Assert in DART
In any programming language, resolving error is the most unwanted and tedious task. At times it becomes very difficult to find the error in the large code. Dart offers an efficient way of managing error in your code by using an ' Assert ' statement. It debugs the code using the Boolean condition for testing. If the Boolean expression evaluates to true, the program continues its normal execution, while if it evaluates to false, the code then ends with as assertion error.
Given below is the syntax of using the assert statement :
assert( condition ) ;
An important point to note :
It is important to remember that you need to enable the assert statement using command prompt before actually using it. This has to be done while execution as assert statement can be used only in the development mode and not in the productive mode. If you do not enable this statement, the compile will simply ignore it while execution.
Let us see how to enable the assert statement while executing a dart file using command prompt :
dart --enable-asserts file_name.dart
Consider the following example in Dart that uses the assert statement in the program
Example 1 :
void main( )
{
String str = " This is the tutorial of assert statement ! " ;
assert( str != " This is the tutorial of assert statement ! " ) ;
print( " This line can be seen as the output on the console ! " ) ;
}
Output :
Without using command prompt :
This line can be seen as the output on the console !
When Asserts are enabled :
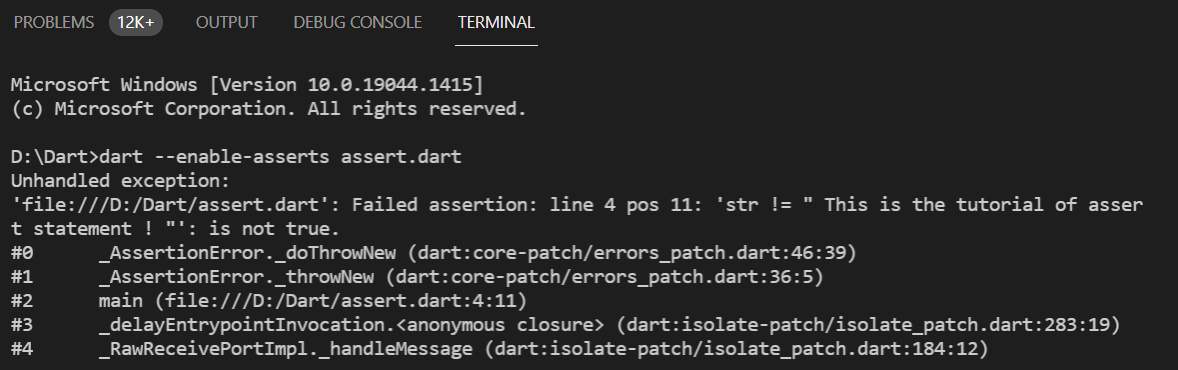
Output through command prompt :
Unhandled exception:
'file:///D:/Dart/assert.dart': Failed assertion: line 4 pos 11: 'str != " This is the tutorial of assert statement ! "': is not true.
#0 _AssertionError._doThrowNew ( dart : core-patch/errors_patch.dart : 46 : 39 )
#1 _AssertionError._throwNew ( dart : core-patch/errors_patch.dart : 36 : 5 )
#2 main ( file:///D :/Dart/assert.dart : 4 : 11 )
#3 _delayEntrypointInvocation.<anonymous closure> ( dart : isolate-patch/isolate_patch.dart : 283 : 19 )
#4 _RawReceivePortImpl._handleMessage ( dart : isolate-patch/isolate_patch.dart : 184 : 12 )
Assert with message
Apart from just catching an error using the assert statement, we can also display a productive message against that assert statement if it evaluates to false.
Syntax of using this is as follows :
assert( condition, ' message ' ) ;
This turns out to be of great advantage when we are handling many errors using the assert statement. This message functionality lets us know which assert statement has actually returned the error in the code.
Consider another example demonstrating the above concept :
Example 2 :
void main( )
{
String s1 = " This is an assert statement ! " ;
assert( s1 == " This is an assert statement ! ", " Cool ! The strings are equal. " ) ;
print( " You Can See This Line on console as an output if the assert returns true. " ) ;
}
Output :
Without using the command prompt :
You Can See This Line on console as an output if the assert returns true.
When Asserts are enabled :
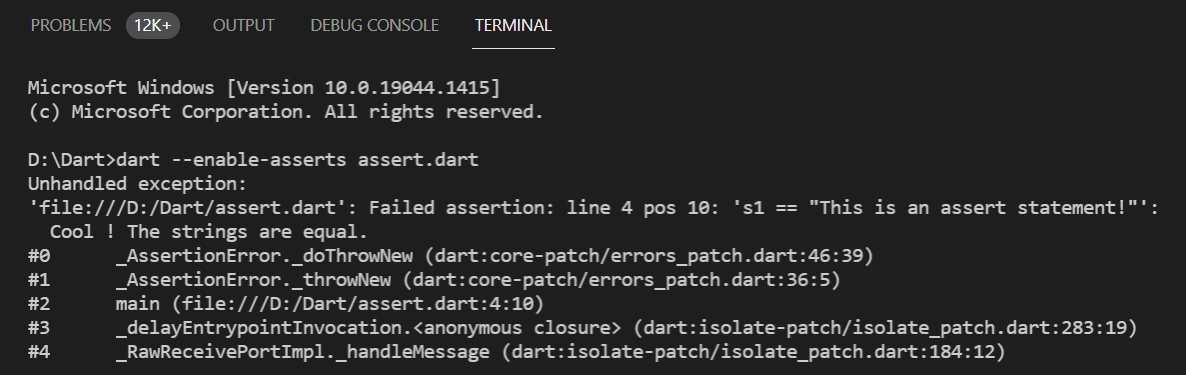
Output through command prompt :
Unhandled exception:
'file:///D:/Dart/assert.dart': Failed assertion: line 4 pos 10: 's1 == "This is an assert statement!"': Cool ! The strings are equal.
#0 _AssertionError._doThrowNew ( dart:core-patch/errors_patch.dart : 46 : 39 )
#1 _AssertionError._throwNew ( dart : core-patch/errors_patch.dart : 36 : 5 )
#2 main ( file:///D:/Dart/assert.dart : 4 : 10 )
#3 _delayEntrypointInvocation.<anonymous closure> ( dart : isolatepatch/ isolate_patch.dart : 283 : 19 )
#4 _RawReceivePortImpl._handleMessage ( dart : isolate-patch/ isolate_patch.dart : 184 : 12 )