Struts 2 URL Validator
In the struts, web application, a url validator is used to check whether the entered url is valid or not. One parameter defined with url validator is:
- fieldName: It is used to specify the name of the field which is to be validated.
Struts Bundled Validators - url Example:
To get input from the user create the index.jsp file:
The index.jsp page takes the input from the user. The user will enter the url. Then after clicking on the Register button, it will redirect to the next resource. It contains one textfield and one button on the form with the link to Register class
<%@ taglib uri="/struts-tags" prefix="s" %>
<html>
<head>
<STYLE type="text/css">
.errorMessage{color:red;}
</STYLE>
</head>
<body>
<s:form action="register">
<s:textfield name="url" label="Website URL"></s:textfield>
<s:submit value="register"></s:submit>
</s:form>
</body>
</html>
Create welcome.jsp file to denote success:
The welcome.jsp page displays Welcome to the world of the Technology and entered url by the user when execute method return success string.
<%@ taglib uri="/struts-tags" prefix="s" %>
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Welcome page</title>
</head>
<body>
Welcome to the world of the Technology.
Entered URL is:<s:property value="url"/>
</body>
</html>
Create the action class Register.java:
The Action class Register.java contains only one field url with their getter and setters. It contains the execute method. On success, it will go to the welcome.jsp page.
import com.opensymphony.xwork2.ActionSupport;
public class Register extends ActionSupport
{
private String url;
public String getUrl()
{
return url;
}
public void setUrl(String url)
{
this.url = url;
}
public String execute()
{
return "success";
}
}
web.xml file is created inside WEB-INF folder in WebContent folder:
The web.xml file defines how elements are processed. The entry of FilterDispatcher is done in the web.xml file. This file is created in WebContent->WEB-INF folder. /* specifies all urls will be parsed. This task is done by struts filter.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>StrutsBundledValidation</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Construct struts.xml file:
In the struts.xml file, make the entry of the action class Register and link for it and result pages. The result determines what browser will display after the execution of the action. Results have optional names like success and input.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<package name="default" extends="struts-default">
<action name="register" class="Register">
<result name="input">index.jsp</result>
<result name="success">welcome.jsp</result>
</action>
</package>
</struts>
Create the validation file:
Create the Register-validation.xml file to validate url. It allows the valid url and displays errors for invalid url.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE validators PUBLIC
"-//OpenSymphony Group//XWork Validator 1.0.2//EN"
"http://www.opensymphony.com/xwork/xwork-validator-1.0.2.dtd">
<validators>
<field name="url">
<field-validator type="requiredstring">
<message>URL can't be blank.</message>
</field-validator>
<field-validator type="url">
<message>URL must be correct.</message>
</field-validator>
</field>
</validators>
Output:
In order to run the application, right-click on the project -> Click on the option Run As -> then select Run on Server. It will show one label to enter the website url with a text field and one register button.
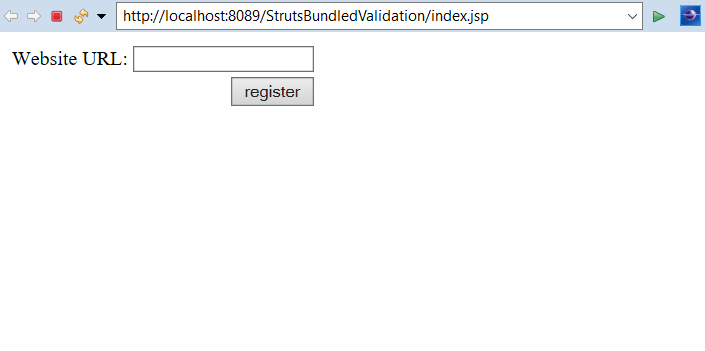
Enter the valid url like http://www.google.com and click on the register button.
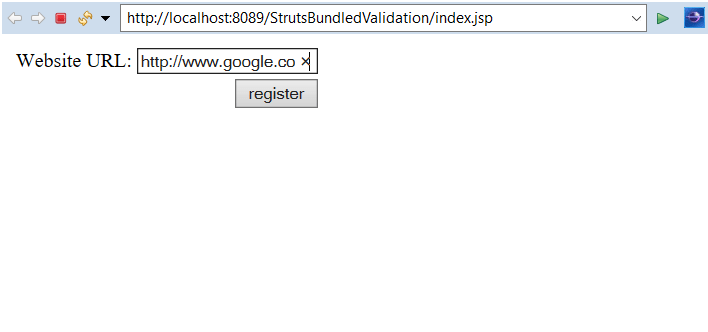
As the url entered is valid, it will redirect to welcome.jsp page displaying Welcome to the world of Technology. It will also display entered url by the user.
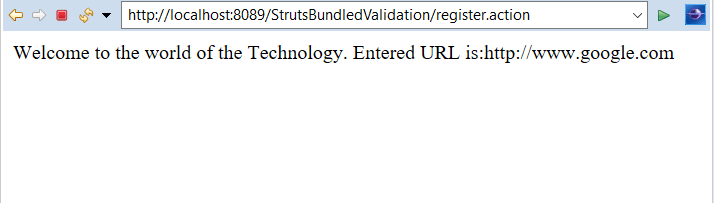