Dart Constants
Dart Constants are the objects or variables whose values can’t change or modify during the execution of the program. Their use case is when we want a particular value to remain unchanged throughout the program’s execution.
Defining Constants in Dart
In Dart, variables can be assigned with constant values using the following two methods :
- Using the ‘ final ’ keyword = final variables can be set only once as they are assigned the value at the compile-time debarring any changes in the future. ‘ final ’ keyword is used with the variable name to assign constant value.
Implementation = final var_name = value ;
Or
final data_type var_name = value ;
The second one is used when data type has to be explicitly mentioned, along with the variable name.
- Using the ‘ const ’ keyword = const is a compile-time constant and is implicitly equivalent to final. ‘ const ’ keyword is used with the variable name to assign constant value.
Implementation = const var_name = value;
Or
const data_type var_name = value;
The second one is used when data type has to be explicitly mentioned, along with the variable name.
In any case, if we try to change the value of constants, the Dart compiler throws an exception.
Program
void main( )
{
// normal variable declaration
var a = 5 ;
// printing the value of a
print( ' \n Value of a is : $a ' ) ;
// changing the value of a
a = 6 ;
// printing the value of a
print( ' \n Value of a is : $a ' ) ;
// variable declaration using final keyword
final b = 1 ;
// printing the value of final variable b
print( ' \n Value of b is : $b ' ) ;
// variable declaration using const keyword
const c = 2 ;
// printing the value of constant variable c
print( ' \n Value of c is : $c ' ) ;
}
Output :
Value of a is : 5
Value of a is : 6
Value of b is : 1
Value of c is : 2
Program
void main( )
{
// normal variable declaration
var a = 5 ;
// printing the value of a
print( ' \n Value of a is : $a ' ) ;
// changing the value of a
a = 6 ;
// printing the value of a
print( ' \n Value of a is : $a ' ) ;
// variable declaration using final keyword
final b = 1 ;
b = 7 ;
// variable declaration using cosnt keyword
const c = 2 ;
c = 8 ;
}
Output :
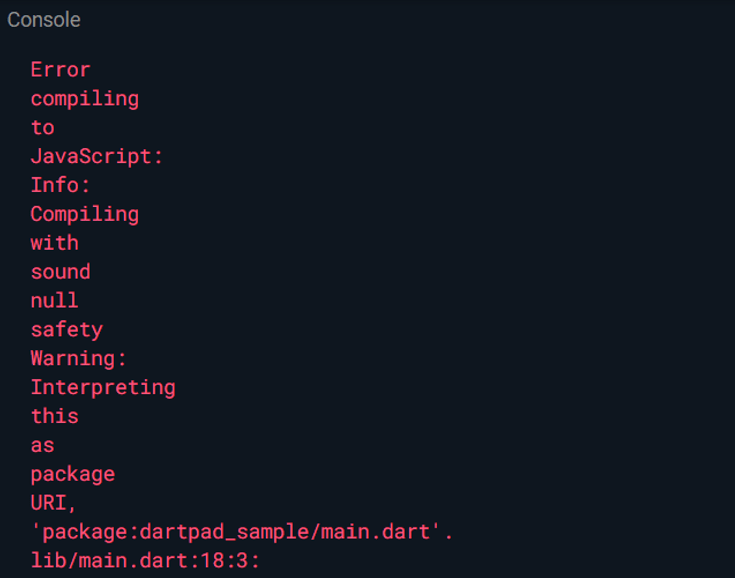
Clearly, the compiler throws an exception since we tried to change the value of b and c which are constants.