> index.jsp </welcome-file>
<welcome-file> default.html </welcome-file>
<welcome-file> default.htm </welcome-file>
<welcome-file> default.jsp </welcome-file>
</welcome-file-list>
<filter>
<filter-name> struts2 </filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name> struts2 </filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. After that run the url, http://localhost:8080/StrutsPush/push.action. It will show the content of the index.jsp page, Struts 2 push tag example. Then it displays Setting the value using bean tag in struts and displays the first name and last name set using bean tag. It will show output as, the First name of the programmer : Sanket
Last name of the programmer : Chaudhari
Then we are Setting the value using the push tag in struts. After setting the value with push tag it displays,
First name of the programmer : Sanket
Last name of the programmer : Chaudhari
Hence it displays the same output in the case of bean and push tag in struts. We can easily use push tag with a property tag to set and retrieve values.
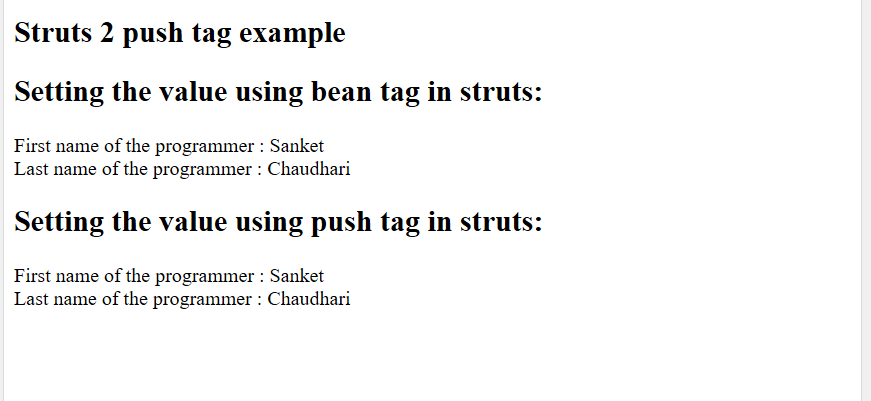