Struts 2 Installation and Example
Struts Installation:
For developing struts applications, you need to have java installed on your machine.
For verifying that Java is already installed on your machine, use the following commands:
- For Windows:
- Open Command line.
- Type the command, C:\>java -version
- Press Enter key. Current version of Java is shown.
- For Linux:
- Open Command Terminal.
- Type the command, $ java-version.
- Press Enter key. Current version of Java is shown.
If Java is not configured on your local machine follow the below steps to install java:
1. Install Java SDK from Oracle website, https://www.oracle.com/java/technologies/javase-downloads.html
2. Install JDK from Download files.
3. Follow the instruction given in Documentation to install setup.
4. Set Path for Java compiler
- For Windows,
Insert ‘;%JAVA_HOME% bin’ at the end of system variable and path
- For Linux,
Export PATH=$PATH:$JAVA_HOME/bin/
5. Set Java_Home variable to the path in your local machine where java is installed
- For Windows,
Set Java_Home variable to C:\Program Files\Java\jdk1.8.0_171
- For Linux,
Export JAVA_HOME=/usr/local/java-current
For Setting up tomcat server in your eclipse IDE:
1. Download apache tomcat version required from http://tomcat.apache.org/
2. Click on Download Tomcat 8.0. Choose 32-bit windows zip / 64-bit windows zip.
3. Choose any location to extract it.
4. Open Eclipse IDE.
5. In Eclipse IDE, click on the Servers tab.
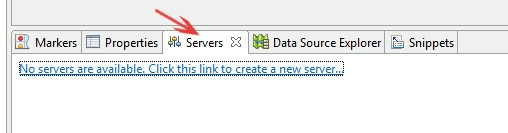
6. In the window click on the link to create a new server.
7. Select the Tomcat v8.0 Server from the available list.
8. Click on the Next button
9. Select the directory where Tomcat is installed and then click on the Finish button.
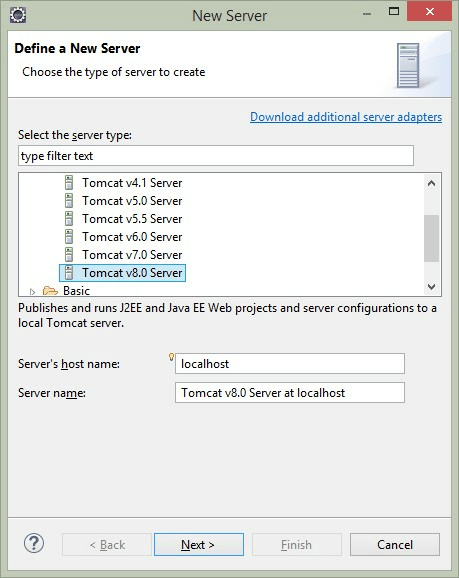
10. You will see Tomcat v8.0 in the Servers tab. Right-click on it and click on start.

For creating struts application in Eclipse IDE,
Download struts zip file from https://struts.apache.org/download.cgi/
Extract these files in any of the folders in any directory.
Struts Example:
In struts, we are developing a Hello World example. The web application takes the name of the user and displays Hello World and username. The following are the components:
- Action: The complete business logic along with the interaction between model, view, and the user is controlled by the Action class.
- Interceptors: It is a part of the controllers if it is required we can use the existing one or can create our interceptor
- View: The JSP pages used to take input from the user and display the final result are included in the View components
- Configuration files: Different files such as struts.xml and web.xml helps to combine action, controller and view.
Open the Eclipse IDE, go to the File menu click on New and then select dynamic web project enter the name as Hello World Struts2, and then click on Finish.
In the next step, click on the option generate web.xml deployment descriptor.
To create the struts the application copy the files from the lib folder of Struts2 and paste them into the WEB-INF\lib folder.
Create an action class named HelloWorld in a package com.example
package com.example;
public class HelloWorld
{
private String name;
public String get() throws Exception
{
return “Success”;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
}
Now to represent the final message JSP page is created, Helloworldname.jsp in the WebContent folder.
<%@ page contentType = "text/html; charset = UTF-8" %>
<%@ taglib prefix = "s" uri = "/struts-tags" %>
<html>
<head>
<title>Hello World</ title >
</head>
<body>
Hello World, <s:property value = "name"/>
</body>
</html>
Create a main page named index.jsp
<%@ page language = "java" contentType = "text/html; charset = ISO-8859-1"
pageEncoding = "ISO-8859-1"%>
<%@ taglib prefix = "s" uri = "/struts-tags"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h3>Hello World Struts Example</h3>
<form action = "hello">
<label for = "name">Enter the user name </label><br/>
<input type = "text" name = "name"/>
<input type = "submit" value = "Submit"/>
</form>
</body>
</html>
Create struts.xml files in classes folder in WEB-INF folder
<?xml version = "1.0" Encoding = "UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name = "struts.devMode" value = "true" />
<package name = "helloworld" extends = "struts-default">
<action name = "hello"
class = "com.example.HelloWorld"
method = "get">
<result name = "Success">/ Helloworldname.jsp</result>
</action>
</package>
</struts>
Create web.xml configuration files as follows
<?xml version = "1.0" Encoding = "UTF-8"?>
<web-app xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xmlns = "http://java.sun.com/xml/ns/javaee"
xmlns:web = "http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation = "http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id = "WebApp_ID" version = "3.0">
<display-name>Struts 2</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>struts2</filter-name>
<filter-class> org.apache.struts2.dispatcher.FilterDispatcher </filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
Output:
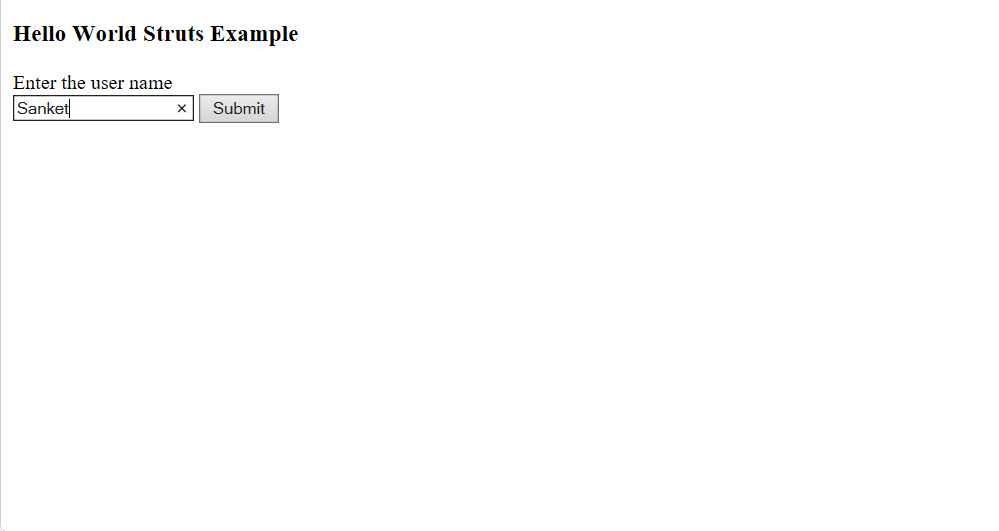
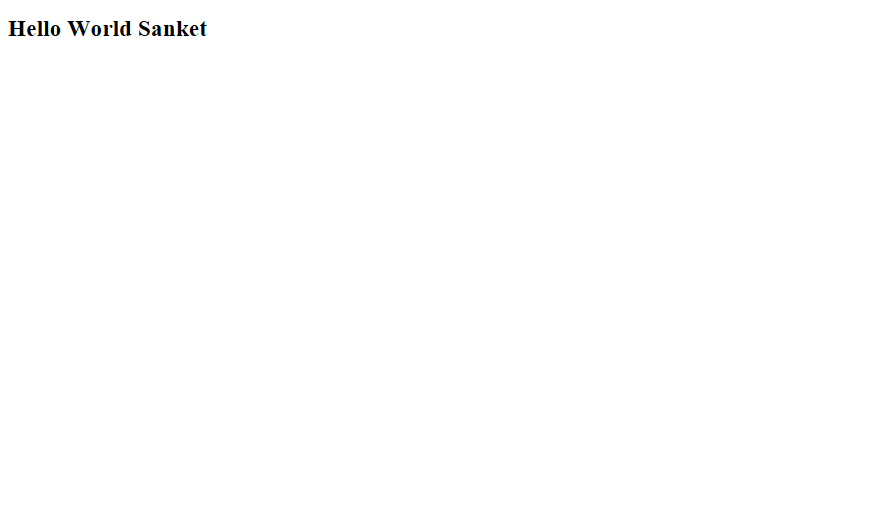