Dart Basic Program
Dart provides various convenient platforms to code and compile the program in Dart language. Some of them are:
1. Using IDE = Visual Studio code is a text editor with IDE. All you have to do is download the Dart extension, write your code with the .Dart extension and compile using the same.
2. Online Compiler = DartPad is an online compiler provided by the Dart language to code and compile the program in Dart.
3. Using Command line = After downloading Dart SDK, one can easily code in Dart using Dart specific commands in the command line client.
In case you haven’t downloaded it, visit the previous tutorial that discusses the installation of the above platforms in detail.
Let’s write our first program in Dart.
Program:
//This is the first Dart program
void main( )
{
var a = 5, b = 6 ;
var c = a + b ;
print( ' Sum of two numbers is $c. ' ) ;
}
- Using Visual Studio Code
- Open Visual Studio Code.
- Type your code.
- Save the file with the .Dart extension.
- Run the code using F5 or the run button on the top right corner.
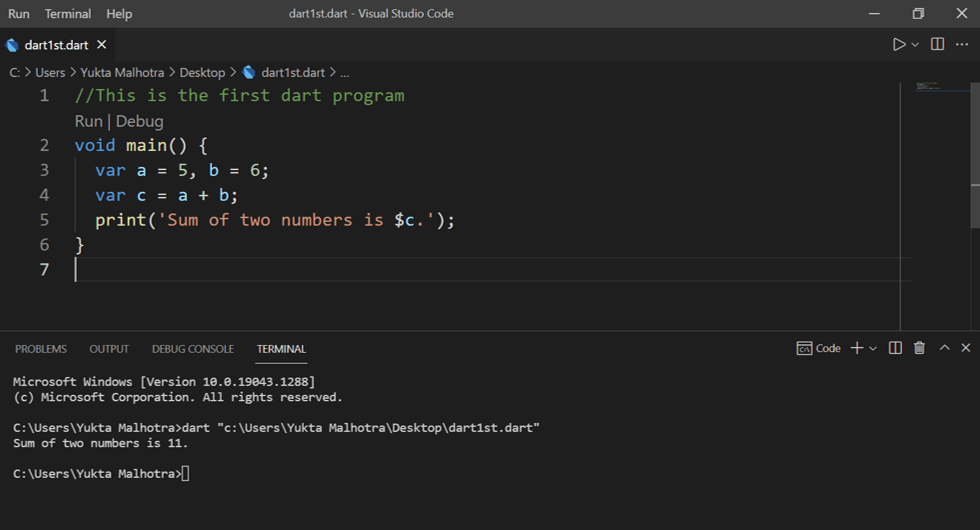
- Using online compiler DartPad.
- Search on Google DartPad.
- Type your code and run it.
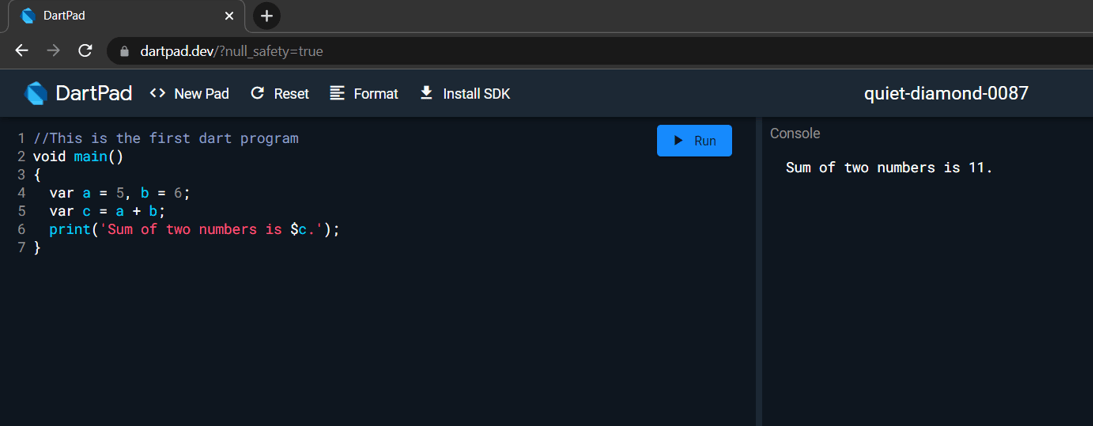
- Using the command line.
- Open notepad and type your code.
- Save the file with the file name as ‘name.Dart’. .Dart to recognize that this is a Dart file.
- Open the command prompt and write the command ‘Dart filename’. Here, the ‘filename’ is to be replaced with the name of your file.
- Press enter, and output will be displayed.
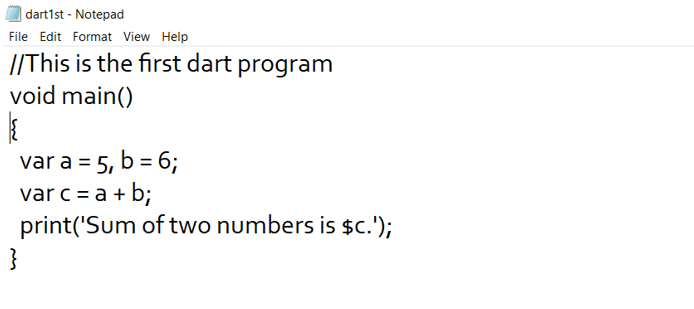
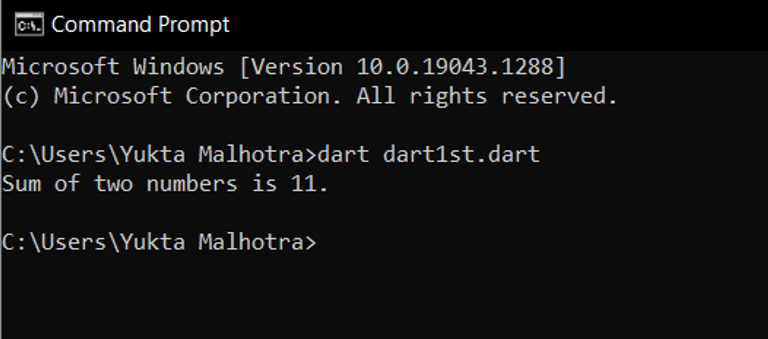
Let’s understand the program in detail.
This program initializes two numbers, adds them and print their sum.
//This is the first Dart program
void main( )
{
var a = 5, b = 6;
var c = a + b;
print( 'Sum of two numbers is $c.' );
}
- This is the first Dart program
The first line beginning with ‘//’ is a single-line comment. Comments do not get executed but are written in order to make the code more descriptive and easier to understand.
Dart also supports multi-line comments in case of multiple lines as follows:
/*…………………
……………………*/
- main( )
Like in C, the execution of the program always and only begins from the main( ) function.
- void
Return type of the function main( ) is void. Return type informs the compiler about the type of value the function will return. void means that the function does not return any value.
It can have other return types as well like, int, double, etc.
- The whole program is contained within the curly braces { }. Remember that group of programming statements will always be enclosed within the curly braces { }.
- var a = 5, b = 6;
This is the single line, multiple variable initialization statement. ‘var’ is a keyword used to declare a variable without specifying any data type. The Dart compiler automatically interprets the data type of the variable by looking at the value initialized to it. For example, here, the values are integer type.
We can specify the data type explicitly also instead of var keyword. Initialization will look like this in that case,
int a = 5, b = 6;
- var c = a + b;
This is an assignment statement that performs the arithmetic operation, addition on variables ‘a’ and ‘b’ using plus (addition) operator ‘+’, and assigns the value of sum on the right-hand side to the variable ‘c’ on the left-hand side using assignment operator ‘=’.
An important point to remember is that in the case of an assignment statement, the value on the right-hand side is always assigned to the variable on the left-hand side. The converse is not true.
- print(‘Sum of two numbers is $c’);
print is an inbuilt function to display output on the output screen. The text to be printed on the output screen is enclosed within single quotes ‘ ’. ‘$’ operator is used to convert an expression to a string using string interpolation.
- Remember that every statement is terminated using a terminator ‘;’.