Dart HTML DOM
All web pages can be viewed as objects and exist within the browser window. We can access a web page using a web browser and it needs to be connected to the internet. DOM is an acronym for Document Object Model. Document object refers to an HTML document displayed in that window. The document object model contains a number of structures that refer to other objects that provide the modification of document content.
The process for accessing the contents of a document is called the Document Object Model, or DOM. Items are sorted by position. The hierarchical structure is used for organizing objects in a web document.
- Window – The first object in the hierarchy is the Window. It the outmost element of the object hierarchy.
- Document - When an HTML document loads in a window it becomes a window object. The document covers the content of the page.
- Elements - Mention content on a web page. Example - Title, text box etc.
- Nodes - These are usually elements, but are also attributes, comments, text and other forms of DOM.
Below is the hierarchy of the few important DOM objects.
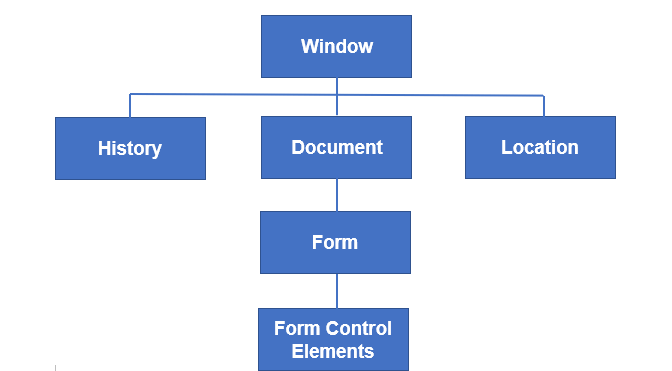
Dart HTML DOM
We can manipulate objects and elements into DOM by using the ‘ dart : html ’ library. The console-based application cannot use the ' dart : html ' library. For work with the HTML library in the web application, we need to import ‘dart : html ’.
import ' dart : html ' ;
Let's understand the DOM operation in the following section.
Finding DOM Elements
A document may contain multiple attributes and sometimes need to search for a specific attribute. The ‘ dart : html ’ library provides the query Selector function to search element in the DOM.
Element querySelector( String selector ) ;
The ‘ querySelector( ) ’ function returns the first element that matches the specified group of the selector. Let's understand the following syntax.
var element1 = document.querySelector( ' .className ' ) ;
var element2 = document.querySelector( ' #id ' ) ;
Let's understand the following example.
Example -
We create a HTML file name index.html and also create a dart file.
< !DOCTYPE html >
< html >
< head >
< meta charset = " utf-8 " >
< meta http-equiv = " X-UA-Compatible " content = " IE = edge " >
< meta name = " viewport " content = " width = device-width, initial-scale = 1.0 " >
< meta name = " scaffolded-by " content = " https://github.com/google/stagehand " >
< title > DemoWebApp < /title >
< link rel = " stylesheet " href = " styles.css " >
< script defer src = " main.dart " type = " application/dart " > < /script >
< script defer src = " packages/browser/dart.js " > < /script >
< /head >
< body >
< h1 >
< div id = " output " > < /div >
< /h1 >
< /body >
< /html >
Main.dart
import ' dart:html ' ;
void main( ) {
querySelector( ' #output ' ).text = ' Your Dart web dom app is running ! ! ! . ' ;
}
Event Handling
The ‘ Dart:html ’ library provides an ‘ onClick ’ event for DOM Elements. The syntax shows how an element can handle a series of click-through events.
querySelector( ' #Id ' ).onClick.listen( eventHanlderFunction ) ;
The ‘ querySelector( ) ’ function returns the feature to the given DOM and ‘ onClick.listen( ) ’ will take the ‘ eventHandler ’ method to be used when the click event is highlighted. The syntax for ' eventHandler ' is provided below :
void eventHanlderFunction( MouseEvent event ) { }
Let us now take an example to understand the concept of Event Handling in Dart :
TestEvent.html
< !DOCTYPE html >
< html >
< head >
< meta charset = " utf-8 " >
< meta http-equiv = " X-UA-Compatible " content = " IE = edge " >
< meta name = " viewport " content = " width = device-width, initial-scale = 1.0 " >
<meta name = " scaffolded-by " content = " https://github.com/google/stagehand " >
< title > DemoWebApp < /title >
< link rel = " stylesheet " href = " styles.css " >
< script defer src = " TestEvent.dart " type = " application/dart " > < /script >
< script defer src = " packages/browser/dart.js " > < /script >
< /head >
< body >
< div id = " output " > < /div >
< h1 >
< div >
Enter you name : < input type = " text " id = " txtName " >
< input type = " button " id = " btnWish " value = " Wish " >
< /div >
< /h1 >
< h2 id = " display " > < /h2 >
TestEvent.dart
import ' dart:html ' ;
void main( ) {
querySelector( ' #btnWish ' ).onClick.listen( wishHandler ) ;
}
void wishHandler( MouseEvent event ) {
String name = ( querySelector( ' #txtName ' ) as InputElement ).value ;
querySelector( ' #display ' ).text = ' Hello Mr. ' + name ;
}